Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial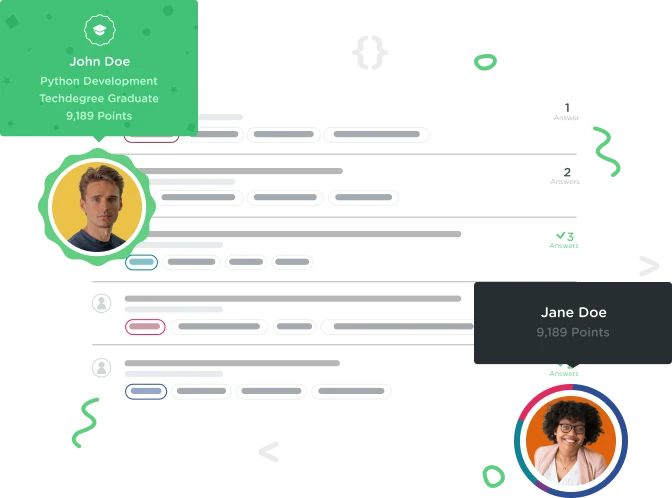

Esther Strom
8,028 PointsDownloaded code doesn't work...
I did the Fun Facts project back when the video was run by the other Ben, so I figured I'd download the code in the teacher's notes for this activity rather than using something that might be out of date.
I've completed the lesson up until the first time we try to run the code. (Added the onSaveInstanceState and onRestoreInstanceState methods.) The app opens and displays the first fact, but when clicking the button to display a new fact, all I get is a blank white screen. There are no errors in the console.
Any ideas?
package com.teamtreehouse.funfacts;
import android.app.Activity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.RelativeLayout;
import android.widget.TextView;
public class FunFactsActivity extends Activity {
private static final String KEY_FACT = "KEY_FACT";
private static final String KEY_COLOR = "KEY_COLOR";
private TextView mFactLabel;
private Button mShowFactButton;
private RelativeLayout mRelativeLayout;
private FactBook mFactBook = new FactBook();
private ColorWheel mColorWheel = new ColorWheel();
private String mFact;
private int mColor;
@Override
protected void onSaveInstanceState(Bundle outState) {
super.onSaveInstanceState(outState);
outState.putString(KEY_FACT, mFact);
outState.putInt(KEY_COLOR, mColor);
}
@Override
protected void onRestoreInstanceState(Bundle savedInstanceState) {
super.onRestoreInstanceState(savedInstanceState);
mFact = savedInstanceState.getString(KEY_FACT);
mFactLabel.setText(mFact);
mColor = savedInstanceState.getInt(KEY_COLOR);
mRelativeLayout.setBackgroundColor(mColor);
mShowFactButton.setTextColor(mColor);
}
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_fun_facts);
// Declare our View variables and assign them the Views from the layout file
mFactLabel = (TextView) findViewById(R.id.factTextView);
mShowFactButton = (Button) findViewById(R.id.showFactButton);
mRelativeLayout = (RelativeLayout) findViewById(R.id.relativeLayout);
View.OnClickListener listener = new View.OnClickListener() {
@Override
public void onClick(View v) {
mFact = mFactBook.getFact();
mFactLabel.setText(mFact);
int color = mColorWheel.getColor();
mRelativeLayout.setBackgroundColor(mColor);
mShowFactButton.setTextColor(mColor);
}
};
mShowFactButton.setOnClickListener(listener);
}
}
2 Answers

christopherkaczenski
16,079 PointsIn the onClick() method of the OnClickListener, the member field mColor is not being used. Change the local variable int color to the member field mColor.

christopherkaczenski
16,079 PointsYou are welcome. Don't worry about not noticing the tiny error with your naked eye, its actually not that important to be able to do that. It is more important to focus on narrowing down where to look for the error, and use the debugger.
For example, with this problem you should be thinking like this: "Okay I know there is an error when I click the button, so let me place a breakpoint at the first line of onClick() method. (tracing through the code line by line) Okay everything seems to be working... wait the argument for the .setBackgroundColor() method is null, why is that?"
By the way, when you ask question on forums like Treehouse or Stackoverflow you should select the best answer so everyone can see the question has been answered.
Esther Strom
8,028 PointsEsther Strom
8,028 PointsThank you! I don't know if I ever would have noticed that.