Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial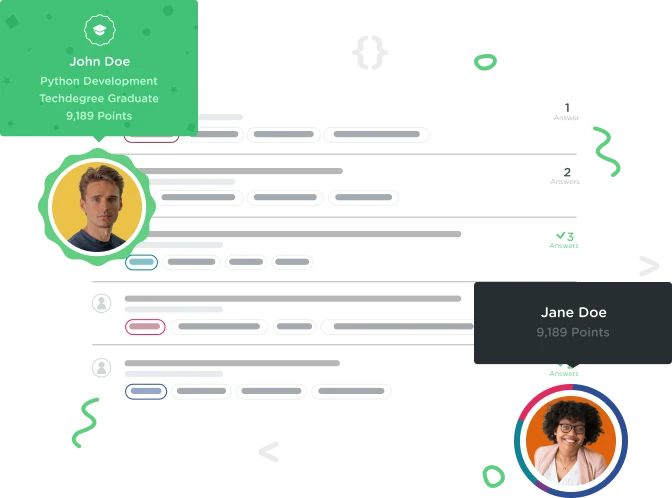

nuralysargaskayev
Courses Plus Student 2,202 PointsDRY | console
I try all my ideas my they didn't work. I still have an error with: Traceback (most recent call last): File "<stdin>", line 1, in <module> AttributeError: 'Goblin' object has no attribute 'attack'
import random
from combat import Combat
COLORS = ['yellow', 'red', 'blue', 'green']
class Monster(Combat):
min_hit_points = 1
max_hit_points = 1
min_experience = 1
max_experience = 1
weapon = 'sword'
sound = 'roar'
def __init__(self, **kwargs):
self.hit_points = random.randint(self.min_hit_points, self.max_hit_points)
self.experience = random.randint(self.min_experience, self.max_experience)
self.color = random.choice(COLORS)
for key, value in kwargs.items():
setattr(self, key, value)
def __str__(self):
return '{} {}, HP: {}, XP: {}'.format(self.color.title(),
self.__class__.__name__,
self.hit_points,
self.experience)
def battlecry(self):
return self.sound.upper()
class Goblin(Monster):
max_hit_points = 3
max_experience = 2
sound = 'squeak'
class Troll(Monster):
min_hit_points = 3
max_hit_points = 5
min_experience = 2
max_experience = 6
sound = 'growl'
class Dragon(Monster):
min_hit_points = 5
max_hit_points = 10
min_experience = 6
max_experience = 10
sound = 'raaaaaaaaaar'
from combat import Combat
class Character(Combat):
experience = 0
hit_points = 10
def get_weapon(self):
weapon_choice = input("Weapon ([S]word, [A]xe, [B]ow): ").lower()
if weapon_choice in 'sab':
if weapon_choice == 's':
return 'sword'
elif weapon_choice == 'a':
return 'axe'
else:
return 'bow'
else:
self.get_weapon()
def __init__(self, **kwargs):
self.name = input("Name: ")
self.weapon = self.get_weapon()
for key, value in kwargs.items():
setattr(self, key, value)
import random
class Combat:
dodge_limit = 6
attack_limit = 6
def dodge (self):
roll = random.randint(1, self.dodge_limit)
return roll > 4
def attack(self):
roll = random.randint(1, self.attack_limit)
return roll > 4
1 Answer
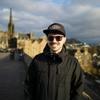
mattamorphic
Courses Plus Student 6,641 PointsHi There :)
Is the indenting correct for the methods in your combat class? In the example you've given it looks like the methods are at the same level as class declaration and not indented 4 spaces
class Combat:
dodge_limit = 6
attack_limit = 6
def dodge (self):
roll = random.randint(1, self.dodge_limit)
return roll > 4
def attack(self):
roll = random.randint(1, self.attack_limit)
return roll > 4
It might not be the case - but worth having a look :)
Iain Simmons
Treehouse Moderator 32,305 PointsIain Simmons
Treehouse Moderator 32,305 PointsI think this would definitely be the case. Without the indentation, the functions will just be part of the main script.
Could probably test by passing the instance of the Goblin class into the function to see what happens... or try
dir(dodge)