Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial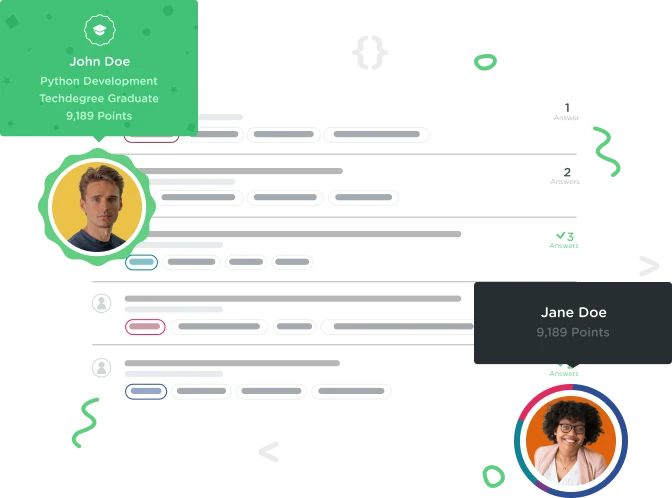

Chidiebere Ekennia
5,950 PointsDon't know what to do about this challenge :(
i don't know how to add logic to make it work well
class GoKart {
public static final int MAX_BARS = 8;
private String color;
private int barCount;
private int lapsDriven;
public GoKart(String color) {
this.color = color;
}
public String getColor() {
return color;
}
public void charge() {
barCount = MAX_BARS;
}
public boolean isBatteryEmpty() {
return barCount == 0;
}
public boolean isFullyCharged() {
return MAX_BARS == barCount;
}
public void drive() {
drive(1);
}
public void drive(int laps) {
int newAmount = lapsDriven += laps;
barCount -= laps;
if(lapsDriven > barCount ) {
throw new IllegalArgumentException("Not enough energy!");
}
barCount = newAmount;
lapsDriven = newAmount;
}
}
2 Answers

Tonnie Fanadez
UX Design Techdegree Graduate 22,796 PointsChidiebere Ekennia sorry I did not clarify why your code wasn't working.
Your if-statement comes when the newAmount and barCount have already been updated to new values. To avoid errors the first thing you want to check is if there is enough energy before trying to decrement the bar count. So the if-statement should come first not after
Your if statement logic is checking if laps driven are more than the remaining bar count. Assuming the cart has just been fully charged and has not taken any lap. Therefore lapsDriven = 0; barCount =8. In this state your code inside if statement will return false (0 is not greater than 8) and throw the IllegalArgumentException yet there is enough barCount to power the cart. So keep it simple and only throw the error when the barCount is less than one - if(barCount < 1){}
You don't need to introduce new variables like the newAmount - Just leave the barCount decrement logic with (-=laps) and increment logic for laps (+=laps) as they are.

Tonnie Fanadez
UX Design Techdegree Graduate 22,796 PointsSee how I did it on the below code; I used the if-statement to check if the barCount is less than one. If it is, an exception is thrown. All you need to fo is to use the keyword throw and creating a new instance of IllegalArgumentException and show the output message.
The if-statement should come before the barCount variable is changed.
public void drive(int laps) {
if(barCount < 1){
throw new IllegalArgumentException("There are not enough bars for this lap");
}
lapsDriven += laps;
barCount -= laps;
}
Let me know if this helps.

Chidiebere Ekennia
5,950 PointsYour code works and i'm grateful but i do not understand the logic. More importantly, i do not understand why mine didn't work. Could you help explain why it didn't work?