Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial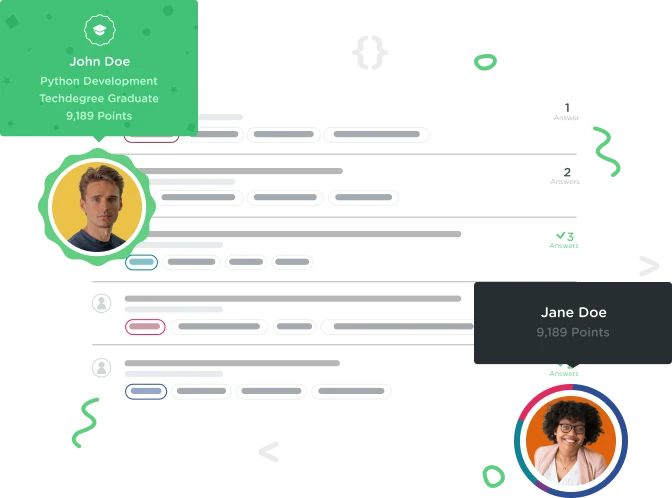

Adam Chez
772 Pointsdon't understand the variable part
no details
function returnValue(argument) {
return argument;
}
returnValue("Hello");
var echo = returnValue();
<!DOCTYPE HTML>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>JavaScript Basics</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
2 Answers
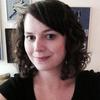
Kaleena Perkins
14,792 PointsHi,
I'm not sure what that challenge is because it doesn't say. However, I have some ideas that might help explain functions, arguments, and return values. A variable holds a value. It helps to think of it as a box that you can put something in. If you have a box named "kitchenSupplies" you can put a lot of different things in the box but the box still has the label "kitchenSupplies".
//Making (declaring) a variable
var kitchenSupplies = "spoon";
//now the variable kitchenSupplies holds the value of "spoon"
var kitchenSupplies = "spatula";
//the same variable, but now holds value of "spatula", the "spoon is thrown away"
A function is a reusable bit of code. If you had a program in which you were continually needing to add numbers together, it would be very useful to have a way to do that. The way JavaScript does it is to create a function. The function uses placeholders, (arguments), so that we can use (call) the function again and again without having to immediately know what numbers we will be adding. We have to give the function a return value so it knows what to do at the end of the function and doesn't just throw away what it did.
// Defines the function and tells the computer that the function will have
//two arguments (number1 and number2)
function addStuff(number1, number2){
//Adds the numbers together and stores it in a variable called "total"
var total = number1 + number2;
//returns the variable "total"
return total;
}
// "Call" the function to use it and "pass in" the arguments,
//in this case the stuff you want to add together
// this will "return" the value 10
addStuff(5,5);
// For the code you have above:
function returnValue(argument){
return argument
};
var echo = "Hello"
returnValue(echo)
//This will return the value of the argument you passed in. In this case it will return "Hello"
I hope this explains variables, functions, arguments, and return values. Let me know if it you have more questions! Take care, Kaleena
P.S. If you like this answer, please upvote it or mark it 'best answer.' It would sure help me out a lot! :)
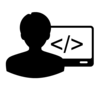
Abe Layee
8,378 PointsThe challenge is asking to pass the returnValue() function to the echo variable. let start by creating the function
function returnValue(){};
next pass a variable into the parameter and return the argument we passed into curly braces.
function returnValue(argument) {
return argument.
};
lastly, create the echo var outside the function
var echo = returnValue("Hello");