Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial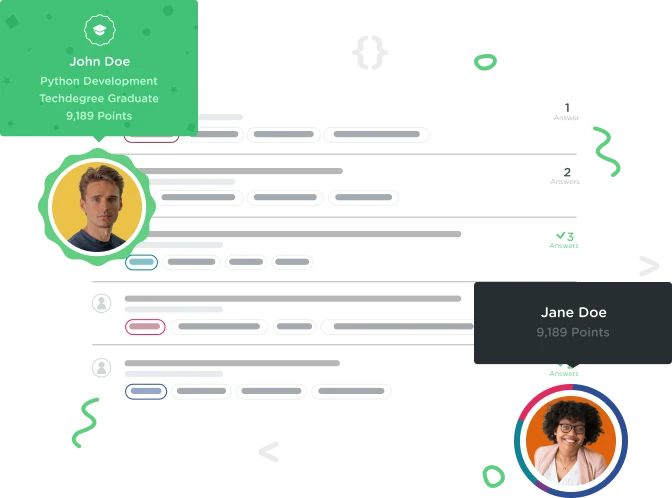
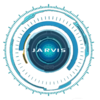
jenyufu
3,311 PointsDon't know what I'm doing wrong but nothing shows up in the Inbox list
All I see in my messages is "No new messages" Here is my code.
package com.joinedit.ribbitpractice;
import android.content.Context;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.ArrayAdapter;
import android.widget.ImageView;
import android.widget.TextView;
import com.parse.ParseObject;
import java.util.List;
/**
* Created by yofu1 on 9/25/2015.
*/
public class MessageAdapter extends ArrayAdapter<ParseObject> {
protected Context mContext;
protected List<ParseObject> mMessages;
//7.4 create custom layout for messages
public MessageAdapter(Context context, List<ParseObject> messages) {
super(context, R.layout.message_item);
mContext = context;
mMessages = messages;
}
//7.4 Override getView() in order to use a custom list adapter.
// for adapters for fragments, the adapter called a certain method to get an appropriate fragment.
// And then it went ahead and adapted it and put it in the view.
//This adapter, which is going to be attached to a list view,
// is going to call a method, called getView,
// and it's going to create the view, inflate it into a layout and then attach it into the list view.
@Override
public View getView(int position, View convertView, ViewGroup parent) {
//7.4 we want to do getView as efficiently as possible because the more efficient we are in this method, the faster it will perform
ViewHolder holder;
//RecyclerView (recycle views if it already exists.)
if (convertView == null) { //if the view holder does not exist: create new holders
convertView = LayoutInflater.from(mContext).inflate(R.layout.message_item, null);
holder = new ViewHolder();
holder.iconImageView = (ImageView) convertView.findViewById(R.id.messageIcon); //if you do (ImageView)findViewById(R.id.messageIcon) it will be RED error. need "convertView." in front
//^ find view by ID is an activity method.
//But we can call it from the convert view because in this instance, we want to get it for the layout for this view.
//Because we're not attached to the activity yet.
//do the same thing for TextView holder:
holder.nameLabel = (TextView) convertView.findViewById(R.id.senderLabel);
convertView.setTag(holder);
} else { // else if it already exits: reuse the old holders
holder = (ViewHolder)convertView.getTag(); //.getTag() method gets the holder that was already created
}
//7.4 Now that we have the view, get the ParseObject that correspond to each item on the list:
ParseObject message = mMessages.get(position); //get the positon of the message
//7.4 change icon of the message in layout based on message type
if (message.getString(ParseConstants.KEY_FILE_TYPE).equals(ParseConstants.TYPE_IMAGE)){ //if image file type ".equals" image then:
holder.iconImageView.setImageResource(R.drawable.ic_action_picture); //set message Icon
} else { //else set it to image icon for video:
holder.iconImageView.setImageResource(R.drawable.ic_action_play_over_video);
}
holder.nameLabel.setText(message.getString(ParseConstants.KEY_SENDER_NAME)); //set message sender name
return convertView;
}
//7.4 Create a ViewHolder class to use viewHolder (android convention)
private static class ViewHolder {
//7.4 a very simple class that just includes the data that is going to be displayed in the custom layout.
ImageView iconImageView;
TextView nameLabel;
}
}
2 Answers
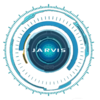
jenyufu
3,311 PointsNvrm, solved it:
super(context, R.layout.message_item, messages); //<-I forgot the ",messages" part

Peter Kazimir
5,277 Pointsthanks! you saved me a lot of time by posting this!