Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial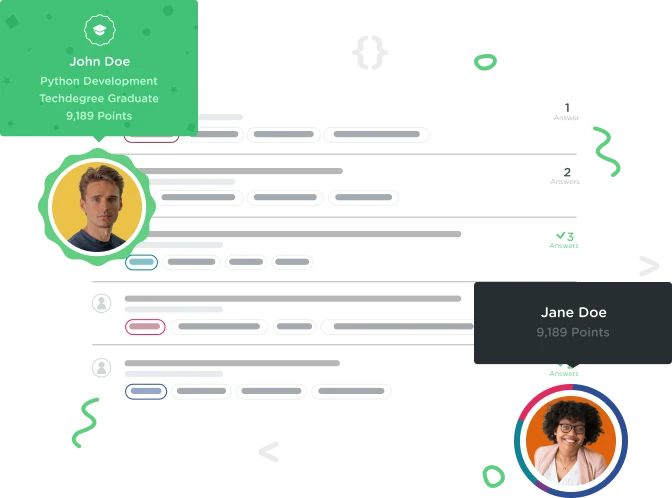
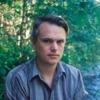
Sean Pierce Sumler
18,968 PointsDrawing rectangles on canvas (fillRect) for HTML5 game workshop
Hey everyone. I'm following along with the "Coding your first HTML5 game" workshop and I'm stuck at a section where I'm supposed to draw rectangles on the canvas. My code looks the same and the instructor's, I'm not sure what I'm doing differently. I've included my code, the part where I'm drawing the rectangle is towards the bottom. Any help is greatly appreciated. Thanks everyone! -Sean
So far- html
<!DOCTYPE html>
<html>
<head>
<title>Game</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<canvas id="canvas" width="400" height="400"></canvas>
<script src="game.js"></script>
</body>
</html>
css
body {
background: #333;
}
canvas {
display: block;
background: #fff;
margin: 0 auto;
}
and script
var canvas = document.getElementById("canvas");
var context = canvas.getContext("2d");
imgHat = new Image();
imgHat.source = "img/frog.png";
imgHat.addEventListener("load",init, false);
var requestAnimFrame =
window.requestAnimationFrame ||
window.webkitRequestAnimationFrame ||
window.mozRequestAnimationFrame ||
window.oRequestAnimationFrame ||
window.msRequestAnimationFrame ||
function (callback) {
window.setTimeout(callback, 1000/60);
};
function init() {
requestAnimFrame(update);
}
function update() {
context.fillRect( 10, 10, 40, 380, "#000000");
// ^ the code in question
requestAnimFrame(update);
}
3 Answers
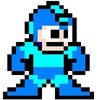
Robert Richey
Courses Plus Student 16,352 PointsUpdated answer below, as a comment
Disclaimer: I have not gone through this workshop.
The init function is what starts the animation loop. Call init()
and it will work.
On an unrelated note, requestAnimationFrame()
is supported in modern browsers, and I'd argue there's no need for a polyfill, if you'd like to reduce your code a bit.
Here's the code I tested with:
var canvas = document.getElementById("canvas");
var context = canvas.getContext("2d");
//imgHat = new Image();
//imgHat.source = "img/frog.png";
//imgHat.addEventListener("load",init, false);
function init() {
requestAnimationFrame(update);
}
function update() {
context.fillRect( 10, 10, 40, 380, "#000000");
requestAnimationFrame(update);
}
init()
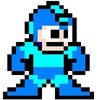
Robert Richey
Courses Plus Student 16,352 PointsAfter reading the lines of code I had commented out - I see that the image load event is supposed to call init. I'll test again using my own image, with your code.
Update: created a local project and found that imgHat.source
should be changed to imgHat.src
, then your code will work.
Cheers!
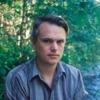
Sean Pierce Sumler
18,968 PointsIt was the "source" vs "src" mistake that wasn't allowing the rest of the code to execute. Weird that I wasn't receiving any errors in the chrome dev console though. Also thanks for the heads up about thee ployfill. Thanks for your help!
Hopefully this solves Anne Kotecha's issue al well.

annekotecha
4,364 PointsThanks Robert Richey and Sean Sumler. Helps to have other people looking at the code.
I did actually have 'src' rather than 'source'. And I did think the 'init' should be called once load was complete. However, I didn't think to look in the chrome dev console! Which showed me that I hadn't written the correct path to the mikethefrog image. So problem solved.

annekotecha
4,364 PointsThanks Robert Richey and Sean Sumler. Helps to have other people looking at the code.
I did actually have 'src' rather than 'source'. And I did think the 'init' should be called once load was complete. However, I didn't think to look in the chrome dev console! Which showed me that I hadn't written the correct path to the mikethefrog image. So problem solved.
annekotecha
4,364 Pointsannekotecha
4,364 PointsI have exactly the same issue and am stuck at the same point. Would love to know the solution!