Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial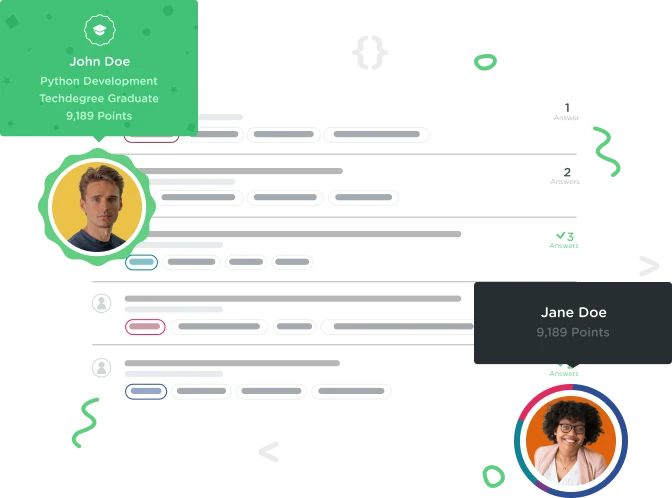

Russell Davidson
2,225 PointsDon't see the temperature
I see the Icon and the day of the week. But not the temperatures that are shown in the video. What am I missing?

Seth Kroger
56,413 PointsWe'd need to at least see your code in order to help.

Kyle Baker
8,211 Points@Seth Kroger Do you know which part the error could be in? I'm guessing Day.java or DayAdapter.java, but I could be wrong. I'll give those and if you need some other sections, please ask. Thank you.
package kybacorps.com.stormy.adapters;
import android.content.Context; import android.view.LayoutInflater; import android.view.View; import android.view.ViewGroup; import android.widget.BaseAdapter; import android.widget.ImageView; import android.widget.TextView;
import kybacorps.com.stormy.R; import kybacorps.com.stormy.weather.Day;
public class DayAdapter extends BaseAdapter {
private Context mContext;
private Day[] mDays;
public DayAdapter(Context context, Day[] days) {
mContext = context;
mDays = days;
}
@Override
public int getCount() {
return mDays.length;
}
@Override
public Object getItem(int position) {
return mDays[position];
}
@Override
public long getItemId(int position) {
return 0;
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
ViewHolder holder;
if(convertView == null) {
convertView = LayoutInflater.from(mContext).inflate(R.layout.daily_list_item, null);
holder = new ViewHolder();
holder.iconImageView = (ImageView) convertView.findViewById(R.id.iconImageView);
holder.temperatureLabel = (TextView) convertView.findViewById(R.id.temperatureLabel);
holder.dayLabel = (TextView) convertView.findViewById(R.id.dayNameLabel);
convertView.setTag(holder);
}
else {
holder = (ViewHolder) convertView.getTag();
}
Day day = mDays[position];
holder.iconImageView.setImageResource(day.getIconId());
holder.temperatureLabel.setText(day.getTemperatureMax() + "");
if(position == 0) {
holder.dayLabel.setText("Today");
}
else {
holder.dayLabel.setText(day.getDayOfTheWeek());
}
return convertView;
}
private static class ViewHolder {
ImageView iconImageView;
TextView temperatureLabel;
TextView dayLabel;
}
}
package kybacorps.com.stormy.weather;
import android.os.Parcel; import android.os.Parcelable;
import java.text.SimpleDateFormat; import java.util.Date; import java.util.TimeZone;
public class Day implements Parcelable { private long mTime; private String mSummary; private double mTemperatureMax; private String mIcon; private String mTimezone;
public long getTime() {
return mTime;
}
public void setTime(long time) {
mTime = time;
}
public String getSummary() {
return mSummary;
}
public void setSummary(String summary) {
mSummary = summary;
}
public int getTemperatureMax() {
return (int)Math.round(mTemperatureMax);
}
public void setTemperatureMax(double temperatureMax) {
mTemperatureMax = temperatureMax;
}
public String getIcon() {
return mIcon;
}
public void setIcon(String icon) {
mIcon = icon;
}
public String getTimezone() {
return mTimezone;
}
public void setTimezone(String timezone) {
mTimezone = timezone;
}
public int getIconId() {
return Forecast.getIconId(mIcon);
}
public String getDayOfTheWeek() {
SimpleDateFormat formatter = new SimpleDateFormat("EEEE");
formatter.setTimeZone(TimeZone.getTimeZone(mTimezone));
Date dateTime = new Date(mTime*1000);
return formatter.format(dateTime);
}
@Override
public int describeContents() {
return 0;
}
@Override
public void writeToParcel(Parcel dest, int flags) {
dest.writeLong(mTime);
dest.writeString(mSummary);
dest.writeDouble(mTemperatureMax);
dest.writeString(mIcon);
dest.writeString(mTimezone);
}
private Day(Parcel in) {
mTime = in.readLong();
mSummary = in.readString();
mTemperatureMax = in.readDouble();
mIcon = in.readString();
mTimezone = in.readString();
}
public Day() {}
public static final Creator<Day> CREATOR = new Creator<Day>() {
@Override
public Day createFromParcel(Parcel source) {
return new Day(source);
}
@Override
public Day[] newArray(int size) {
return new Day[size];
}
};
}
6 Answers

