Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial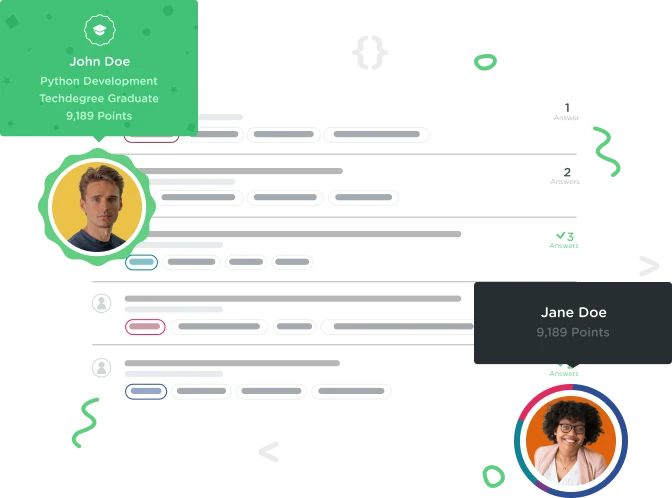

kamal diriye
1,597 PointsDungeon game; draw_map
def draw_map():
print(' _ _ _')
tile = '|{}'
for idx, cell in enumerate(cells):
if idx in [0, 1, 3, 4, 6, 7]:
if cell == player['location']:
print(tile.format('X'), end='')
elif cell in player['path']:
print(tile.format('.'), end='')
else:
print(tile.format('_'), end='')
else:
if cell == player['location']:
print(tile.format('X|'))
elif cell in player['path']:
print(tile.format('.|'))
else:
print(tile.format('_|'))
It's hard to understand this code when the video for it has been retired. Can someone please explain this to me in simple terms?
Thanks
2 Answers
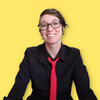
Mel Rumsey
Treehouse ModeratorHey kamal diriye,
It's been quite a while since I completed this course, so I will try and remember what it does.
for idx, cell in enumerate(cells):
if idx in [0, 1, 3, 4, 6, 7]:
This part is going through each cell on the map which looked like a grid if I remember properly. It goes through the index and the cell. If it is 0, 1, 3, 4, 6, or 7 it does these checks:
if cell == player['location']:
print(tile.format('X'), end='')
elif cell in player['path']:
print(tile.format('.'), end='')
else:
print(tile.format('_'), end='')
The player["location"]
was where the player is at on the grid.
The `player["path"] was where the player has been.
This changes what is inside the cell based on these conditions.
else:
if cell == player['location']:
print(tile.format('X|'))
elif cell in player['path']:
print(tile.format('.|'))
else:
print(tile.format('_|'))
This part runs for any other idx
outside of 0, 1, 3, 4, 6, or 7. I believe it was just adding the |
to keep the grid formatted properly.
It's definitely difficult to explain without the rest of the code for this but it's basically just a for loop and conditional checks that update the grid based on the conditions being true.
Sorry I can't be more help on this!

kamal diriye
1,597 Pointsimport random
player = {'location': None, 'path': []}
cells = [(0, 0), (0, 1), (0, 2),
(1, 0), (1, 1), (1, 2),
(2, 0), (2, 1), (2, 2)]
def get_locations():
monster = random.choice(cells)
door = random.choice(cells)
start = random.choice(cells)
if monster == door or monster == start or door == start:
monster, door, start = get_locations()
return monster, door, start
def get_moves(player):
moves = ['LEFT', 'RIGHT', 'UP', 'DOWN']
if player in [(0, 0), (1, 0), (2, 0)]:
moves.remove('LEFT')
if player in [(0, 0), (0, 1), (0, 2)]:
moves.remove('UP')
if player in [(0, 2), (1, 2), (2, 2)]:
moves.remove('RIGHT')
if player in [(2, 0), (2, 1), (2, 2)]:
moves.remove('DOWN')
return moves
def move_player(player, move):
x, y = player['location']
player['path'].append((x, y))
if move == 'LEFT':
player['location'] = x, y - 1
elif move == 'UP':
player['location'] = x - 1, y
elif move == 'RIGHT':
player['location'] = x, y + 1
elif move == 'DOWN':
player['location'] = x + 1, y
return player
def draw_map():
print(' _ _ _')
tile = '|{}'
for idx, cell in enumerate(cells):
if idx in [0, 1, 3, 4, 6, 7]:
if cell == player['location']:
print(tile.format('X'), end='')
elif cell in player['path']:
print(tile.format('.'), end='')
else:
print(tile.format('_'), end='')
else:
if cell == player['location']:
print(tile.format('X|'))
elif cell in player['path']:
print(tile.format('.|'))
else:
print(tile.format('_|'))
monster, door, player['location'] = get_locations()
while True:
moves = get_moves(player['location'])
print("Welcome to the dungeon!")
print("You're currently in room {}".format(player['location']))
draw_map()
print("\nYou can move {}".format(', '.join(moves)))
print("Enter QUIT to quit")
move = input("> ")
move = move.upper()
if move == 'QUIT':
break
if not move in moves:
print("\n** Walls are hard! Stop running into them! **\n")
continue
player = move_player(player, move)
if player['location'] == door:
print("\n** You escaped! **\n")
break
elif player['location'] == monster:
print("\n** You got eaten! **\n")
break
else:
continue
'''
This is the rest of the code if it helps.