Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial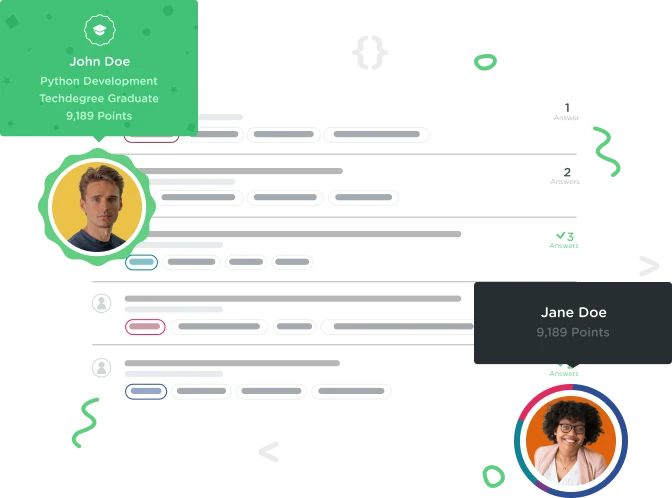

Marguerite Holden
6,075 PointsDon't get this
Using a loop construct, assign the value of the get_answer() method to an answer variable. Use the break keyword to exit the loop if the answer variable is equal to the string e. Assume get_answer() is already written.
# Assume get_answer() is already defined
loop do
print "Enter an answer ("e" to exit): "
answer = gets.chomp
break if answer == "e"
end
2 Answers
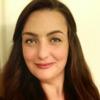
Jennifer Nordell
Treehouse TeacherHi Marguerite! There are a few problems here, and some of them have to do with following the instructions of the challenge. It's always a good idea to not do anything the challenge doesn't specifically ask for. In this case, you've printed a line and then used gets.chomp
to get the answer. However, neither of these are required nor expected by the challenge.
Take a look:
loop do
answer = get_answer()
if answer == "e"
break
end
end
Here we start our loop. As indicated by the instructions and the comment already available in the code, the value of answer
will be provided by calling the function get_answer
. Now, if answer is equal to "e", the loop will break. Then we end our if statement. Then we end our loop.
Hope this helps!
edited for additional note
Alexander Davison is correct. The if statement in combination with the break can be shortened to break if answer == "e"
. I have edited my answer to reflect this.
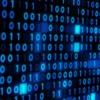
Alexander Davison
65,469 PointsAlso, a little note to Jennifer, just FYI Ruby has a shortcut.
Ruby lets you shorten this:
if answer == "e"
break
end
To this:
break if answer == "e"
Try it in the IRB and they should work the same :)
Just a little note
~alex
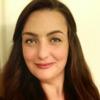
Jennifer Nordell
Treehouse TeacherThanks, I actually didn't know you could shorten that line that way (or didn't remember). Excellent point!
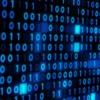
Alexander Davison
65,469 PointsCool! Also, like many other languages, Ruby also has a Ternary Operator that I use even more often.
You might know how it works because it is supported in many languages including JavaScript.
Here's the ternary operator in action:
# This program has no purpose it is just a demo. LOL
random_number = 5
# Note: no need for the parentheses it just looks a little nicer to me :)
food = (random_number == 5 ? "apple" : "orange")
If you wanted to write that same thing the regular way, you would have to do this:
random_number = 5
if random_number == 5
food = "apple"
else
food = "orange"
end
Here's how the ternary operator works:
- First you specify the condition.
- Next, you put a question mark. This is like asking a question.
- Then, you put your first value (Ruby will pick this value if the condition is true)
- Next, put a colon. This is like saying
else
. - Lastly, put another value. If the condition is false, Ruby will pick this value.
I hope this helps! ~alex

Marguerite Holden
6,075 PointsThanks for your input and explaining the ternary operator.
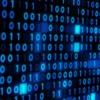
Alexander Davison
65,469 PointsNo problem :)