Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial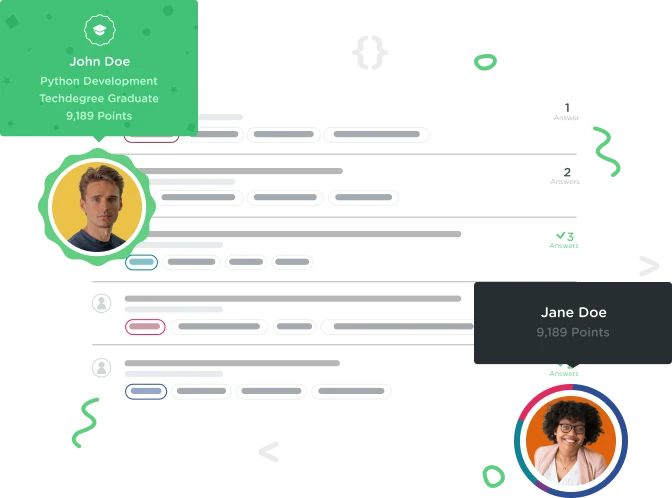

Raul Cisneros
7,319 PointsDont see benefit to arrow functions.
I guess other than being able to use const in the arrow function, I really dont see it being less verbose than the ES5 syntax. Are there other benefits to arrow functions? I went to the MDN website but alot of the lingo in there is still a bit overwhelming.

Raul Cisneros
7,319 PointsI agree. I planned on embracing them as well, I guess I was just looking for a little more insight. Thanks for recommending the flow chart. It will make a good reference.
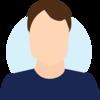
Mike Hatch
14,940 PointsYou're welcome.

Roman Brandt
806 Points???? THIS !!!!!
5 Answers
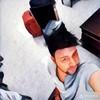
Ari Misha
19,323 PointsHiya there! Well, I've been working with JavaScript for years now and I use Arrow functions literally everywhere. Arrow functions don't just make your code cleaner, it limits the scope of the local variables and local functions inside the same function. Arrow functions don't let the context gets lost to the Global Scope, which simply means it prevents the leaking of the scope. Also, it does the implicit returns which are just a bonus, right? Have you ever seen using this inside arrow functions? Exactly! (;
I hope it helped!
~ Ari

Raul Cisneros
7,319 PointsThank you for your info, it was helpful.

Gary A
3,753 PointsWhen you get into using more function delegates arrow functions are fantastic. At the base level, a function expression is a pointer to the place in memory where a function exists. It's an address.
Let's say you have an array of ages and you want to filter out all those who are legal drinking age in Washington which is 21.
const ages = [12,15,42,11,21,19,93]
( in real life, you'd probably have an array of people coming from a database with their ages )
Well, rather than loop through the ages and do a check, it's better to use a function like filter
. The filter
function expects a function as a parameter. It will fun this function for each piece of content... so in ES5 you'd write it like this:
function isLegalAgeInWA( age ){
return age >= 21;
}
let drinkers = ages.filter( isLegalAgeInWA );
This works, but what happens when you expand to other areas where the drinking age isn't 21? Are you going to write a function for each one? Your code can get pretty chaotic pretty quickly. One way to do this is like so:
// function declaration. returns 21 in the USA and 19 everywhere else.
function getLegalAge( region ){
if (region === 'usa') return 21
else return 19
}
Problem is, this won't work easily as you need to pass a function callback:
// THIS won't work
ages.filter ( isLegalAge( getLegalAge( 'usa' ) )
Now, you COULD create a function for each region isLegalinUSA
isLegalinQuebec
and there are other solutions, but look at how short this complete program is:
const ages = [12,15,42,11,21,19,93]
ages.filter( age => age >= 21 )
You no longer need a separate isLegalAge function which is just reduced to age >= 21
if you want to expand it to be 21 in the USA and 19 everywhere else:
// returns 21 in the US and 19 everywhere else. This would usually be a call to a database or API.
function getLegalAge( region ){
if (region === 'usa') return 21 else return 19 ; // I'd use a ternary expression here, normally
}
let region = 'usa'; // or get user input here
const drinkers = ages.filter( age => age >= getLegalAge( region ) );
Now that's some nice to read code with no specialized utility functions that only exist to be passed to one location.
And you COULD even hard code the values into one line, but it's harder to read and in this case, you're probably going to be getting the legal drinking age from an API call or database table so this is less useful.
const ages = [12,15,42,11,21,19,93]
ages.filter( age => age >= (region == 'usa' ? 21 : 19 ) ) );
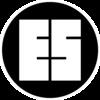
Ephraim Smith
11,930 PointsGary A, thanks for taking the time to spell this out. Much appreciated!
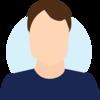
Mike Hatch
14,940 PointsHey Ari Misha,
I was just playing around with an arrow function. I just happen to be going through a tutorial on the subject and was able to figure out a way to solve the issue by using the arrow function. Just a coincidence that I read what you said earlier and thought of it as a way to solve the problem. Take a look if you will:
const tree = {
name: 'The Treehouse',
ari: function() {
this.name = 'Revamped Treehouse'
console.log(this)
const createName = (newName) => {
this.name = newName
}
createName('Ari\'s Treehouse')
console.log(this)
}
}
tree.ari()
Without the createName arrow function it would result in an error.
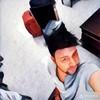
Ari Misha
19,323 PointsMike Hatch It shouldn't throw any errors 'coz its syntactically correct. Just remove the parenthesis when calling the ari function. Using this can get tricky and it trumps even the most experienced programmers. Now, how'd you know what'd this points to? It's simple. It points to the owner Object(instance). Even though JavaScript is a prototypal language, you can see the bits of object-oriented in JavaScript. In the above example, this will always point to the Object's constructor. You can even call the getters and setters and even constructor method on the "tree" variable. I find it pretty amazing tbh. Btw there is no leakage of the scope in your code that's why it shouldn't throw any context errors.
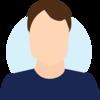
Mike Hatch
14,940 PointsAri, if I just type tree.ari
without invoking the function it will print the function. I'm not sure what you mean.
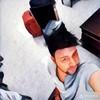
Ari Misha
19,323 PointsMike Hatch lol even I'm not sure why I said it either. I got distracted. My bad. But yeah you're right, and perhaps you might wanna practice writing code in use strict
mode and you might see some interesting results while using this inside Classes and constructor functions. (:

Masha Blair
13,005 Points@Mike Hatch, I think what Ari meant is that you can remove the parenthesis around 'newName' since it's the only argument.

tkati
4,703 PointsI believe other languages refer to this as lambda and when you're using anonymous class / function assignments inline the basic benefit is that you're not "jumping" to another spot in the code to read the details. I think javascript is similar in that not all lambdas will be anonymous, and not all anonymous functions will be lambdas, or I guess arrowscript in this case. Particularly in other languages the old way would be that you'd create a seperate class and/or function, possibly even creating listeners and assigning those funcitons/class to the listener etc. Basically you were writing a lot of "boiler plate" code that was mainly used in one location.
My personal opinion is that shorter code != Always more readable code. Readable code == Readable code. That is sometimes a shorter version will be more readable sometime it won't be.
What helps me in reading => is when I read the => i say to my self "such that". So const square = x => x * x; would be x such that x * x
Mike Hatch
14,940 PointsMike Hatch
14,940 PointsYou're not alone. I'm ambiguous about arrow functions myself. Kyle Simpson created this funny flow chart. I'm going with the flow, though. Modern JavaScript developers seem to be overwhelmingly embracing them. They also seem necessary to know if you want to move into React as well.