Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial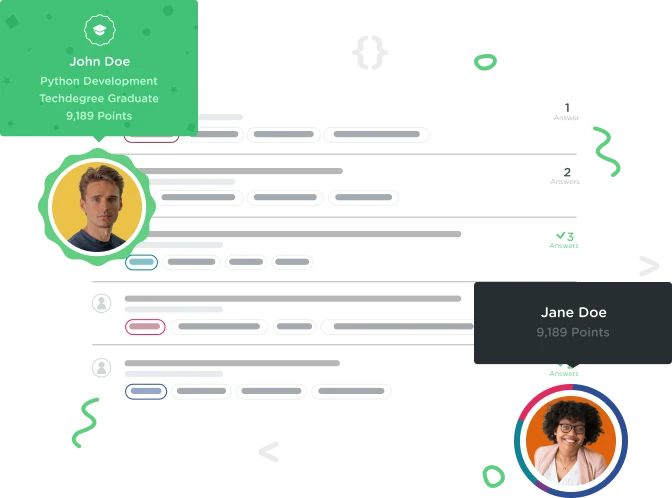

Chaz Hall
1,970 PointsDrive Arguments and the new variable in the argument- start at 0 or 1?
(int mBarsCount= 8 for this question)
public void drive() { drive(1); }
public void drive(int laps) { // Other driving code omitted for clarity purposes if (laps <= mBarsCount) { mBarsCount -= laps; } else { throw new IllegalArgumentException(); } }
In a previous question I finally understood that methods can have the same name as long as the argument is different. The method drive() seems to increase drive by 1 each time it is run.
The next method drive(int laps) seems to create an Integer that starts at 0. If in fact it starts at 0 then the if loop would always be stuck at 8 because of [mBarsCount=mBarsCount(8)-laps(0) and mBarsCount stays at 8.
How do we get the drive(int laps) to at least become 1 so that mBarsCount can decrease?
I notice the method drive() states drive(1). Does that means that the second method drive(int laps) makes the (int laps) variable 1 to begin with? If so, please explain the logic. Thank you all.
public class GoKart {
public static final int MAX_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public void drive() {
drive(1);
}
public void drive(int laps) {
// Other driving code omitted for clarity purposes
mBarsCount -= laps;
}
public void charge() {
while (!isFullyCharged()) {
mBarsCount++;
}
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
return mBarsCount == MAX_BARS;
}
}
2 Answers
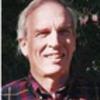
jcorum
71,830 Points1) The drive() method returns no variable, //that's correct - it's return type is void
but declares //no, methods don't declare anything. You declare variables. E.g., int laps; String mColor; etc.
that any method with the overloaded name drive(int laps) or things like drive(int wedgies), drive (int funnyPants) would all contain 1 as the integer. //No, it calls the other drive() method with the value 1. The other drive() method has a formal parameter laps, and so, to call it, you have to pass an int value. drive() is calling it with a value of 1.
Does the method drive() not change because it is not a variable? // drive() doesn't change, but it has nothing to do with not being a variable. methods are not variables! They don't hold values. Classes have 3 major parts: fields (variables, constants), constructors, and methods. Fields store values. Constructors create objects. Methods give objects behavior -- they do things. drive() calls the drive(int laps) method with a value of 1, and drive(int laps) will take that value and decrement the value of mBarsCount (a variable). drive() is what some call a convenience method. It's not really necessary, as you could always call the other drive() method with a value of 1: drive(1), just like you could call it with a value of 2: drive(2).
2)If the drive() method didn't declare drive(1) //No, methods don't declare anything
then would drive(int laps) be drive(0)? //as just noted above, writing drive() in code would has the same result as writing drive(1), because the drive() method calls the drive(int laps) method with a value of 1, which is the same as calling the drive(int laps) method with a value of 1.
3) When a method has no arguments, does that means that each method with the same name, but different arguments looks to it as the "parent" or the template in which it uses to define themselves? // No, there is no child/parent relationship. Each has to "define" itself. But one method can call another. The reason drive() calls drive(int laps) is the DRY principle. You don't want to repeat the code that is in drive(int laps) in drive().
Can the method of the same name, but a argument, ever affect the method with no arguments? //No, on reasonable interpretations of "affect".
Hope this helps.
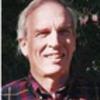
jcorum
71,830 PointsInteresting. The editor accepts your code, even though you didn't specify the error message it requested!!
"In a previous question I finally understood that methods can have the same name as long as the argument is different. The method drive() seems to increase drive by 1 each time it is run."
Yes, the term, if you haven't heard it, is overload. A method overloads another if the name is the same and the method signature is different (the type and number of parameters).
public void drive() {
drive(1);
}
public void drive(int laps) {
// Other driving code omitted for clarity purposes
mBarsCount -= laps;
}
Here drive() overloads drive(int laps). If it is called it calls the drive(int laps) method and passes it a value of 1. drive(int laps) takes that value, stores it in a local variable named laps, and then uses it to decrement mBarsCount. It does not "increase drive by 1 each time it is run". There is no drive variable to increase or decrease.
"The next method drive(int laps) seems to create an Integer that starts at 0. If in fact it starts at 0 then the if loop would always be stuck at 8 because of [mBarsCount=mBarsCount(8)-laps(0) and mBarsCount stays at 8."
No. First, there is no loop. But each time the method is called it decrements mBarsCount by the value of laps. The challenge was to make sure mBarsCount doesn't become negative. You can't have mBarsCount(8) because mBarsCount is not a method. It is a member (or instance) variable that holds an int value. It does not stay at 8 -- it is decremented each time the drive() or drive(int laps) method is called. If drive(int laps) is called this way: drive(8) then the method would decrease mBarsCount by 8. If it were called this way: drive(2) it would decrement mBarsCount by 2. Etc.

Chaz Hall
1,970 PointsI believe my "stuck at 8 because..." sentence used symbols and such like mBarsCount(8) that it became unclear what I was asking, which I apologize for.
For some reason the posted code wasn't my completed code, which is why it seems it was accepted, because it wasn't completed.
Of course, these answers beg similar questions.
1) The drive() method returns no variable, but declares that any method with the overloaded name drive(int laps) or things like drive(int wedgies), drive (int funnyPants) would all contain 1 as the integer. Does the method drive() not change because it is not a variable? 2)If the drive() method didn't declare drive(1) then would drive(int laps) be drive(0)? 3) When a method has no arguments, does that means that each method with the same name, but different arguments looks to it as the "parent" or the template in which it uses to define themselves? Can the method of the same name, but a argument, ever affect the method with no arguments?
Thanks you for your help!