Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial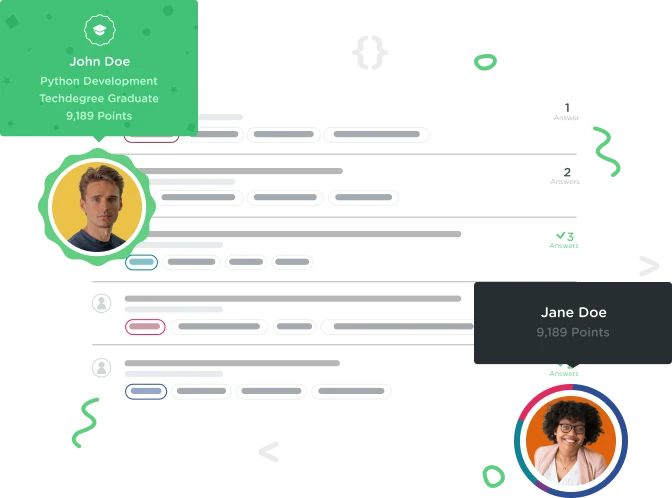

Aditya Puri
1,080 PointsDont really get it...
I don't really get what he is trying to explain..
What is he saying about converting objects of 1 class to objects of another class?? How can we convert 2 different types into each other? And why doesn't it work the other way around?
6 Answers
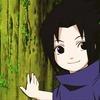
Matthew Francis
6,967 PointsYou are confused with upcasting and extending, let's start with Upcasting:
If you upcast Dog to Animal, no it will not remember the fields from Dog, it will only remember the methods from Dog. This is because you are telling Dog to become an Animal.
public class Main{
public static void main(String []args){
Animal a = (Animal) new Dog();
System.out.println(a.legs); //error
System.out.println(a.teeth); //no error - because a is upcasted to the Animal object
}
}
class Dog extends Animal{
String legs = "10 legs";
void method1(){
}
}
class Animal{
String teeth = "32 teeth";
void method2(){
}
}
Extending: If you only extend but did not upcast anything, yes what you said is correct, Dog will inherit both teeth and legs, this is because you are telling Dog to inherit EVERYTHING from Animal.
public class Main{
public static void main(String []args){
Dog a = new Dog();
System.out.println(a.legs);
}
}
class Dog extends Animal{
String legs = "10 legs";
void method1(){
}
}
class Animal{
String teeth = "32 teeth";
void method2(){
}
}
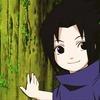
Matthew Francis
6,967 PointsLet's start with upcast:
Upcasting makes the child class to forget everything about it's class properties. In fact, it will obtain every properties of the class Animal. (Note: I said properties, not methods).
class Animal{ //parent class of Dogg
String legs = 2;
}
class Dog extends Animal{ //child class of animal
String legs = 4;
}
Pretend that this is in a main method:
Animal Bob = (Animal) new Dog(); //this is now an Animal class
//Upcasting is automatic, you dont have to include (Animal), it is optional.
if you print Bob, Bob will have 2 legs, not 4.
Now downcasting:
You cannot downcast a parent to a child class, that's a huge no-no. But you can downcast a class that has been upcasted before.
Taking the same example above, you can downcast (Animal) new Dog() into (Dog) new Dog(). Now Bob is back being a dog, and he has his 4 legs back.
Hope this helps :)

Aditya Puri
1,080 Points"Upcasting makes the child class to forget everything about it's class properties." What are properties?
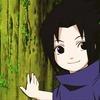
Matthew Francis
6,967 PointsTo put into simple terms, properties are another word for fields for the class.
In this case the property of Dog and Animal is "legs".

Aditya Puri
1,080 Pointsso we can still use the methods of the upcasted class, but the field variables will change?
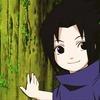
Matthew Francis
6,967 PointsPretty much. But when you cast a class and use it's methods, take account of polymorphism (I believe you have learned this) and super()(you will learn this later, don't worry about it for now).

Aditya Puri
1,080 PointsWhat if one of its methods use a field variable that has now been erased as the class has been upcasted? Will it give an error?
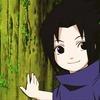
Matthew Francis
6,967 PointsIt's out of the scope and as you mentioned, properties are erased after upcasted, so it will not work.
eg;
public class HelloWorld{
public static void main(String []args){
Animal a = (Animal) new Dog();
a.method1();
}
}
class Dog extends Animal{
String name = "Bob";
void method1(){
}
}
class Animal{
void method2(){
System.out.println(name);
}
}

Aditya Puri
1,080 PointsSo dog will no longer have any field variables because animal doesn't have any fields?
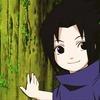
Matthew Francis
6,967 PointsWhoah, this was one month ago. Time flies hahah
But yup, you're right

Aditya Puri
1,080 PointsXD i paused my account..but according to my understanding..dog will still have its fields...
Let's say we have a field called legs in animal class and a field called teeth in dog class..then if we make a dog object which inherits frm animal, won't this new object have both teeth and legs fields?

Elsa Lee
837 PointsThe java documentation on inheritance is very helpful! I have a much clearer picture on what it is about after reading it. This is the link: http://docs.oracle.com/javase/tutorial/java/IandI/subclasses.html, you can also find it under teacher's note.

Fernando Martinez
Android Development Techdegree Student 921 Pointsokay, let see if I got it.!! Animal is a Parent class. Dog is a Child class. Child can be upcast to parent but the original parent can't be downcast to child. if you upcast Dog to animal you can only use animal "methods". Ex. Animal a = (Animal) new Dog(); but if extends Dos class to animal now you can access to animal's methods. Ex. class Dog extends Animal i'm right or wrong ??

Aditya Puri
1,080 Pointsso in upcasting, we have to manually tell the program that Dog is an Animal, like Animal a = (Animal)new Dog();
, and only then we can access the methods.
But if we extend the class in the class declaration itself, by class Dog extends Animal
, we don't need to manually upcast then. We can access the methods by default?
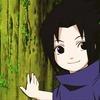
Matthew Francis
6,967 PointsIgnore what I said earlier, I suggest you try playing with this code and you'll get what I mean:

Fernando Martinez
Android Development Techdegree Student 921 PointsLet said if Animal class have an "int number" I can't get access to that propriety through Dog class? Only the methods ?
Aditya Puri
1,080 PointsAditya Puri
1,080 Pointsoh...I am still a little confused but they haven't taught this thing to me yet so maybe I will get a hold of it in upcoming lessons. Thanks!!