Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial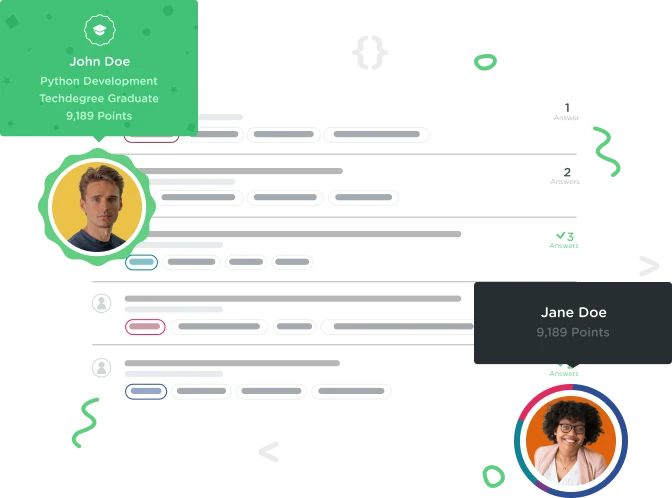
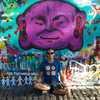
Andrew Stelmach
12,583 PointsDon't understand what's asked in the Extra Credit
Can a member of staff clarify what is being asked in the Extra Credit?
"expand on that by asking the user how many iterations they would like to run and then sorting the output from the quickest to slowest finding."
I understand the iterations part, but what do you mean by 'sorting the output from the quickest to the slowest finding.'?
4 Answers
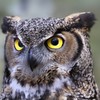
avremel
8,448 PointsWorking off Andrew's code I have managed to make the code run with a local variable for "findings". I have also modified the printing of "findings" to use the ".each" method.
class SimpleBenchmarker
def self.go(how_many=1)
findings = []
puts "--------------Benchmarking started------------------"
start_time = Time.now
puts "Start Time: \t#{start_time}\n\n"
how_many.times do
print "."
time = rand(0.1..1.0)
sleep time
findings.push time
end
print "\n\n"
end_time = Time.now
puts "End Time:\t#{end_time}\n"
puts "-------------Benchmarking finished-------------------"
puts "Result:\t\t#{end_time - start_time} seconds"
print "\n\n"
findings = findings.sort!
puts "The lengths of the intervals, shortest to longest, were:"
print "\n"
findings.each do |item|
puts "#{item} seconds"
end
end
end
puts "How many iterations would you like to run?"
iterations = gets.to_i
SimpleBenchmarker.go iterations

Unsubscribed User
3,309 PointsSince how_many was already being passed to the method I left the entire SimpleBenchmarker as-is and just added this at the end:
print "How many times would you like the program to run? "
how_many = gets.to_i
result = []
SimpleBenchmarker.go how_many do
time = rand(0.1..1.0)
sleep time
result.push time
end
puts "\nThe benchmark times were, in ascending order: \n\n"
result.sort.each { |time| puts "#{time} seconds" }
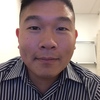
Yi Ren
4,990 PointsThis is my answer without using global variables. It works. I hope this is the right answer:
class SimpleBenchmarker
def self.go(how_many=1, &block)
print "How many times do you want to run? "
iteration = $stdin.gets.to_i
sorted_run_time = []
i = 0
until i == iteration do
puts "------------Benchmarking started--------------"
start_time = Time.now
puts "Start Time:\t#{start_time}\n\n"
how_many.times do |a|
print "."
block.call
end
print "\n\n"
end_time =Time.now
puts "End Time:\t#{end_time}\n"
puts "-----------Benchmarking finished-----------------\n\n"
result = end_time - start_time
puts "Result:\t\t#{end_time - start_time} seconds"
i += 1
puts "Performing test #{i}"
sorted_run_time.push result
end
puts "The result is #{sorted_run_time.sort!}"
end
end
SimpleBenchmarker.go 6 do
time = rand(0.1..1.0)
sleep time
end

Jason Seifer
Treehouse Guest TeacherHey Andrew Stelmach you're right! The extra credit is meant to keep track of the lengths of times it takes to run each benchmark and then print it out in a sorted way. Try seeing if you can do it without global variables :)
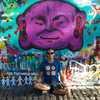
Andrew Stelmach
12,583 PointsI have! (And failed!). I spent several hours trying to work this out for myself (and also scouring the web for info and help. All to no avail. I'm just not prepared to do that again without a hint or two. I would really appreciate some help to at least narrow things down.
Andrew Stelmach
12,583 PointsAndrew Stelmach
12,583 PointsI've made the assumption that they mean sorting the time intervals from shortest to longest and displaying them.
I've made this code, and it works, BUT I resorted to creating a global variable, because I couldn't make it work without doing that. I don't understand Scope well enough and I need some pointers.
I experimented with putting the 'findings' array variable in various places, along with the .push in various places, too. But something always went wrong - some sort of MethodError or some problem with Scope I think (the 'findings' variable not being available).
So, in the end I just thought 'sod it' and just made 'findings' a global variable, '$findings'. It works and it made things easy, but I'm sure it must be sloppy practice?
Please help me clear this up.
My code: