Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial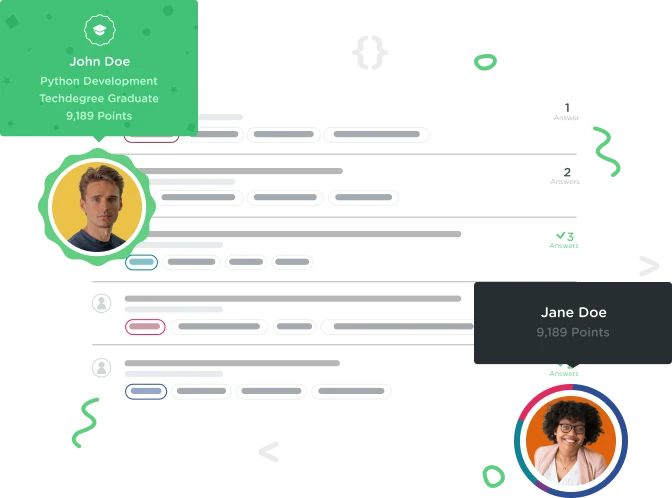

saro lin
Courses Plus Student 531 PointsDRY
I really don't get the concept of DRY.. Can anybody please more specific?
1 Answer
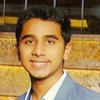
Anish Walawalkar
8,534 PointsDRY - DON'T REPEAT YOURSELF
Im going to explain this concept with an example
Suppose you have a list of numbers:
number_list = list(range(1,100)) #generating a list of numbers from 1 to 99
You need to obtain the 2nd, 3nd, 4th power of the numbers in number_list list and print the results or stores the result in another list. You can approach this problem in 2 different ways:
The first way involves writing separate functions to calculate the respective powers as such:
def power2(number_list):
new_number_list = []
for num in number_list:
new_number_list.append(num**2)
return new_number_list
def power3(num):
new_number_list = []
for num in number_list:
new_number_list.append(num**3)
return new_number_list
def power4(num):
new_number_list = []
for num in number_list:
new_number_list.append(num**4)
return new_number_list
number_list = list(range(1, 100))
print(power2(number_list))
print(power3(number_list))
print(power4(number_list))
As you can clearly see, this isn't a very efficient way of coding, the function power2, power3 and power4 have the exact same lines of code and perform essentially the same operation (REPETITION)
A better way to solve this problem would be write a single function that takes 2 parameters:
- the original list
- the power you want to raise the the numbers in the list to
so in code it looks like this:
def power(number_list, pow):
new_number_list = []
for num in number_list:
new_number_list.append(num**pow)
return new_number_list
number_list = list(range(1, 100))
print(power(number_list, 2))
print(power(number_list, 3))
print(power(number_list, 4))
This solves 2 problems:
- You are not repeating code any more. your code is DRY
- Your function is now generic, it can now find the nth power of a number
saro lin
Courses Plus Student 531 Pointssaro lin
Courses Plus Student 531 PointsThank you so much. Now gotcha :)