Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial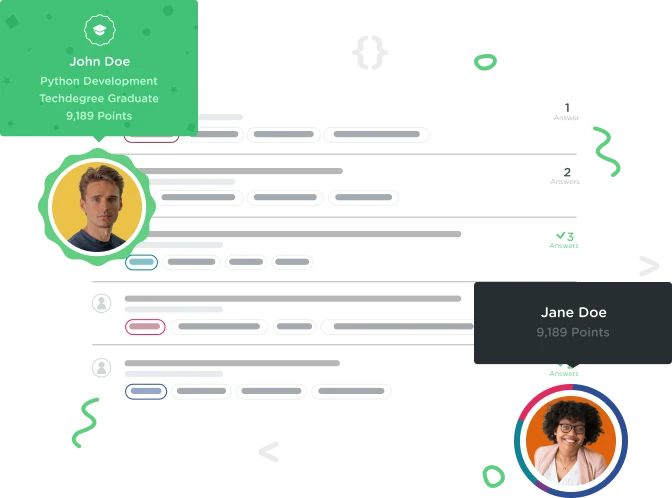
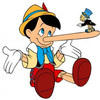
Jonathan Dell'Ova
Courses Plus Student 6,283 PointsDon't understand why there I get "TypeError: 'str' object cannot be interpreted as an integer" in my code
from Yatzy import dice
class Hand(list):
def __init__(self, size=0, die_class=None, *args, **kwargs):
if not die_class:
raise ValueError("You must provide a die class!")
super().__init__()
for _ in range(size):
self.append(die_class())
def __len__(self):
str_num = str(super().__len__())
return "There are {} die in this hand".format(str_num)
hand = Hand(size=5, die_class=dice.D6)
print(hand)
print(len(hand))
When I try to run this code it gives me this error:
TypeError: 'str' object cannot be interpreted as an integer
I've tried analyzing the problem with is instance()
for example but I've not come to any conclusions where the problem lies.
I know that print()
can take a string as an argument. I also know that if I check the instance of super()__len__()
I get back True
when I check for an int. If I then do str(super()__len__())
and check what type of instance it is, I get back True
for a string and False
for an int. When I then return it to be used in print()
I get this weird error.
What is happening here?
This is just something extra I wanted to add to keep practicing with overwriting inbuilt methods but I would really like to learn from this.
1 Answer
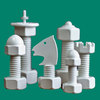
Steven Parker
241,807 PointsThe override of "__len__
" is returning a string message, but the system expects it to return a numeric value.
Did you perhaps intend to override "__str__
" instead using this code?
Jonathan Dell'Ova
Courses Plus Student 6,283 PointsJonathan Dell'Ova
Courses Plus Student 6,283 PointsYes, this was the issue. I didn't realize that the system was expecting a numeric value that had to be filled.
Overriding
__str__
with the exact same syntax for the body made it work when usingprint(hand)
and alsoprint(str(hand))
which obviously give the same output.Thanks!
Steven Parker
241,807 PointsSteven Parker
241,807 PointsThat's right, because "print" implicitly performs string conversion so you get the same output either way.