Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial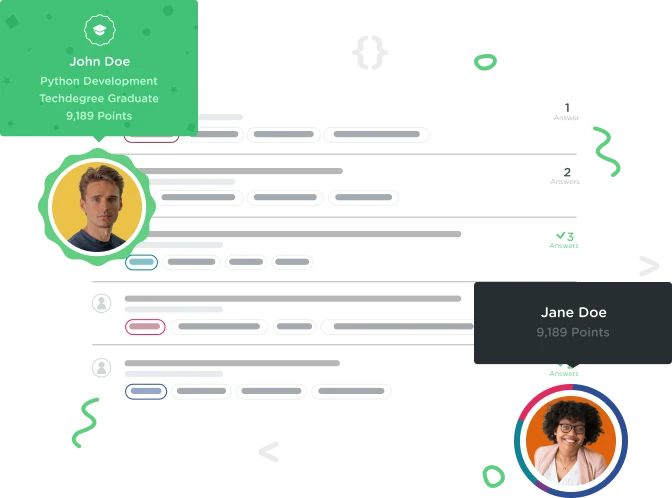

Richard Price
2,050 Pointsdon't get a value returning ENUM just get (function)
when returning from the function I don't get a value I just get (function) in brackets
anyone know whats going on?
this is my code
enum Weapons : Int {
case Sword = 500, shield = 222, Axe = 3434, startingEnergy = 5000
func hitMe(weapon : Weapons) -> Int {
return Weapons.startingEnergy.rawValue - weapon.rawValue
}
}
var s = Weapons.Sword
Weapons.hitMe(s)
1 Answer

Melissa Garza
12,671 PointsAll you really have to do to fix this particular error so it returns the correct value would be to change your last line of code:
Weapons.hitMe(s) // Returns the hitMe() method itself (that's why it shows "function")
s.hitMe(s) // This would return the value you want
The line of code returning the function isn't accessing an instance of a weapon you created (which is in the s variable), but rather the enum itself (which is Weapons). Hence why there's no runtime error. It's returning what you asked, it's just the wrong thing.
Also note that having startingEnergy as an enum member doesn't quite fit. It'd be weird to create a weapon of type startingEnergy. It would make more sense to place that as a parameter to one of the enum's methods in this case.
As stated in the video at around 3:22, he says the line of code like shown below is redundant. That's where the keyword self comes into play. Instead of having to pass in the variable to a method that already has access to it through an instance (the variable s), simply use the keyword self to access the data.
today.daysTillWeekend(today) // Like in the video
s.hitMe(s) // You have something similar going on
So instead of passing in the weapon as a parameter to the method, just replace weapon.rawValue with self.rawValue.
Here's a revised version of your code with Swift coding conventions in mind:
enum Weapon : Int {
case Sword = 500,
Shield = 222,
Axe = 3434
func calculateRemainingEnergy(#currentEnergy: Int) -> Int {
return currentEnergy - self.rawValue
}
}
var coolSword = Weapon.Sword
coolSword.calculateRemainingEnergy(currentEnergy: 900) // Result: 400
var neatAxe = Weapon.Axe
neatAxe.calculateRemainingEnergy(currentEnergy: 3500) // Result: 66
Hope this helps you or anyone else stumbling upon it :)
Mely