Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial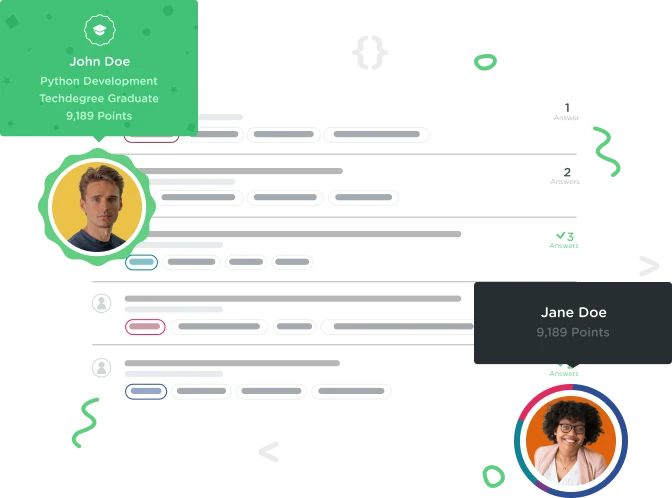

Javier Mendoza Dominguez
1,986 Pointsdungeon game
import os
import random
def grid(num):
list_num = list(range(num))
list_grid = []
y = 0
while y < num:
for x in list_num:
list_grid.append((x, y))
y += 1
return list_grid
def clear_screen():
os.system('cls' if os.name == 'nt' else 'clear')
def get_locations(num):
return random.sample(CELLS, num)
def move_player(player, move):
x, y = player
if move == "LEFT":
x -= 1
if move == "RIGHT":
x += 1
if move == "UP":
y -= 1
if move == "DOWN":
y += 1
return x, y
def make_monsters(num):
list_monsters = []
for num in list(range(num)):
list_monsters.append("monster{}".format(num))
return tuple(list_monsters)
def move_monsters(monsters):
list_moves = []
for monster in monsters:
moves = get_moves(monster)
move = random.choice(moves)
x, y = monster
if move == "LEFT":
x -= 1
if move == "RIGHT":
x += 1
if move == "UP":
y -= 1
if move == "DOWN":
y += 1
list_moves.append((x,y))
return tuple(list_moves)
def get_moves(player):
moves = ["LEFT", "RIGHT", "UP", "DOWN"]
x, y = player
limite = tam_grid - 1
if x == 0:
moves.remove("LEFT")
if x == limite:
moves.remove("RIGHT")
if y == 0:
moves.remove("UP")
if y == limite:
moves.remove("DOWN")
return moves
def draw_map(draws):
print(" _"*tam_grid)
tile = "|{}"
monsters, door, player, movs_history = draws
list_monsters = []
list_movs = []
limite = tam_grid -1
for monster_value in monsters:
list_monsters.append(monster_value)
for value in movs_history.values():
for tv in value:
list_movs.append(tv)
for cell in CELLS:
x, y = cell
if x < limite:
line_end = ""
if cell in list_movs:
output = tile.format(chr(177))
elif cell in list_monsters:
output = tile.format("&")
elif cell == door:
output = tile.format("]")
elif cell == player:
output = tile.format(chr(169))
else:
output = tile.format("_")
else:
line_end = "\n"
if cell in list_movs:
output = tile.format("*|")
elif cell in list_monsters:
output = tile.format("&|")
elif cell == door:
output = tile.format("]|")
elif cell == player:
output = tile.format(chr(169))
else:
output = tile.format("_|")
print(output, end=line_end)
def record_player(record):
list_records = []
for dic in record:
list_records.append("{} | Total de movimientos en la partida: {}".format(dic["partida"], dic["movimientos"]))
print("_ " * 30)
print("\n*** Lista de partidad jugadas ***")
print("_ " * 30)
for index, item in enumerate(list_records, start = 1):
print("\nPartida {}: {}".format(index, item))
print("_ " * 30)
input("\n *** !presiona enter para regresar al juego! *** ")
def game_loop():
monsters = make_monsters(numero_monsters)
monsters = get_locations(numero_monsters)
movs_history = {"player": []}
door, player = get_locations(numero_players + numero_doors)
playing = True
while playing:
clear_screen()
draws_map = monsters, door, player, movs_history
draw_map(draws_map)
valid_moves = get_moves(player)
print("Actualmente estas en la sala {}".format(player))
print("Puedes moverte {}".format(", ".join(valid_moves)))
print("QUIT para salir")
print("RECORD paver ver detalles de partidas jugadas")
move = input("> ")
move = move.upper()
if move == "QUIT":
print("\n *** Hasta la proxima *** \n")
break
if move == "RECORD":
record_player(record)
continue
if move in valid_moves:
monsters = move_monsters(monsters)
movs_history["player"].append(player)
player = move_player(player, move)
if player in monsters:
print("\n *** !Has perdido el moustro te tiene¡. Suerte para la proxima *** \n")
record.append({"partida": "Perdida", "movimientos": len(movs_history["player"])})
playing = False
if player == door:
print("\n ***! Felicidades has logrado escapar *** \n")
record.append({"partida": "Ganada", "movimientos": len(movs_history["player"])})
playing = False
else:
input("\n ** !la pered es dura evitala¡*** \n")
else:
if input("¿Jugar otra vez? [s/n] >").lower() != "n":
game_loop()
clear_screen()
print("Bienvenido a Dungeon!")
input("presiona Enter para iniciar el juego")
clear_screen()
tam_grid = 15
numero_monsters = 20
numero_players = 1
numero_doors = 1
CELLS = grid(tam_grid)
record = []
game_loop()
[MOD: added ```python formatting -cf]