Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial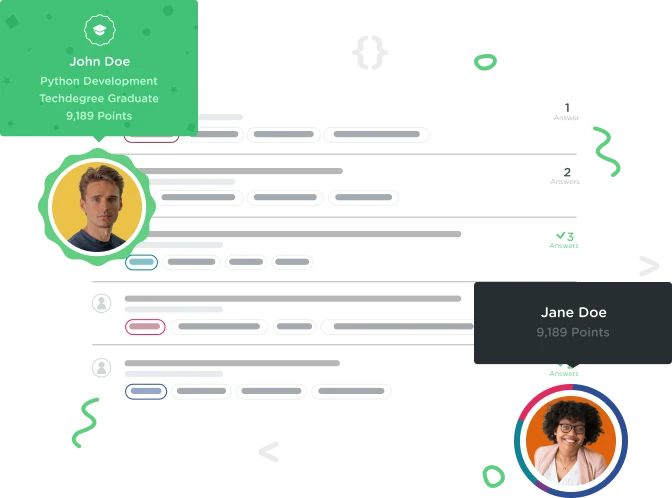

Mark Lovett
15,853 PointsDont understand this question - can anyone shed some light?
The genericGreet function doesnt appear to be part of an object like the examples.
'''var greeting = andrew.genericGreet.apply(andrew, args1);'''
4 Answers

Dino Paškvan
Courses Plus Student 44,108 PointsThe correct answer is the first code snippet in my reply:
var greeting = genericGreet.apply(andrew, args1);
You attempted to call genericGreet
as if it was a method on andrew
, but there is no such method, so the challenge doesn't pass.
When you use .apply()
on a function, that function will act like a method on the object that you pass as the first parameter to .apply()
:
var someVariable = myFuntion.apply(objectThatMyFunctionWillActAsAMethodOn, parametersForMyFunctionInTheFormOfAnArray);

Dino Paškvan
Courses Plus Student 44,108 PointsIt doesn't need to be a part of the object. The important thing is that the function tries to output this.name
. this
can be made to point at a certain object.
When you use Function.prototype.apply
, you pass two parameters. The first one gets explicitly bound as the value of this
inside the function that it was called on. The other is an array of parameters.
var greeting = genericGreet.apply(andrew, args1);
The same is true for Function.prototype.call
, only it doesn't take an array parameters, you just list them one by one.
The value of this
inside a function can be bound in four ways. If you use a constructor, this
gets bound to the object that's created:
function MyConstructor(someValue) {
this.someValue = someValue;
this.myMethod = function () {
console.log(this.someValue);
};
}
var myObject = new MyConstructor(); // binding through the use of a constructor
myObject.myMethod(); // logs myObject.someValue
You can explicitly bind it by using .call()
, .apply()
or .bind()
. In this case, the function will behave as if it was a method on the object that you passed as the first parameter. An example of that is this code challenge.
It can get implicitly bound, if the function is called in the context of an object:
var someFunction = function() {
console.log(this.someValue);
};
var myObject = {
someValue: "some text",
someMethod: someFunction // implicit binding
};
myObject.someMethod(); // logs myObject.someValue
And finally, if none of these scenarios apply, this
is bound to the global object (inside a browser, that would be the window
object). Or if you are running in strict mode, then it's undefined
.

Mark Lovett
15,853 PointsDino, Thank you for taking the time to give all this valuable info, although your answer is still a little over my head right now. This challenge came before the constructor concept was introduced so it's a little fuzzy for me now. If you could simplify your answer, and give me a brief explanation of what is wrong with my answer, and what the correct answer is, I would really appreciate it. Thanks much, Mark

Mark Lovett
15,853 PointsNow that I understand! Thanks very much.