Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial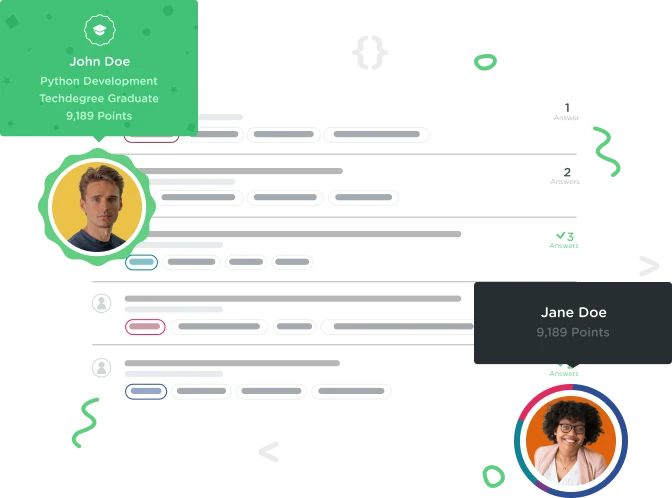

Adam Beck
9,345 PointsDouble check your implementation for the Down direction and make sure the location's y property decreases by 1
Can someone help me out with this problem? This code doesn't feel right but works in Xcode. Perhaps I don't understand the question, but I would love some feedback. Thank you!
class Point {
var x: Int
var y: Int
init(x: Int, y: Int){
self.x = x
self.y = y
}
}
class Machine {
var location: Point
init() {
self.location = Point(x: 0, y: 0)
}
func move(direction: String) {
print("Do nothing! I'm a machine!")
}
}
class Robot: Machine {
override func move(direction: String) {
let direction: String = "Up"
location = Point(x: 0, y: 1)
switch direction {
case "Down":
location = Point(x: 0, y: -1)
case "Left":
location = Point(x: -1, y: 0)
case "Right":
location = Point(x: 1, y: 0)
default:
break
}
}
}
1 Answer

Keli'i Martin
8,227 PointsYour code is not actually changing the x and y coordinate by 1. You are really just saying that the point is one of four different points: (0, 1), (1, 0), (0, -1), or (-1, 0). You really need to be taking the point of that object and increasing or decreasing either x or y by 1.
Say, for example, you have a Robot object, named robot1. At a certain point in time, robot1's location is set to (4, 3) and you call the func() method and pass in "Left". Your code currently would make the location (-1, 0), when really you want the new location to be (3, 3) (passing "Left" should decrease your x-coordinate by 1).
Your code really needs to be doing things like location.x -= 1
or location.y += 1
, depending on what direction is passed into the function.
Hope this helps!