Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial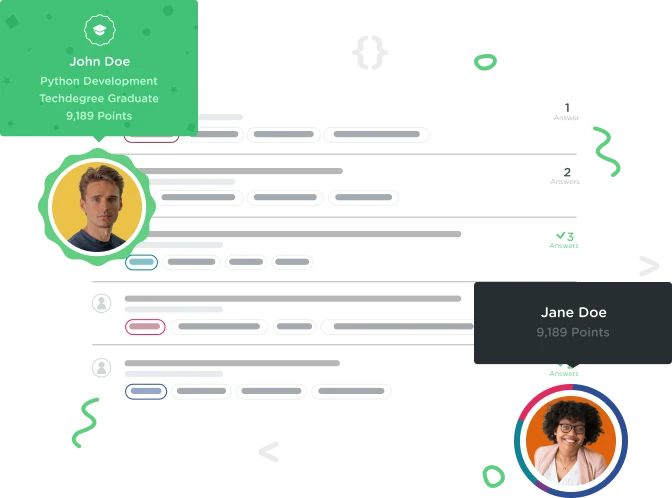

fahad lashari
7,693 PointsDon't know of any alternative to zip(). Any help?
The last time I completed this track. I used the zip() function to iterate through two loops simultaneously. I am however not aware of how to achieve this in an alternative matter. Any help would be much appreciated.
Kind regards,
Fahad
# combo([1, 2, 3], 'abc')
# Output:
# [(1, 'a'), (2, 'b'), (3, 'c')]
def combo(list1, list2):
list = []
for i, j in zip(list1, list2):
list.append((i, j))
return list
1 Answer
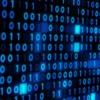
Alexander Davison
65,469 PointsKenneth put a little change on this code challenge that makes you so you can't use zip
.
If you can't think of another way, you can only loop through the indexes of the first/second list. Then you use those indices to get the element at the same indices on both lists.
def combo(iter1, iter2):
result = []
for index in range(len(iter1)):
result.append((iter1[index], iter2[index]))
return result
What I'm doing:
- I set up the function definition and the
result
variable to be an empty list. - I go though every index of the first iterable. (It doesn't matter if it's the first or the second, because the challenge said they will be the same length)
- I add a pair (a tuple with two items is sometimes called a pair) with the first element to be the first iterable's
index
index and the second element to be the second iterable'sindex
index. - I finally return the
result
list. (duh)
You sometimes have to think what you would do if you had to solve it without a computer then do that on the computer.
I hope this helps. ~Alex
Alexey Serpuhovitov
6,931 PointsAlexey Serpuhovitov
6,931 PointsAlex, could you explain, why we need
for index in range(len(iter1)):
pattern and not just
for index in iter1:
Alexander Davison
65,469 PointsAlexander Davison
65,469 Pointsfor index in iter1
Will loop through every item in
iter1
, andfor index in range(len(iter1))
Will go through every index.
If we enter this into both loops...
print(index)
The first loop will print this (if
iter1
was the list ['a', 'b', 'c']):The second loop will print:
Joshua Dam
7,148 PointsJoshua Dam
7,148 PointsYeah, that works! You could also use the enumerate() function! I only bring that up because Kenneth covers enumerate() within the tuples section of the course just before this challenge.