Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial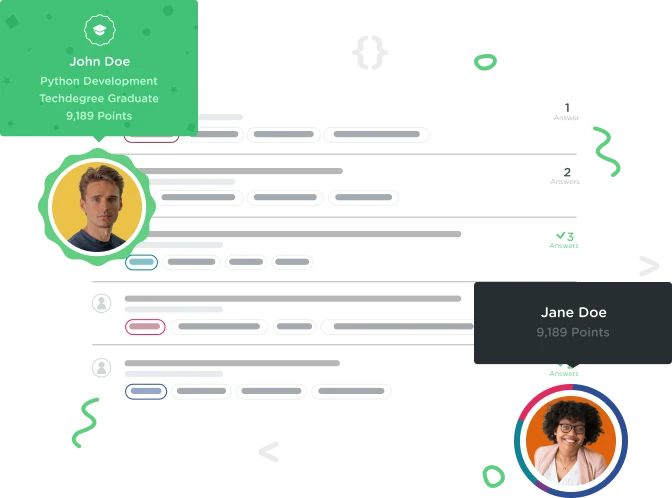

Micheal Jones
3,972 PointsDont really know if Im going about this the right way.
What im I doing wrong here.
const money = 9;
const today = 'Friday'
if ( money > 10 || today === 'Friday' ) {
alert("Its Friday but I dont have enough money to go out.");
}
<!DOCTYPE HTML>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Basics</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
1 Answer
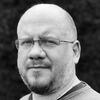
Phil Wright
3,654 PointsHey Michael
There are a couple of different things going on here and the example in the challenge is useful because you can work your way through it, reading the conditional statements and seeing if they make sense.
If they donβt then that is likely where a problem would occur.
I'll add some comments after each conditional statement that describe what each is doing. Hopefully, that will help you see what is happening.
Phil
const money = 9;
const today = 'Friday'
if ( money > 10 || today === 'Friday' ) {
// money is greater than 10 OR today is exactly "Friday"
alert("Time to go to the theater.");
} else if ( money >= 50 || today === 'Friday' ) {
//money is greater or equal to 50 OR today is exactly "Friday"
alert("Time for a movie and dinner.");
} else if ( today !== 'Friday' ) {
// today does not exactly equal "Friday"
alert("It's Friday, but I don't have enough money to go out.");
} else {
// Anything else
alert("This isn't Friday. I need to stay home.");
}
NB: As soon as any condition is met, the code will progress to the relevant alert line and the rest of the script never runs.
Things to consider:
- Remember that
||
in a conditional means OR, this will match any conditions - Where
&&
in a conditional means AND, this must match all conditions - Using the wrong operator might be generating a false positive
- When testing the value of today, make sure you have the right equality operator
Have a look at this instead.
Tweaks made to get the conditionals doing what we want them to.
const money = 9;
const today = 'Friday'
if ( money > 10 && today === 'Friday' ) {
// Must have BOTH more money than 10 AND it be Friday
alert("Time to go to the theater.");
} else if ( money >= 50 && today === 'Friday' ) {
// Must have BOTH at least 50 AND it be Friday
alert("Time for a movie and dinner.");
} else if ( today === 'Friday' ) {
// If you don't have enough money for either of those, but it is Friday
alert("It's Friday, but I don't have enough money to go out.");
} else {
// Last option must not be Friday as we've exhausted our Friday and money options
alert("This isn't Friday. I need to stay home.");
}
Micheal Jones
3,972 PointsMicheal Jones
3,972 PointsThanks man for really breaking it down like this I appreciate it.