Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial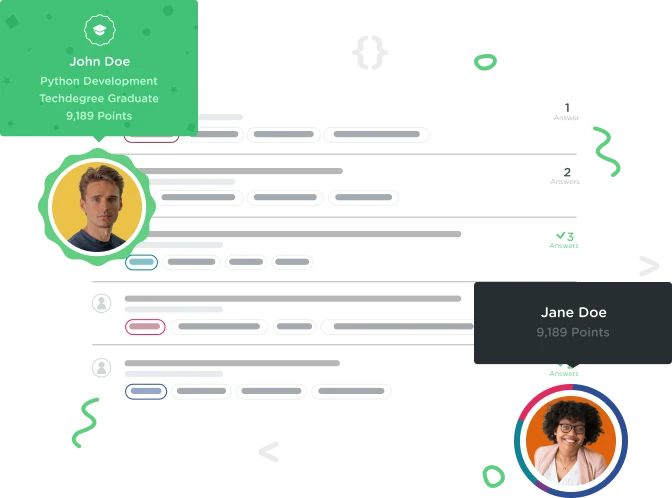
janeporter
Courses Plus Student 23,471 Pointsdon't quite get what she's asking for in the 3rd phase of this challenge.
here is the challenge phase:
Challenge Task 3 of 3
Return an array containing the public lot parameter of each house object whose public roof_color OR wall_color match the passed color parameter.
The error I'm getting: Bummer! Your return value should be an array.
the buttons in the challenge (the Get Help button doesn't work in this phase):
Preview Get Help Reset Code Recheck work
index.php
my code:
<?php
// add code below this comment
class Subdivision
{
public $houses = array();
public function filterHouseColor($color)
{}
}
?>
where should I start??
<?php
// add code below this comment
?>
3 Answers
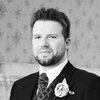
Joel Bardsley
31,249 PointsHi Jane, this particular task has caused a lot of confusion among the community, but hopefully this helps to get you started:
Return an array containing the public lot parameter of each house object whose public roof_color OR wall_color match the passed color parameter.
Although the task used the word parameter, the lot is really a property of the House object, much like the roof_color and wall_color. Since we know from the task that we need to return an array of these lots, we can create a $lots array and return it within the filterHouseColor method:
<?php
// add code below this comment
class Subdivision
{
public $houses = array();
public function filterHouseColor($color)
{
$lots = array();
// Code to check whether the House object's roof_color OR wall_color match the $color parameter
return $lots;
}
}
?>
(If you're unfamiliar with the term 'lot' in this context, think of it as the designated area of land for each house to be built on)
Regarding the House objects themselves, there is already a $houses array available that you can loop through and run a conditional to check the wall_color / roof_color in comparison to the passed $color parameter. If there's a match, that house can be added to the $lots array as shown above.
Hopefully this helps to get you started without giving the answer away.
janeporter
Courses Plus Student 23,471 Pointswow. that worked. thank you so much for your help.
janeporter
Courses Plus Student 23,471 PointsWhen I add this code:
public function filterHouseColor($color)
{
$lots = array();
foreach ($houses as $house) {
if ($house["roof_color"] == $color || $house["wall_color"] == $color) {
$lots[] = $house["lot"];
}
}
return $lots;
}
I get this error:
Bummer! Make sure you are checking both the "roof_color" AND "wall_color" to see if EITHER of them match the passed $color parameter.
What am I doing wrong??
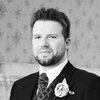
Joel Bardsley
31,249 PointsYou're nearly there! Just remember that you need to use the -> object operator in order to access the properties of an object. As $houses is a property of the Subdivision class, you'll need to access it in your foreach loop as follows:
foreach ($this->houses as $house) {
}
As $houses contains an array of House objects, each $house represents a House object, not an array, so you access its properties via $house->property_name.