Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial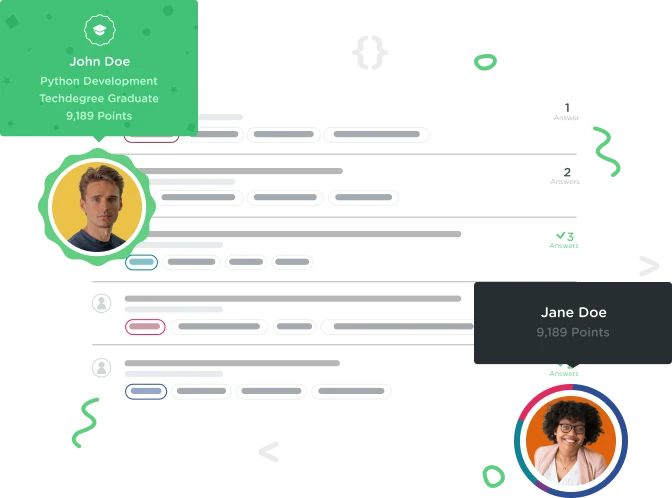
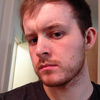
Adam Wieczorek
129 PointsDuplicate input
For some reason, during the shopping list exercise, I'm being asked to input data twice. As you can guess I'm pretty fresh with ruby so anyone can help? ;(
def create_list print "What is the list name? " name = gets.chomp
hash = { "name" => name, "items" => Array.new } return hash end
def add_list_item print "What is the item called? " item_name = gets.chomp
print "How much? " quantity = gets.chomp.to_i
hash = { "name" => item_name, "quantity" => quantity} return hash end
list = create_list() puts list.inspect list['items'].push(add_list_item())
puts add_list_item().inspect
puts list.inspect
1 Answer

Nick Paridon
5,848 PointsHey Adam,
I think its the second to the last line giving you issues. If you get rid of
puts add_list_item().inspect
It shouldn't ask you twice.
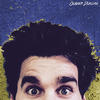
Oliver Duncan
16,642 PointsI would just add that parentheses after a method call with no arguments are unnecessary, and even kind of "non-rubyist",
i.e. list = create_list
is preferable to list = create_list()
.
Oliver Duncan
16,642 PointsOliver Duncan
16,642 PointsFormatting is extremely important for readability. The code above is almost nonsensical. Try formatting it with the markdown cheatsheet, like this.