Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial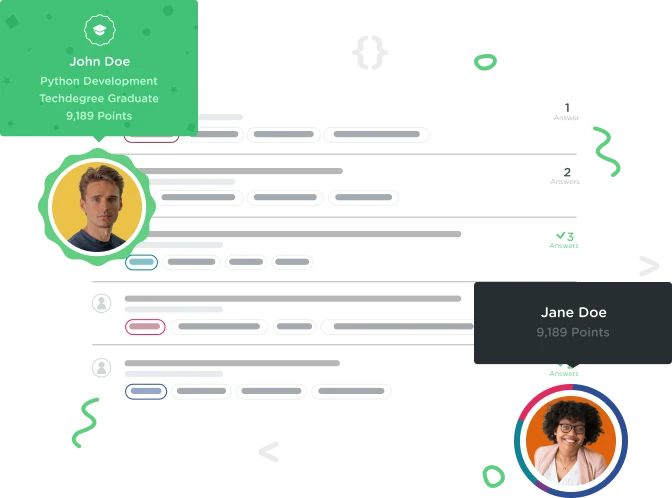
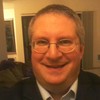
Jeff Mauro
5,374 PointsDrawing application
I can't seem to get my drawing application to work. I have been trying to debug for a couple of hours. The console says I have an error on line 65 below. I will paste my app.js and HTML also.
Line 65:
}).mouseLeave(function(){
app.js:
//Problem: No user interaction causes no change to application
//Solution: When user interacts cause changes appropriately
var color = $(".selected").css("background-color");
var $canvas = $("canvas");
var context = $canvas[0].getContext("2d");
var lastEvent;
var mouseDown = false;
//When clicking on control list items
$(".controls").on("click", "li", function(){
//Deselect sibling elements
$(this).siblings().removeClass("selected");
//Select clicked element
$(this).addClass("selected");
//cache current color
color = $(this).css("background-color");
});
//When "New Color" is pressed
$("#revealColorSelect").click(function(){
//Show color select or hide the color select
changeColor();
$("#colorSelect").toggle();
});
//update the new color span
function changeColor() {
var r = $("#red").val();
var g = $("#green").val();
var b = $("#blue").val();
$("#newColor").css("background-color", "rgb(" + r + "," + g +", " + b + ")");
}
//When color sliders change
$("input[type=range]").change(changeColor);
//When "Add Color" is pressed
$("#addNewColor").click(function(){
//Append the color to the controls ul
var $newColor = $("<li></li>");
$newColor.css("background-color", $("#newColor").css("background-color"));
$(".controls ul").append($newColor);
//Select the new color
$newColor.click();
});
//On mouse events on the canvas
$canvas.mousedown(function(e){
lastEvent = e;
mouseDown = true;
}).mousemove(function(e){
//Draw lines
if(mouseDown) {
context.beginPath();
context.moveTo(lastEvent.offsetx, lastEvent.offsety);
context.lineTo(e.offsetx, e.offsety);
context.strokeStyle = color;
context.stroke();
lastEvent = e;
}
}).mouseup(function(){
mouseDown = false;
}).mouseLeave(function(){
$canvas.mouseup();
});
<!DOCTYPE html>
<html>
<head>
<title>Simple Drawing Application</title>
<link rel="stylesheet" href="css/style.css" type="text/css" media="screen" title="no title" charset="utf-8">
</head>
<body>
<canvas width="600" height="400"></canvas>
<div class="controls">
<ul>
<li class="red selected"></li>
<li class="blue"></li>
<li class="yellow"></li>
</ul>
<button id="revealColorSelect">New Color</button>
<div id="colorSelect">
<span id="newColor"></span>
<div class="sliders">
<p>
<label for="red">Red</label>
<input id="red" name="red" type="range" min=0 max=255 value=0>
</p>
<p>
<label for="green">Green</label>
<input id="green" name="green" type="range" min=0 max=255 value=0>
</p>
<p>
<label for="blue">Blue</label>
<input id="blue" name="blue" type="range" min=0 max=255 value=0>
</p>
</div>
<div>
<button id="addNewColor">Add Color</button>
</div>
</div>
</div>
<script src="http://code.jquery.com/jquery-1.11.0.min.js" type="text/javascript" charset="utf-8"></script>
<script src="js/app.js" type="text/javascript" charset="utf-8"></script>
</body>
</html>
2 Answers
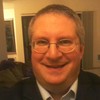
Jeff Mauro
5,374 PointsIt was the capitalization that was making the difference. I changed the x's and y's below to X's and Y's as you did and it enabled the drawing application. Thanks for steering me in the right direction.
``` context.moveTo(lastEvent.offsetX, lastEvent.offsetY); context.lineTo(e.offsetX, e.offsetY);

Keri Nicole
15,134 PointsNow this is making me crazy. I compared your code and my code from the project and they appear to be identical, with the exception of some capitalization here and there. When I try to use the drawing app with your code, it doesn't work. With mine, it does. But they look the same!
I copied my working app.js below. Here's a link to a side by side comparison. Your code is on the left, mine is on the right. It looks like there's more differences due to capitalization and spacing variations, but they look the same otherwise. I can't figure this out!
// Problem: No user interaction causes no change to application.
// Solution: When user interacts, cause changes appropriately.
var color = $(".selected").css("background-color");
var $canvas = $("canvas");
var context = $canvas[0].getContext("2d");
var lastEvent;
var mouseDown = false;
// When clicking on control list items
$(".controls").on("click", "li", function() {
// Deselect sibling elements
$(this).siblings().removeClass("selected");
// Select clicked element
$(this).addClass("selected");
// Cache current color
color = $(this).css("background-color");
});
// When "New Color" is clicked
$("#revealColorSelect").click(function(){
// Show color select or hide the color select
changeColor();
$("#colorSelect").toggle();
});
// Update the new color span
function changeColor() {
var r = $("#red").val();
var g = $("#green").val();
var b = $("#blue").val();
$("#newColor").css("background-color", "rgb(" + r + "," + g + "," + b + ")");
}
// When color sliders change
$("input[type=range]").change(changeColor);
// When "Add Color" is pressed
$("#addNewColor").click(function(){
// Append the color to the control list
var $newColor = $("<li></li>");
$newColor.css("background-color", $("#newColor").css("background-color"));
$(".controls ul").append($newColor);
// Select the new color
$newColor.click();
});
// On mouse event on the canvas
$canvas.mousedown(function(e){
lastEvent = e;
mouseDown = true;
}).mousemove(function(e){
// Draw lines
if(mouseDown) {
context.beginPath();
context.moveTo(lastEvent.offsetX, lastEvent.offsetY);
context.lineTo(e.offsetX, e.offsetY);
context.strokeStyle = color;
context.stroke();
lastEvent = e;
}
}).mouseup(function(){
mouseDown = false;
}).mouseleave(function(){
$canvas.mouseup();
});

Keri Nicole
15,134 PointsAs a side note, my app.js code works with your html and the css from the project files. So I'm pretty sure this is something in the JS, but really, I have no idea what it could be.
Keri Nicole
15,134 PointsKeri Nicole
15,134 PointsGlad you got it figured out! I tried changing the capitalization on }).mouseLeave(function(){ to see if maybe that was it, but it worked either way. Good to know that the offset is case-sensitive.