Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial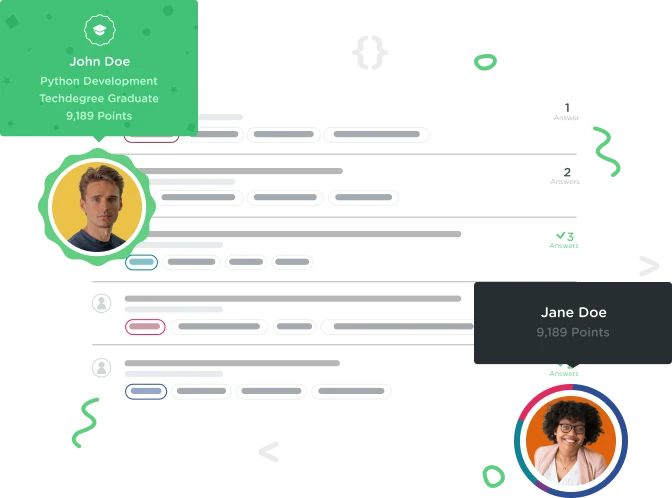

Nour El-din El-helw
8,241 PointsDynamic properties in the constructor method
Why not create a dynamic property in a constructor method with a default value, then create some code to change the property's value based on the current time or something? Is it just all about simplifying stuff?
2 Answers
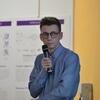
Dima Fedin
7,651 PointsFrom my point of view, there isn't difference what to use. Getter is just new feature of ES6 but you may use the method which was in old version. Getter is just more semantic.
Before ES6:
function Blog() {
this.posts = []
}
Blog.prototype.getPosts = function() {
this.posts = fetch('https://blog.api') // refresh posts via API
}
ES6:
class Blog() {
get posts() {
const posts = fetch('https://blog.api')
return posts
}
}
Mix of ES6 and before ES6:
class Blog() {
constructor() {
this.posts = []
}
getPosts() {
this.posts = fetch('https://blog.api')
}
}
You can use any method but the second example, I'm sure, is good practice. If I'm not right correct me.

Nour El-din El-helw
8,241 PointsThanks!
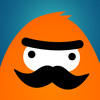
Muhammad Umar
7,817 PointsSo my question is similar to that of OP. This training module explains that the idea behind using getters is to define a property value on an object that will be dynamic or computes a value.
What I am trying to differentiate is, in which scenario getters would be ideal rather than defining a method in the Constructor method and assigning it to a function. When that method is called, it will still run through the function and computers or generate a dynamic value, right?
So in this getter/setter module, I tried the code below and now really trying to understand why I would use get activity(){}
instead of defining the method this.activity
in the constructor method and calling when needed. At this stage, the only difference I see obvious how we make the call. If we use getters we call it like a normal property and if we use a method we will have to call is using the () in the end. Are there other big-picture differences that I am missing?
Another thing that also stood out for me is that getters can be used to define a property that you do not want to show up in every single instance of the object you create from a given Class
Any help would be much appreciated!
class Pet {
constructor(animal, age, breed, sound) {
this.animal = animal;
this.age = age;
this.breed = breed;
this.sound = sound
this.activity = function () {
const today = new Date();
const hour = today.getHours();
if (hour > 8 && hour <= 20) {
return `${this.animal} is playing right now`
} else {
return `${this.animal} is sleeping right now`
}
}
}
set owner(name) {
this.name = name;
console.log(`setter called ${name}`)
}
speak() {
console.log(this.sound);
}
}
const ernie = new Pet('dog', 1, 'pug', 'yip yip ');
console.log(ernie.activity());
Nour El-din El-helw
8,241 PointsNour El-din El-helw
8,241 PointsSorry for not being clear. What I was trying to say is instead of using getters inside a class to create dynamic properties, can we use just use the constructor method to create the dynamic property? If we can't, why not?