Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial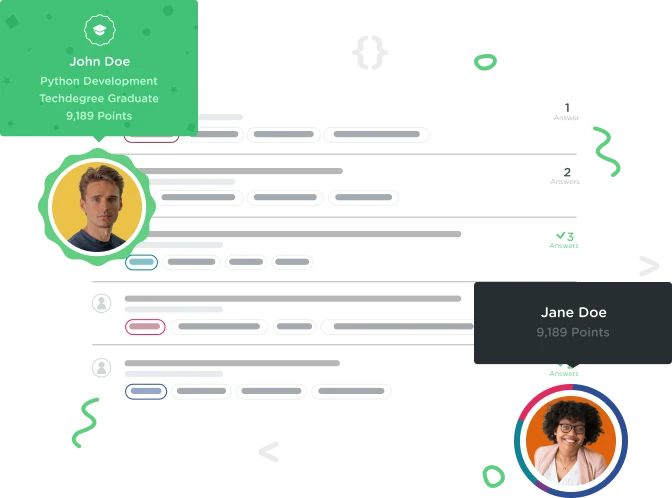
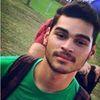
Gustavo Winter
Courses Plus Student 27,382 PointsDon't get the syntax.
Hi, i don't understand why and how he can use the name of the class instead of String or Object to declare the type.
public KaraokeMachine(SongBook songBook){ // Why he use SongBook to declare a variable songBook
mSongBook = songBook;
new InputStreamReader(System.in)
}
If you can explain the purpose and how it's available, i'll appreciate.
package com.teamtreehouse.model;
import java.util.ArrayList;
import java.util.List;
public class SongBook{
private List<Song> mSongs;
public SongBook(){
mSongs = new ArrayList<Song>();
}
public void addSong(Song song){
mSongs.add(song);
}
public int getSongCount(){
return mSongs.size();
}
}
3 Answers

Steven Kelsey
12,524 PointsNo, SongBook (singular and Capital S) is the class, a class is the blueprint of how to make an object. When we use the new keyword followed by any class name we get a single instance/object (object and instance are interchangeable). Like:
Object object = new Object();
The capital Object is the class (the blueprint for how to make a single object) and the lower case object is the variable that hold the value - new Object()
//Class Datatype //assignment operator =
Object object = new Object();
//variable name //new keyword used to create a object of type Object
The same would be for any other object: Object object = new Object();
SongBook songBook = new SongBook();
SongBook songBook1 = new SongBook();
SongBook songBook2 = new SongBook();
Above I made 3 instances of SongBook, each would have difference variable values

Steven Kelsey
12,524 Pointspublic KaraokeMachine(SongBook songBook)
The SongBook with the capital S is the datatype, while the songBook is the variable.
Similar would be: public KaraokeMachine(String songBook) but now the datatype of songBook is String not a SongBook object meaning developers could only pass in String Object arguments.
Sidenote: Java is a strongly type aka strictly typed language, meaning once you declare the datatype of a variable e.g.
String phoneNumber;
phoneNumber = "123";
You can only assign Strings to that variable. I can't do:
phoneNumber = 123;
This would give me a compilation error as we previously told the compiler that the datatype is String.
Verse other languages like JavaScript that is LooselyTyped meaning you can change the datatype of a variable anytime like:
var age = 10; //datatype = Number
age = "10; //datatype now = String
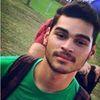
Gustavo Winter
Courses Plus Student 27,382 PointsSo, the new songBooks is a subclass of SongBook ?
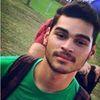
Gustavo Winter
Courses Plus Student 27,382 PointsI guess i understand. Thank you.
Steven Kelsey
12,524 PointsSteven Kelsey
12,524 PointsThe 3 instance currently share the same values but if you changed the value of one variable it wouldn't the value of the other like:
To make this easier to understand I have to modify the SongBook Class a little:
I gave the SongBook class another instance variable called songBookName, and its datatype is String This will allow me to:
3 different objects of the Class SongBook, all have different variable values, changing one WON'T change the others