Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial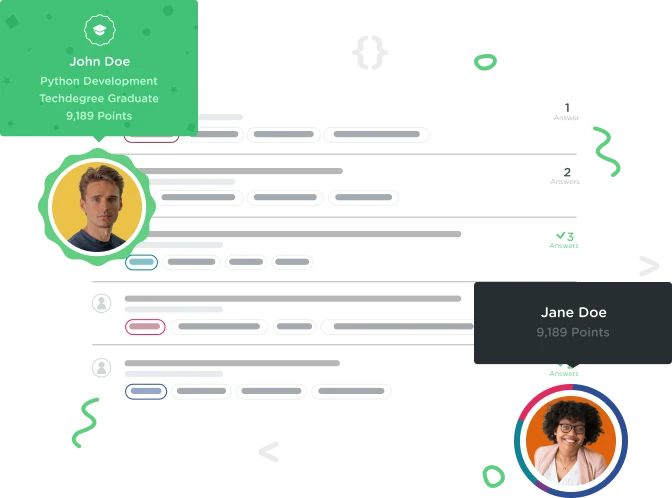

Andrew Kircher
16,612 Pointsdraw_map function seems to have redundant code
The draw_map function in the video looks like this:
def draw_map(player):
print(" _ "*5)
grid ="|{}"
for cell in CELLS:
x, y = cell
if x<4:
line_end= ""
if cell == player:
output =grid.format("X")
else:
output = grid.format("_")
else:
line_end= "\n"
if cell == player:
output =grid.format("X|")
else:
output = grid.format("_|")
print(output, end=line_end)
It seems that the last if statement would over ride the output variable. When I changed the function to:
def draw_map(player):
print(" _ "*5)
grid ="|{}"
for cell in CELLS:
x, y = cell
if x<4:
line_end= ""
else:
line_end= "\n"
if cell == player:
output =grid.format("X|")
else:
output = grid.format("_|")
print(output, end=line_end)
It seemed to produce the same results. The code can even be reduced to
def draw_map(player):
print(" _ "*5)
grid ="|{}"
for cell in CELLS:
x, y = cell
if x<4:
line_end= ""
if cell == player:
output =grid.format("X|")
else:
output = grid.format("_|")
else:
line_end= "\n"
print(output, end=line_end)
And produce the same results. So is there a reason we added all of this extra code?
1 Answer
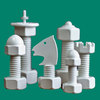
Steven Parker
231,269 PointsDid you try running with these changes? It doesn't produce the same output as the original.
But even if it did, remember that the objective of the course is for you to learn the techniques, not necessarily build the most efficient sample program. There are cases where a little extra verbosity helps make the teaching point much more clearly.
As you progress in the courses and the sample programs get more complex, there will often be opportunities to make the code more compact and/or efficient. The fact that you notice these is a good indication of your learning progress!
And it is possible to make this function much more compact and still work the same, here's an example:
def draw_map(p):
print(" _"*5)
for c in CELLS:
print("|X" if c==p else "|_", end="" if c[0]<4 else "|\n")
But this might not be as easy to understand for most students of a "Beginner" level course.
Nader Alharbi
2,253 PointsNader Alharbi
2,253 PointsYou Sir ... you keep impressing me every time i read one of your comments! Keep up the good work, your code is much easier to read and understand than the long one. I didn't even know that i could include my if conditions in the print function !