Robert Dandy
3,743 PointsHi Russel,
I was just stuck with this issue but I've managed to figure out a solution.
In DayAdapter.getView() I added a couple of lines...
holder.imgTempCircle = (ImageView) convertView.findViewById(R.id.imgTempCircle);
.....
holder.imgTempCircle.setImageResource(R.drawable.bg_temperature);
and in the ViewHolder class...
ImageView imgTempCircle;
Seems that this forces the image to reload which then allows the text to be positioned on top of it, although I'm far from an expert so this may not be the best solution.
You may need to rename imgTempCircle to whatever you called the ImageView as I didn't use the names which Ben suggested.
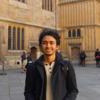
Mohamad Fadhli Ismail
11,935 PointsI came across the problem and this is what I did as well. I think maybe Ben forgot to include it in the video.
Other solution might be hardcode it into the layout but I havent given that a try.
Temperature wont show at first because we align it to the circle. So I guess hardcoded the circle might do the trick?

J A
Full Stack JavaScript Techdegree Student 4,646 PointsWorks great, thank you! Would you mind giving a brief explanation on why this works?

Ni Yao
3,852 PointsThis worked for me, thanks!

Ryan Huai
6,665 Pointsthis worked for me as well. Thanks

Sachin Jayaram
5,461 PointsGood job with the solution :)

DHANANJAY TRIVEDI
Courses Plus Student 403 PointsRobert Dandy! Thanks a lot! I had spent my last 4 days, rewatching the Tutorials, trying to figure out where I might have been wrong! Finally so releaved to fix it! Thanks a lot!

Alwin Lazar V
5,267 Pointsyou just change like this in your Daily Adapter.
holder.circleImageView = (ImageView) convertView.findViewById(R.id.circleImageView); holder.circleImageView.setImageResource(R.drawable.bg_temperature); ImageView circleImageView;
you can check my code below
@Override public View getView(int position, View convertView, ViewGroup parent) { ViewHolder holder;
if (convertView == null) {
//brand new
convertView = LayoutInflater.from(mContext).inflate(R.layout.item_daily_list, null);
holder = new ViewHolder();
holder.iconImageView = (ImageView) convertView.findViewById(R.id.iconImageView);
holder.temperatureLabel = (TextView) convertView.findViewById(R.id.temperatureLabel);
holder.dayLabel = (TextView) convertView.findViewById(R.id.dayNameLabel);
holder.circleImageView = (ImageView) convertView.findViewById(R.id.circleImageView);
convertView.setTag(holder);
}
else {
holder = (ViewHolder) convertView.getTag();
}
Day day = mDays[position];
holder.iconImageView.setImageResource(day.getIconId());
holder.temperatureLabel.setText(day.getTemperatureMax() + "");
holder.dayLabel.setText(day.getDayOfTheWeek());
holder.circleImageView.setImageResource(R.drawable.bg_temperature);
return convertView;
}
public static class ViewHolder {
ImageView iconImageView;
TextView temperatureLabel;
TextView dayLabel;
ImageView circleImageView;
}

