Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial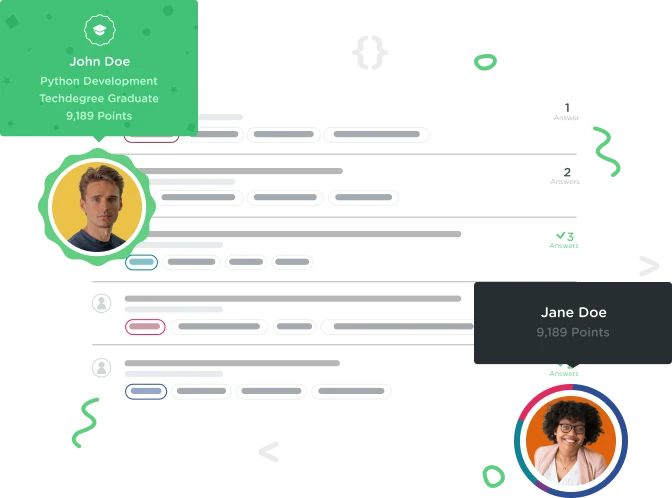

matthias papsdorf
Courses Plus Student 4,152 Pointsdungeon game for review - any tips are welcome
This should hopefully run fine. Any tips are welcome. As for player, I kind of felt the urge of using a player class but I wait till we do classes in the course.
Question about the convention, a function that is only called within a specific function is having an underscore in it's name? I am not quite sure what the standard conventions are.
Adding my game below:
import random
CELLS = [(0, 0), (0, 1), (0, 2),
(1, 0), (1, 1), (1, 2),
(2, 0), (2, 1), (2, 2)]
def get_locations():
available_coords = CELLS[:]
pos = ()
def _do_pos():
pos = available_coords.pop(available_coords.index(random.choice(available_coords)))
return pos
monster = _do_pos()
door = _do_pos()
start = _do_pos()
return monster, door, start
def move_player(player, move):
x = player[0]
y = player[1]
if move == "LEFT":
x -= 1
elif move == "RIGHT":
x += 1
elif move == "UP":
y += 1
elif move == "DOWN":
y -= 1
player = (x, y)
return player
def get_moves(player):
MOVES = ['LEFT', 'RIGHT', 'UP', 'DOWN']
if player[0] == 0:
MOVES.remove("LEFT")
if player[0] == 2:
MOVES.remove("RIGHT")
if player[1] == 0:
MOVES.remove("DOWN")
if player[1] == 2:
MOVES.remove("UP")
return MOVES
monsterpos, doorpos, playerpos = get_locations()
while True:
print("Welcome to the dungeon!")
print("You're currently in room {}".format(playerpos)) # fill in with player position
print("You can move {}".format(get_moves(playerpos))) # fill in with available moves
print("Enter QUIT to quit")
move = input("> ")
move = move.upper()
if move == 'QUIT':
break
if move in get_moves(playerpos):
playerpos = move_player(playerpos, move)
elif move not in get_moves(playerpos):
print("You cannot go there")
if playerpos == doorpos:
print("you have won, yay!")
input("Press any key")
quit()
elif playerpos == monsterpos:
print("ZONK!!! You're dead")
input("Press any key")
quit()
else:
continue
1 Answer
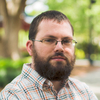
Kenneth Love
Treehouse Guest TeacherFunctions starting with an underscore are typically thought of as being private. Most of the time you'll actually see this applied more to methods in a class than functions in functions or functions in a module.
matthias papsdorf
Courses Plus Student 4,152 Pointsmatthias papsdorf
Courses Plus Student 4,152 PointsThanks for the answer. I will keep that in mind.
Matthias