Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial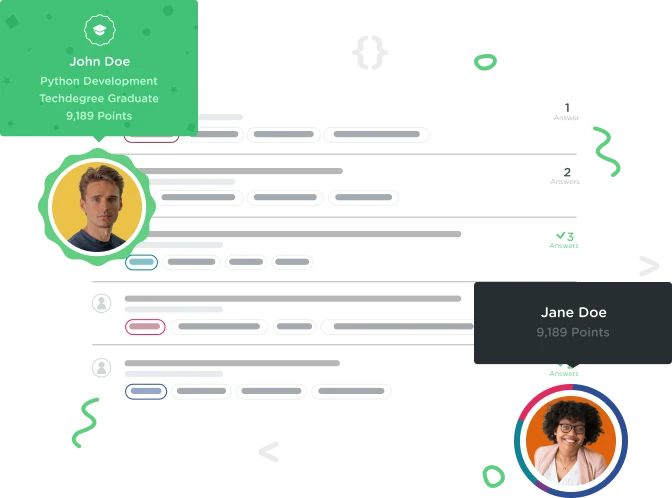

Uriah Nevins
1,121 PointsDon't know what I am doing
I have followed along the java objects course and have been doing pretty well up until the last few videos. I feel like Craig Dennis is not explaining things thoroughly. He just sort of mentions some new concept, then goes on to something else without explaining. I have attached my code with all the things I don't understand pointed out:
//Q #1. First off, why multiple files???
//Hangman.java file
public class Hangman {
public static void main(String[] args) {
Game game = new Game("treehouse"); //Q #2. What is line this doing?
Prompter prompter = new Prompter(game); //Q #3. And this one?
boolean isHit = prompter.promptForGuess(); //Q #4. I think that the 'example.exampleTwo();' concept is very confusing. What is it doing?
if (isHit) {
System.out.println("We got a hit!");
}else{
System.out.println("Oops, we got a miss.");
}
}
}
//Prompter.java file
import java.io.Console;
public class Prompter{
private Game mGame; //Q #5. What are member variables for?
public Prompter(Game game){
mGame = game;//Q #5. cont'd. And why are they just made equal again?
}
public boolean promptForGuess() {
Console console = System.console(); //Q #6. What is this line doing?
String guessAsString = console.readLine("Enter a letter: ");
char guess = guessAsString.charAt(0);
return mGame.applyGuess(guess);
}
}
//Game.java File (I think I understand this one)
public class Game{
private String mAnswer;
private String mHits;
private String mMisses;
public Game(String answer) {
mAnswer = answer;
mHits = "";
mMisses = "";
}
public boolean applyGuess(char letter){
boolean isHit = mAnswer.indexOf(letter) >= 0;
if(isHit){
mHits += letter;
}else{
mMisses += letter;
}
return isHit;
}
}
I'd be glad for any help. Thanks!
-Uriah
1 Answer

Evan Maroge
5,277 Points2.
when you type "Game game", you declare an object of type "Game" (a class you made) and with a reference "game."
when you type "new game("treehouse");" you are instantiating that object. The class Game is a blueprint on how to make a game. when you type new Game(""), you are making an object from the blueprint you created (the class Game).
what goes in the parenthesis is the parameters. That depends on what kind of constructor you created. in your case, the class Game has a constructor
public Game(String answer) { mAnswer = answer; mHits = ""; mMisses = ""; } that accepts string. which is what you are passing with "new Game("treehouse");
- same thing as above. you are declare and instantiating.
4. boolean isHit = prompter.promptForGuess(); for this, you are declaring a boolean type with the word "boolean" and reference to it is the "isHit" the "prompter." is the reference to the object you created of the class Prompter. ( Prompter prompter = new Prompter(game);). the "." is a special key in java, called dot operator. after you type the reference to the object, you then type the dot operator. here, you typer prompter then the .
the dot gives you access to the methods in that class which you can call.
5. private Game mGame; this is a game object that is private. encapsulation is the topic here. more to read on this for sure but the basic idea is to keep certain objects in the class private so other classes cant modify it.
the other part of 5 is just getting the value of the mGame reference of Game object to what you pass in.
- I have no used the Console class before.