Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial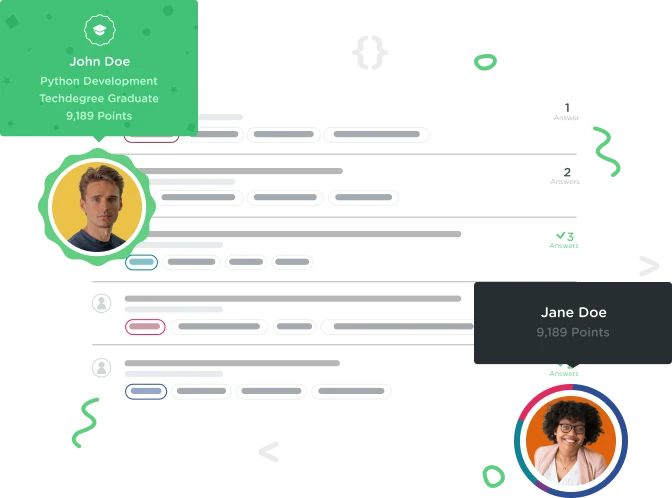

Jason Portilla
15,345 PointsDungeon game and letter guess....
Can someone explain how the code works using the draw map function using the tiles and underscore part and having the X move around. He did the same thing with the letter game if you guessed the letter it showed up part of the word...
_ _ _ _ _ _ a _
It's in a for loop but I'm still confused how it works.
4 Answers
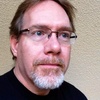
Chris Freeman
Treehouse Moderator 68,460 PointsMany times printing text to the screen is straightforward using a single print
statement, perhaps using the format()
method. Other times, it needs to constructed character by character with adding end-of-line ("carriage returns") explicitly using loops. In these cases, I think of an old style typewriter.
To print a single character to the screen and stay on the same line, print
has an optional argument end
that specifies what should be printed at the end of this output. The default is an end-of-line ("carriage return"). By specifying end=''
(empty string), or end=' '
(space), the cursor will stay on the same line waiting for the next character(s).
The letter game, uses this method to print out the current progress:
# loop over every letter in secret_word
for letter in secret_word:
# check if this letter has been guessed
if letter in good_guesses:
# if letter guessed print letter character with no EOL
print(letter, end='')
else:
# if not guessed print placeholder character '_' with no EOL
print('_', end='')
# after looping over secret_word characters
# print a blank character, *with* EOL to end the line
print('')
In the Dungeon game, the same technique is used, but instead of looping over characters in a word, it loops over grid coordinates in a list. In this code, row_end
is the length of a row, so index % row_end
< row_end - 1
is a check to see if this index is the last character(s) for this row:
# for each cell in cell list, get the current index and cell contents
for index, cell in enumerate(cells):
# check if this is last cell for this row
if index % row_end < row_end - 1:
# not last cell: use end='' to avoid EOL character
# check which character to print: 'X', '.', or '_'
if cell == player['location']:
print(tile.format('X'), end='')
elif cell in player['path']:
print(tile.format('.'), end='')
else:
print(tile.format('_'), end='')
else:
# IS last cell: allow EOL character
# check which character to print: 'X', '.', or '_'
if cell == player['location']:
print(tile.format('X|'))
elif cell in player['path']:
print(tile.format('.|'))
else:
print(tile.format('_|'))
This use of a formatted tile
string allows the output to be customized in one location without having to changing many lines of code. If you wanted a wider grid output, simply change the tile
value:
tile = '| {} ' # added space around cell contents
The dungeon code could also have been written without the tile
:
for index, cell in enumerate(cells):
if index % row_end < row_end - 1:
if cell == player['location']:
print('|X', end='')
elif cell in player['path']:
print('|.', end='')
else:
print('|_', end='')
else:
if cell == player['location']:
print('|X|')
elif cell in player['path']:
print('|.|')
else:
print('|_|')

james white
78,399 PointsYes, please, more info...
If you search the forum for the word "Dungeon" most of the results that come up
are associate with this Python course:
https://teamtreehouse.com/library/python-collections
I would be interested in any effort of develop a Dungeon related game NOT in Python

Jason Portilla
15,345 PointsThank you so much Chris...but why is letter in good_guesses know the letter is in secret word if it starts out as an empty list?
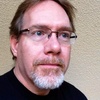
Chris Freeman
Treehouse Moderator 68,460 PointsNot sure I understand your question.
Good_guesses starts off empty. When each letter of secret_word is compared to the empty list you will not be found and an underscore character will be printed.
As letters are added to good_guesses, The matching letter within secret_word will be found in good_guesses it will be printed.

Jason Portilla
15,345 Pointsthank you so much.
Alex Brown
3,555 PointsAlex Brown
3,555 PointsCould you add some more context, preferably code? This is a hard question to understand.