Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial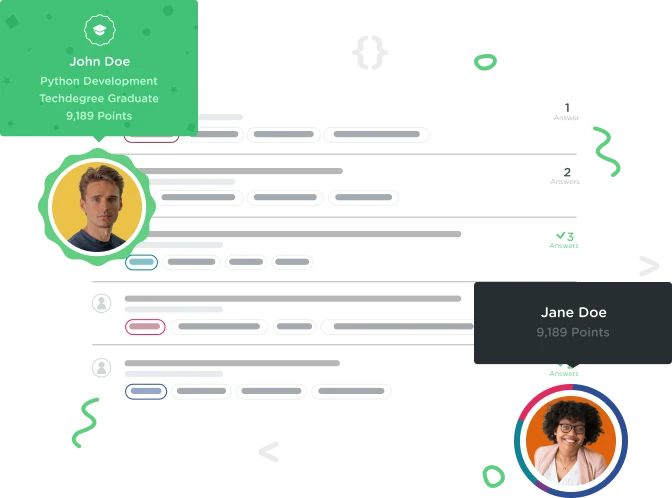

Jackson Whang
1,418 PointsDot notation versus parenthesis?
I'm confused by the step we take after we create a struct or class. We often subsequently create a variable or constant and assign to it some instance of the object we just created. For example:
struct User {
var fullName: String
var email: String
var age: Int
}
let someUser = User(fullName:.........)
When dealing with the contents of this constant, in this case someUser, I don't understand when we use dot notation and when we use the parenthesis. It seems like sometimes we say:
let someUser = User(fullName: "Bob Jones", ......)
and other times we say:
let someUser = User.fullName()
or something along those lines.
Can someone explain what these respective notations do/mean? Thank you!
2 Answers
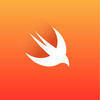
Steven Deutsch
21,046 PointsHey Jackson Whang,
You use dot notation when you are accessing a property or method of an object.
For example:
var array = [1, 2, 3]
array.append(4)
// Here we use dot syntax to access the append method on Array
let count = array.count
// Here we use dot syntax to access the count property on Array
As for parenthesis... you'll see them when:
// Define a method/function
func printThis(text: String) {
print(text)
}
// You call a function/method
print("Hello World")
// You use an initializer (also a function but a special one)
let user = User(name: "A Name")
// Tuples
let tuple: (Int, String) = (1, "String")
// Evaluating Expressions
let result = (1 + 1) == 2
Hope this clears somethings up
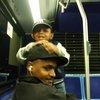
Xavier D
Courses Plus Student 5,840 PointsHey Jackson Whang,
I created a struct example based on your info.
struct User
{
var firstName: String
var lastName: String
var email: String
var age: Int
func fullName() -> String
{
return firstName + " " + lastName
}
}
Now that the struct has been declared, I will show you when I use parentheses and dot notation when working on this struct.
First I want to use the struct and put your info in it...
let someUserInstance = User(firstName: "Jackson", lastName: "Whang", email: "jwhang@email.com", age: 20)
Above, I created an instance of the struct so I can put your info in it and stored the instance in the someUserInstance constant. I had to use the parentheses in order to access the stored properties of the struct so that I can put in your name, email and age; Xcode autocompleted the fields of the stored properties for me so that I can fill in your data--Xcode will autocomplete right after you type the open-parenthesis...
let someUserInstance = User(
Before I could work with a class or struct, I had to create an instance and store it in a variable or constant. Therefore I had to first use the parenthesis before using dot notation.
Now that the instance of the User struct has been created and stored in the someUserInstance, I can now work with the User struct by using dot notation on its instance--I cannot work directly with the User struct, but I can work on it's instance like below...
someUserInstance.fullName()
let displayedAndStoredYourFullNameConstant = someUserInstance.fullName()
Here, I used the dot notation on the someUserInstance in order to access the struct's fullName method--I wanted to access this method in order to display your full name. I accessed the method twice, and on the second time, I displayed your full name and then stored the data in the retrievedAndStoredYourName constant. I also had to type out parentheses at the end of the method name because it's required by the syntax and also the parentheses are part of the method name, if you look inside the body of the struct. Xcode would give an error if I left out the parentheses and just put...
someUserInstance.fullName // this would give an error
Below, I going to use dot notation without any parenthesis in order to work with struct (by working with it's instance) and pull data from it...
someUserInstance.firstName
someUserInstance.lastName
let jacksonWhangEmail = someUserInstance.email
let jacksonWhangAge = someUserInstance.age
Here I used dot notation followed by the name of the struct's stored properties to display your name email and age. I also stored your email and age data in two constants. Hope this helps you when using parentheses vs dot notation...