Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial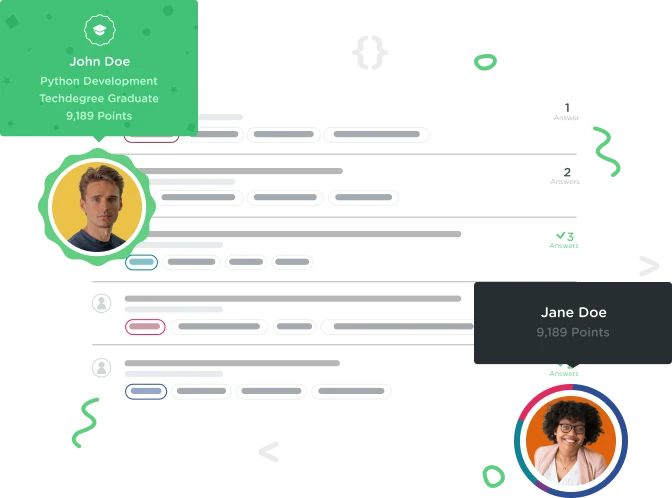
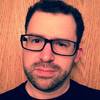
Chris Shaffer
12,030 Pointsdo/while loop boolean question
I'm unclear why the condition we set for the do/while loop in this exercise is (! correctGuess)
. This effectively changes the var correctGuess = false
to "true".
I don't understand why saying, "do this while this is true" results in the code executing over and over.
Logically, it would seem, "do this while this is FALSE" would be the idea, right?
I'm just going back and doing a refresher in JS before going through the JS Stack track and this is breaking my brain.
Kirby Abogaa
3,058 Pointsthey should change it to "Do/Until"...LoL
i got stuck on the same and I am thankful to have read this...
i felt very very lost for a moment...
Cheers!
2 Answers
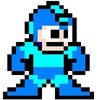
Robert Richey
Courses Plus Student 16,352 PointsHi Chris,
Conditional checks in loops can be hard to reason about. The way I think of this, is, while the guess is not correct, keep looping - keep asking for a number until user makes a correct guess.
var correctGuess = false;
do {
// code
} while (!correctGuess); // while it's not a correct guess, loop
Another way of writing this could be:
var wrongGuess = true;
do {
// code
} while (wrongGuess); // while it's a wrong guess, loop

Ayush Bahuguna
2,092 PointsHi Robert Richey. So does that mean " ! " does not alter the predefined value of correctGuess ?
Also, can we use this code instead of that stated by the instructor?
while ( correctGuess === false )
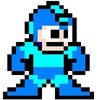
Robert Richey
Courses Plus Student 16,352 PointsHi Ayush,
Correct. !
does not change the variable's value, instead it inverts the boolean expression resulting from the variable (perhaps more confusing that it should be...).
For example
var orangesAreOrange = true;
!orangesAreOrange; // results in an expression with the value of false
orangesAreOrange; // still true
The problem with while ( correctGuess === false )
is that if during the first iteration of the loop (do ... while loops always have at least one iteration) correctGuess is set to true
, you would have an endless loop. What would make your code work is to add a bang !
to invert the value of the expression.
while ( ! ( correctGuess === false ) )
which is a very complicated way of writing while ( !correctGuess )
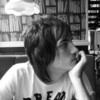
Colin K
3,417 PointsRobert could you explain why you would have an endless loop if you used ( correctGuess === false ) ?
Thanks.
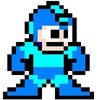
Robert Richey
Courses Plus Student 16,352 PointsHi Colin,
Great question and observation! I must have still been half asleep when responding - I need to stop doing that!
Looking back on the question and related video, I'm not really sure how I arrived at the conclusion. I understand coding can be confusing to begin with but is absolutely impossible when given a confusing (and wrong) explanation. I own that mistake and sincerely apologize. It likely won't be my last, but I am getting better about first understanding the question and then crafting a coherent response.
var correctGuess = false;
// this do while loop is the same as...
do {
// code
} while (correctGuess === false);
// ...this do while
do {
// code
} while (!correctGuess);
Thanks for catching this and pointing it out.
Chris Shaffer
12,030 PointsChris Shaffer
12,030 PointsOkay actually I figured this out. I used a number of
console.log
statements to log each step of the do/while to see when/where it changed from true to false.Specifically, I did this:
Which made it nice and clear. Seems simple, but I haven't messed w/do/while for a while so I'm a bit rusty.
For anyone caught on this, the simple answer is: The loop basically says run this until
correctGuess
isnot false
. My confusion was reading it as though it said, run thiswhile correctGuess
ISnot false
.