Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial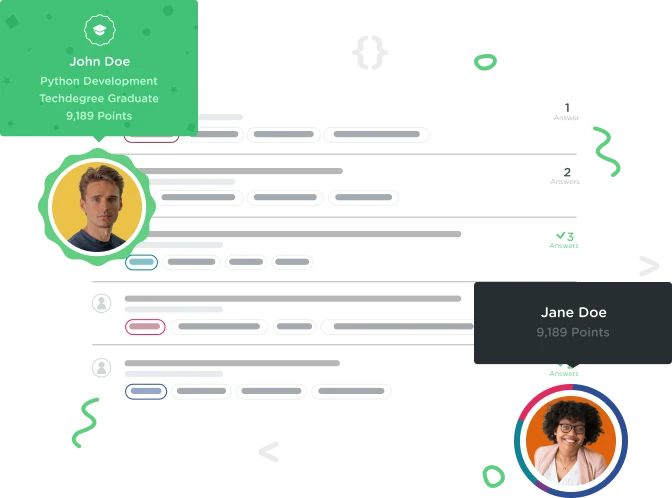
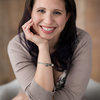
Michelle Harrison
8,782 PointsDRY Programming
I applied DRY Programming to the exercise:
var shopping = [ 'carrots', 'milk', 'eggs'];
for(var i = 0; i < shopping.length; i += 1) {
console.log(shopping[i]);
}
I tried to do document.write instead of console.log, but every item comes out as "undefined"
var shopping = [ 'carrots', 'milk', 'eggs'];
var y = ' ' + shopping[i] + ','
for(var i = 0; i < shopping.length; i += 1) {
document.write(y);
}
I assume this is something we'll learn in a later video, but I've found that I absorb things more when I expand on the given exercises.
2 Answers

Frederick Carle
7,054 PointsHi Michelle! If you want to not have to worry about the last comma, you can use the join method on the shopping array like so.
var shopping = [ 'carrots', 'milk', 'eggs'];
var str = shopping.join(", ");
document.write(str);
No need for a for loop in this case.

Edwin Carbajal
4,133 PointsHi Michelle,
Fredericks answer is correct and probably easier, but if you were trying to write out the loop, this is how it would work:
var shopping = [ 'carrots', 'milk', 'eggs' ];
var y = '';
for(var i=0; i < shopping.length; i++) {
y += " " + shopping[i];
}
document.write(y);
Michelle Harrison
8,782 PointsMichelle Harrison
8,782 PointsOooh! I got it! (Well, sort of. Doing it this way will leave a comma at the end of the array.) I just had to remember about local and global scope.