Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial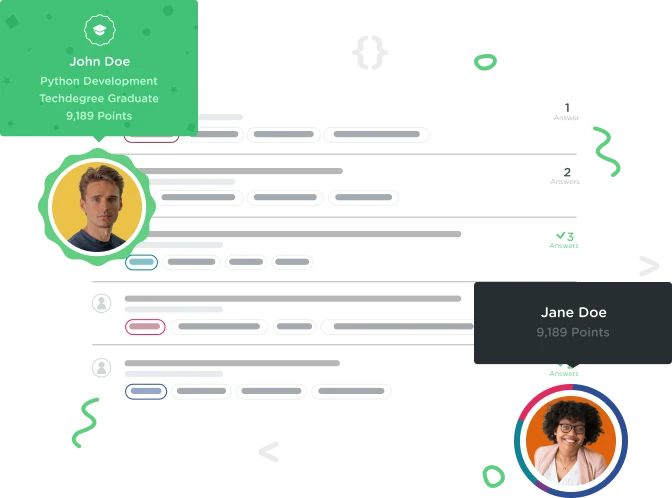

Jonas Kristensen
2,738 PointsDon't get the challenge?
Create an instance of the class RoundButton and name the instance rounded. How do i do?
class Button {
var width: Double
var height: Double
init(width:Double, height:Double){
self.width = width
self.height = height
}
class RoundButton: Button{
var cornerRadius = 5.00
}
}
var rounded = RoundButton(cornerRadius: 5.00)
3 Answers
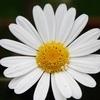
Mindy Sandilands
15,743 Pointsclass Button {
var width: Double
var height: Double
init(width:Double, height:Double){
self.width = width
self.height = height
}
}
class RoundButton: Button{
var cornerRadius = 5.0
}
var rounded = RoundButton(width: 5.0, height: 5.0)
This is the code I used to complete the challenge. It's probably just a simple syntax error. Check your curly braces. I believe you need to move one of the curly braces at the end of your code to before the class RoundButton.
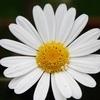
Mindy Sandilands
15,743 PointsFor this challenge RoundButton is a subclass of the superclass Button so it inherits the properties(width and height) from Button. You need to provide these properties values when you create an instance of RoundButton. So your instance of RoundButton should look like this:
var rounded = RoundButton(width:10, height:10).
You can chose whatever value for width and height you want I just put in 10.
Hope this helps, Mindy

Jonas Kristensen
2,738 Pointsclass Button {
var width: Double
var height: Double
init(width:Double, height:Double){
self.width = width
self.height = height
}
class RoundButton: Button {
var cornerRadius = 5.0
var rounded = RoundButton(width: 5.0, height: 5.0)
}
}

Jonas Kristensen
2,738 PointsIt still won't work?
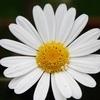
Mindy Sandilands
15,743 PointsYou need to call the instance outside of the class RoundButton. Move the code outside of the subclass RoundButton by placing it after the curly braces.
class RoundButton: Button{ var cornerRadius = 5.0}
var rounded = RoundButton(width: 5.0, height: 5.0)
Mindy

Jonas Kristensen
2,738 PointsIt doesn't work. It says Swift swift_lint.swift:16:16: error: use of unresolved identifier 'RoundButton'
var rounded = RoundButton(width: 5.0, height: 5.0)
Jonas Kristensen
2,738 PointsJonas Kristensen
2,738 PointsWooops stupid mistake. I made my Roundbutton into the Button class. But i wonder it could be accepted anyways? Thanks a lot!!!!