Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial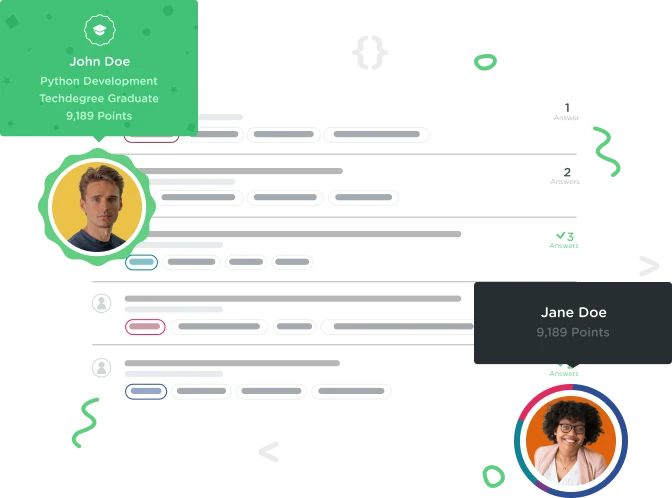

Julie Dowler
7,851 PointsDon't know what's wrong here...
I'm doing the "Extending Object-Oriented PHP" Challenge Task 2 of 3. Here's the instructions: Challenge Task 2 of 3
Step 1: Add an additional array property to the Developer class named $skills that CANNOT be accessed from outside that class, but CAN be accessed by a child.
Step 2: Create a public facing GETTER named "getSkills" that will return the $skills property as an array.
Step 3: Create a public facing SETTER named "setSkills" that will accept an array and set the $skill property to that array value.
So, I entered this code and I get this error: Bummer! The "skills" property should be set to the parameter passed to the method.
I think I'm setting $skills to the parameter passed to the method. Does anyone know what's wrong?
<?php
//do not modify this file
class User {
protected $name;
protected $title;
public function __construct($name = null, $title = null) {
$this->name = $name;
$this->title = $title;
}
public function __toString() {
return $this->getSalutation();
}
public function getSalutation() {
return $this->title . " " . $this->name;
}
public function getName() {
return $this->name;
}
public function setName($name) {
$this->name = $name;
}
public function getTitle() {
return $this->title;
}
public function setTitle($title) {
$this->title = $title;
}
}
<?php
class Developer extends User
{
protected $skills = array();
public function getSkills()
{
return $this->$skills;
}
public function setSkills($anArray)
{
foreach($anArray as $skill)
{
$this->$skills[] = $skill;
}
}
}
//add new child class here
10 Answers
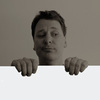
Sean T. Unwin
28,690 PointsRemove the dollar sign($
) from the variable name(s) after $this->...
in each function (get and set).
e.g. $this->skills

Heber Pavon
4,456 PointsHope this helps guys!
class Developer extends User
{
protected $skills = array();
public function getSkills()
{
return $this->skills;
}
public function setSkills($string)
{
$this->skills = $string;
}
}

Xheni Myrtaj
Courses Plus Student 2,636 Points<?php class Developer extends User { protected $skills = array();
public function getSkills() { return $this->skills; }
public function setSkills($array) { $this->skills = $array;
} } //add new child class here
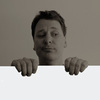
Sean T. Unwin
28,690 PointsIn the body of setSkills()
:
$this->$skills = $anArray;
If you wanted to be robust you could check if $anArray
is, in fact, an array via:
if (is_array($anArray) {
$this->$skills = $anArray;
}
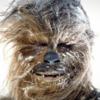
codingchewie
8,764 PointsShouldn't it be $this->skills = $anArray;
without the dollar sign for skills
?

Julie Dowler
7,851 PointsI already tried that. I tried it again just to be sure. It didn't work the second time either. Here's what I entered:
<?php
class Developer extends User
{
protected $skills = array();
public function getSkills()
{
return $this->$skills;
}
public function setSkills($anArray)
{
$this->$skills = $anArray;
}
}
//
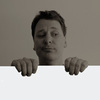
Sean T. Unwin
28,690 PointsFor some reason the Challenge doesn't like the use of $this
when setting the class variable from the setSkills
function.
Using self::$skills = $anArray;
or static::$skills = $anArray;
works, however.
In my OP when I tested the challenge, I think I used static::$skills
out of habit so my apologies there.

Julie Dowler
7,851 PointsThat didn't work. The property $skills was not declared as static, so referring to it as static shouldn't work. The error message says "Use $this to refer to internal properties". I get that error message regardless of whether I use $this or static:: or self::

Chris Craigman
13,659 PointsHere's what worked for me.
<?php
//add new child class here class Developer extends User { protected $skills = array();
public function getSkills() { return $this->skills; }
public function setSkills($skills) { $this->skills = $skills; } }

Andras Guseo
621 PointsHere's my full solution:
<?php
//add new child class here
class Developer extends User {
protected $skills = array();
public function getSkills() {
return $this->skills;
}
public function setSkills( $skills ) {
$this->skills = $skills;
}
function displayUserSkills() {
$name = $this->getName();
$skills = $this->getSkills();
$string = $name . ' has the following skills: ' . implode( ', ', $skills );
return $string;
}
}
$one = new Developer();
$one->setName('Alana');
$one->setSkills( ['PHP', 'MySQL', 'HTML' ] );
//var_dump($one);
echo $one->displayUserSkills();
The trick is that you cannot set the skills one by one, like:
$one->setSkills( 'PHP' );
$one->setSkills( 'MySQL' );
$one->setSkills( 'HTML' );
It needs to be set as an array, like this:
$one->setSkills( ['PHP', 'MySQL', 'HTML' ] );
or the old-fashioned way:
$one->setSkills( array( 'PHP', 'MySQL', 'HTML' ) );
Hope this will be helpful.

Julie Dowler
7,851 PointsThat worked. Thank you!