Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial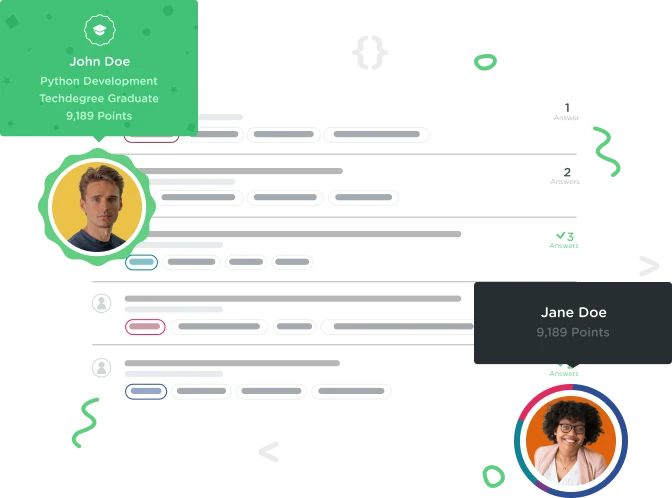

hun ee4
161 PointsDuring Iterating and Rendering with map() ,why and where it comes from ? why typing props.initialPlayers.map
why typing props.initialPlayers.map , not props.Players.map
<Player name="Guil" score={50} />
<Player name="Treasure" score={90} />
<Player name="Ashley" score={85} />
<Player name="James" score={80} />
{props.initialPlayers.map( player =>
<Player
name={player.name}
score={player.score}
/>
)}
2 Answers
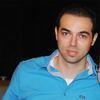
Ezra Siton
12,644 PointsinitialPlayers
is the name of the prop VS Players
- is the value of initialPlayers
(Could be "hello", "55" or "bla bla" or const with an array of objects
).
Take a look at this example (I put list-of-objects directly inside initialPlayers instead of const
):
ReactDOM.render(
<App initialPlayers={[{name: "john"}, {name: "ben"}]} />,
document.getElementById('root')
);
The map() method creates a new array with the results of calling a provided function on every element in the calling array. https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/map
You want to loop throw the array (Otherwise you get this error):
Uncaught TypeError: Cannot read property 'map' of undefined
One more Very simple example:
const soccerPlayers = [
{
name: "Ronaldo",
score: 100
},
{
name: "Messi",
score: 100
},
{
name: "Bale",
score: 90
}
];
const App = (props) => {
return (
<ul>
{/* Players list */}
{props.initialPlayers.map( player =>
<li>{player.name}: {player.score} </li>
)}
</ul>
);
}
ReactDOM.render(
<App initialPlayers={soccerPlayers} />,
document.getElementById('root')
);
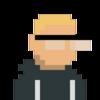
Everton Carneiro
15,994 PointsBecause initialPlayers is the props of the App component.
marcell gibbs
3,744 Pointsmarcell gibbs
3,744 Pointsi am getting that error even while using map