Petko Atanasov
Courses Plus Student 3,262 PointsThanks Alwin! Not using circleImageView was strange, but I thought there is a dependency somewhere that I missed.
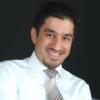
Tareq Alothman
17,921 PointsWhat the video is using in the "daily_list_item.xml" for the image source on "circleImageView" is the
app:srcCompat="@drawable/bg_temperature"
If you change the above line to:
android:src="@drawable/bg_temperature"
This will fix the temperature label, no need to modify any code on the DayAdapter.
Good Luck!

Russell Davidson
2,225 PointsI looked through the text and couldn't find any significant differences. Here is my text for DayAdapter:
package com.example.russty.stormy.adapters;
import android.content.Context; import android.view.LayoutInflater; import android.view.View; import android.view.ViewGroup; import android.widget.BaseAdapter; import android.widget.ImageView; import android.widget.TextView;
import com.example.russty.stormy.R; import com.example.russty.stormy.weather.Day;
/**
- Created by 09250 on 10/6/2016. */
public class DayAdapter extends BaseAdapter {
private Context mContext;
private Day[] mDays;
public DayAdapter(Context context, Day[] days) {
mContext = context;
mDays = days;
}
@Override
public int getCount() {
return mDays.length;
}
@Override
public Object getItem(int position) {
return mDays[position];
}
@Override
public long getItemId(int position) {
return 0; // we aren't going to use this. Tag items fore easy reference
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
ViewHolder holder;
if(convertView == null) {
// brand new
convertView = LayoutInflater.from(mContext).inflate(R.layout.daily_list_item, null);
holder = new ViewHolder();
holder.iconImageView = (ImageView) convertView.findViewById(R.id.iconImageView);
holder.temperatureLabel = (TextView)convertView.findViewById(R.id.temperatureLabel);
holder.dayLabel = (TextView) convertView.findViewById(R.id.dayNameLabel);
convertView.setTag(holder);
} else {
holder = (ViewHolder) convertView.getTag();
}
Day day = mDays[position];
holder.iconImageView.setImageResource(day.getIconId());
holder.temperatureLabel.setText(day.getTemperatureMax() + "");
holder.dayLabel.setText(day.getDayOfTheWeek());
return convertView;
}
private static class ViewHolder {
ImageView iconImageView; // public by default
TextView temperatureLabel;
TextView dayLabel;
}
}
and here is my text for Day.java:
package com.example.russty.stormy.weather;
import android.os.Parcel; import android.os.Parcelable;
import java.text.SimpleDateFormat; import java.util.Date; import java.util.TimeZone;
/**
-
Created by 09250 on 9/13/2016. */ public class Day implements Parcelable { private long mTime; private String mSummary; private double mTemperatureMax; private String mIcon; private String mTimezone;
public long getTime() { return mTime; }
public void setTime(long time) { mTime = time; }
public String getSummary() { return mSummary; }
public void setSummary(String summary) { mSummary = summary; }
public int getTemperatureMax() { return (int)Math.round(mTemperatureMax); }
public void setTemperatureMax(double temperatureMax) { mTemperatureMax = temperatureMax; }
public String getIcon() { return mIcon; }
public void setIcon(String icon) { mIcon = icon; }
public String getTimezone() { return mTimezone; }
public void setTimezone(String timezone) { mTimezone = timezone; }
public int getIconId() { return Forecast.getIconId(mIcon); }
public String getDayOfTheWeek() { SimpleDateFormat formatter = new SimpleDateFormat("EEEE"); formatter.setTimeZone(TimeZone.getTimeZone(mTimezone)); Date dateTime = new Date(mTime * 1000); return formatter.format(dateTime); }
@Override public int describeContents() { return 0; }
@Override public void writeToParcel(Parcel dest, int flags) { dest.writeLong(mTime); dest.writeString(mSummary); dest.writeDouble(mTemperatureMax); dest.writeString(mIcon); dest.writeString(mTimezone); }
private Day(Parcel in) { mTime = in.readLong(); mSummary = in.readString(); mTemperatureMax = in.readDouble(); mIcon = in.readString(); mTimezone = in.readString(); }
public Day() {}
public static final Creator<Day> CREATOR = new Creator<Day>() { @Override public Day createFromParcel(Parcel source) { return new Day(source); }
@Override public Day[] newArray(int size) { return new Day[size]; }
}; }

Jason Force
7,508 PointsIt's a little frustrating that it's been 11 months since this post was made and this discrepancy still exists in the course.

