Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial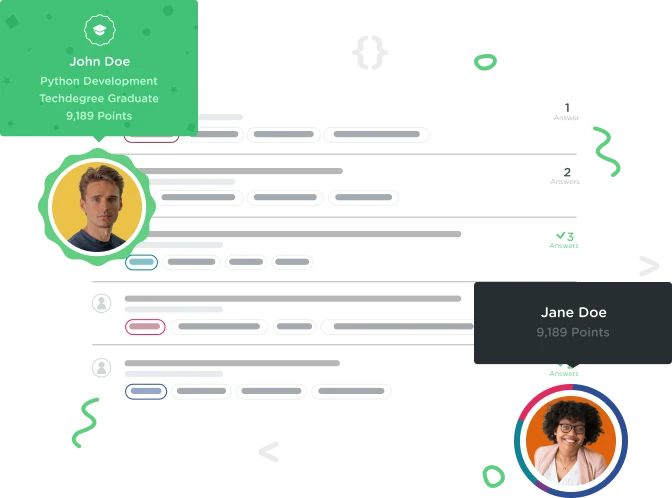

Adam Williams
4,215 Points"Double Quotes" vs 'Single Quotes'
I always thought the choice between using double quotes and single quotes in your Javascript was upto you but I've come across a strange occurrence in this exercise.
Note: nothing to do with the JSON file, I know that requires double quotes.
This code runs perfectly:
var xhr = new XMLHttpRequest();
xhr.onreadystatechange = function () {
if(xhr.readyState === 4) {
var employees = JSON.parse(xhr.responseText);
var statusHTML = '<ul class="bulleted">';
for (var i=0; i < employees.length; i++) {
if (employees[i].inoffice === true) {
statusHTML += '<li class="in">';
} else {
statusHTML += '<li class="out">';
}
statusHTML += employees[i].name;
statusHTML += "</li>"
}
statusHTML += "</ul>";
document.getElementById("employeeList").innerHTML = statusHTML;
}
};
xhr.open("GET", "data/employees.json");
xhr.send();
Yet this code throws up the error "Unexpected Identifier" on Line 5.
var xhr = new XMLHttpRequest();
xhr.onreadystatechange = function () {
if(xhr.readyState === 4) {
var employees = JSON.parse(xhr.responseText);
var statusHTML = "<ul class="bulleted">";
for (var i=0; i < employees.length; i++) {
if (employees[i].inoffice === true) {
statusHTML += "<li class="in">";
} else {
statusHTML += "<li class="out">";
}
statusHTML += employees[i].name;
statusHTML += "</li>"
}
statusHTML += "</ul>";
document.getElementById("employeeList").innerHTML = statusHTML;
}
};
xhr.open("GET", "data/employees.json");
xhr.send();
Weirdly also, if I remove the class attributes from lines 5, 8 & 10 the script runs perfectly fine, just without the CSS styling of course.
Can anybody shed some light on this?
1 Answer
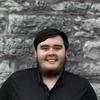
Michael Hulet
47,913 PointsIn general, it is your choice whether you want to use single or double quotes, but a reason you might choose to use single quotes instead of double quotes is exposed directly by this example. Look at the difference in syntax highlighting in the code between your two examples. On its own, JavaScript can't distinguish between double quotes that you want to be in the HTML you're writing (which requires double quotes) and the double quotes to wrap your JavaScript string. It's not the only example in your code, look at line 5. See how the purple in your first example lasts until the semicolons unbroken, and see how there are 2 separate blocks of purple on the same line in the second example? That's because in the second example, JavaScript sees a string "<ul class="
, then a variable bulleted
, then a string ">"
. It tells you that there's an unexpected identifier because it sees 2 different strings and nothing but a variable in between, and it wants you do do something to combine those, like put plus signs in between. However, you want one string containing all of that, so you have 2 options: you can either use single quotes to denote the string, or you could tell JavaScript that you really want those double quotes on the inside to be part of the string by escaping them, like this:
var statusHTML = "<ul class=\"bulleted\">";
//Notice the backslashes. They won't be in the final string, but the double quotes after them will
Adam Williams
4,215 PointsAdam Williams
4,215 PointsHi Michael,
Thanks very much for your clear answer. That make sense now :)