Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial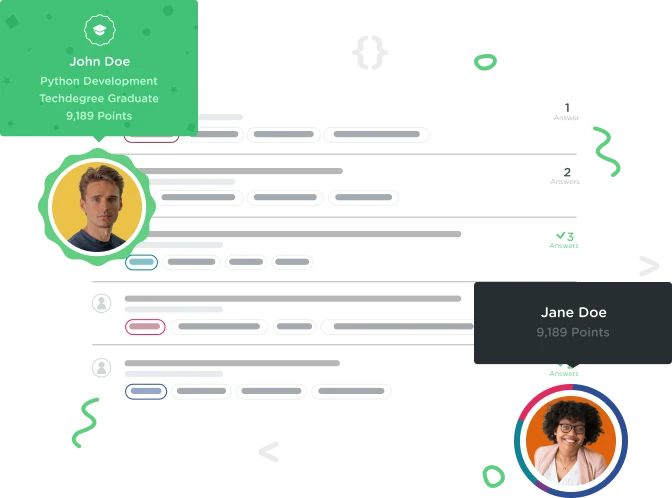

Emily Strobl
5,429 Pointsdon't understand what the task is asking
I'm confused on what I'm suppose to do
class Letter:
def __init__(self, pattern=None):
self.pattern = pattern
class S(Letter):
def __init__(self):
pattern = ['.', '.', '.']
super().__init__(pattern)
2 Answers
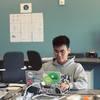
Maxwell Newberry
7,693 PointsThey want you to create a method within the Letter
class. Which you can add right underneath the first __init__
method.
class Letter:
def __init__(self, pattern=None):
self.pattern = pattern
def __str__(self):
Then we want to initialize a string to return that will eventually contain our pattern in text form.
class Letter:
def __init__(self, pattern=None):
self.pattern = pattern
def __str__(self):
return_string = ""
Once we have that, we need to create for-loop that will iterate through the entire pattern, item by item, so that we can check whether it is a .
or _
and change the content to text form of dot
or dash
.
class Letter:
def __init__(self, pattern=None):
self.pattern = pattern
def __str__(self):
return_string = ""
for index, item in enumerate(self.pattern):
# iterate through each item in pattern
The next part is to check whether the current item is a dot or dash and then add that item to the return string.
class Letter:
def __init__(self, pattern=None):
self.pattern = pattern
def __str__(self):
return_string = ""
for index, item in enumerate(self.pattern):
# iterate through each item in pattern
if item == '.':
# dot
return_string += "dot"
else:
# dash
return_string += "dash"
Then, the final step is to separate each item by a hyphen. But, we don't want to add a hyphen to the last item so we need to check whether the item we're on is the last item or not using an if-statement. The following code does this after we add the text, note the indentation of this if-statement, it is within the for-loop, but outside of the if-statement. This is because we want to do this at the end of loop iteration each item.
class Letter:
def __init__(self, pattern=None):
self.pattern = pattern
def __str__(self):
return_string = ""
for index, item in enumerate(self.pattern):
# iterate through each item in pattern
if item == '.':
# dot
return_string += "dot"
else:
# dash
return_string += "dash"
if index != len(self.pattern)-1:
# separate with hyphen if not final index
return_string += "-"
return return_string
Return your final string and you're done!

nikel Hayo
1,943 Pointshi, indentation at the last line(return return_string) should be at the same line with "for" above.