Raveesh Agarwal
2,994 PointsPlease let me explain this, for after much experimentation I have come to some conclusions. Seth Kroger sir, please corroborate or correct my findings with your guidance.
Here is my adapter before the circle background and temperature icons were visible.
package com.example.raveesh.stormy.adapters
import android.content.Context
import android.view.View
import android.view.ViewGroup
import android.widget.BaseAdapter
import com.example.raveesh.stormy.R
import com.example.raveesh.stormy.weather.Day
import kotlinx.android.synthetic.main.daily_list_item.view.*
import org.jetbrains.anko.imageResource
import org.jetbrains.anko.layoutInflater
class DayAdapter(mContext: Context,
private val mDays: Array<Day>) : BaseAdapter() {
private val inflater = mContext.layoutInflater
lateinit var view :View
override fun getItem(position: Int): Any = mDays[position]
override fun getCount(): Int = mDays.size
override fun getItemId(position: Int): Long = 0//not used
override fun getView(position: Int, convertView: View?, parent: ViewGroup?): View {
view =
if (convertView == null) inflater.inflate(
R.layout.daily_list_item,
parent,
false
) else convertView
view.iconImageView.imageResource = mDays[position].getIconId()
view.temperatureLabel.text = mDays[position].mTemperatureMax.toInt().toString()
view.dayNameLabel.text = mDays[position].getDayOfTheWeek()
return view
}
}
As we can see, circleImageView has not been initialized, this could be one of the reasons why.
Here is the adapter that works perfectly:
package com.example.raveesh.stormy.adapters
import android.content.Context
import android.view.View
import android.view.ViewGroup
import android.widget.BaseAdapter
import com.example.raveesh.stormy.R
import com.example.raveesh.stormy.weather.Day
import kotlinx.android.synthetic.main.daily_list_item.view.*
import org.jetbrains.anko.imageResource
import org.jetbrains.anko.layoutInflater
internal class DayAdapter(mCtx: Context,
private val mDays: Array<Day>) : BaseAdapter() {
private val mInflater = mCtx.layoutInflater
private lateinit var row : View
override fun getItem(position: Int): Day = mDays[position]
override fun getCount(): Int = mDays.size
override fun getItemId(position: Int): Long = 0//not used
override fun getView(position: Int, convertView: View?, parent: ViewGroup?): View{
row = convertView ?: mInflater.inflate(R.layout.daily_list_item, parent, false)
/*make sure to initialize each and every view in the order you want drawn.
use an if-else block and put the non reetetive assignments into the if null block but need
to initialise at least once:->
*/
row.dailyCircleImageView.imageResource = R.drawable.bg_temperature
row.dailyTemperatureLabel.text = "${Math.round(mDays[position].mTemperatureMax)}"
row.dailyIconImageView.imageResource = mDays[position].getIconId()
row.dailyNameLabel.text = mDays[position].getDayOfTheWeek()
return row
}
}
It works because I made sure to initialise every single view. It seems that for the first time, we need to initialise every component view inside the view that we return, or else that remains null.
Also note, that I have changed the names of the views because our tooling (Android Studio) was importing the circleImageView and temperatureLabel from the activity_main.xml. So having separate names also helped.
I guess there is still room for improvement where I could change the ?: operator back into the if-else block and move all the parts of the assignments that don't change into the if block (for example: row.dailyCircleImageView.imageResource = R.drawable.bg_temperature)
These are my personal assumptions, please let me know if I'm wrong.
Kyle Baker
8,211 PointsKyle Baker
8,211 PointsI second this, and I don't see any typos in my code yet. If anyone needs more info to help us, please ask what you need.