Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial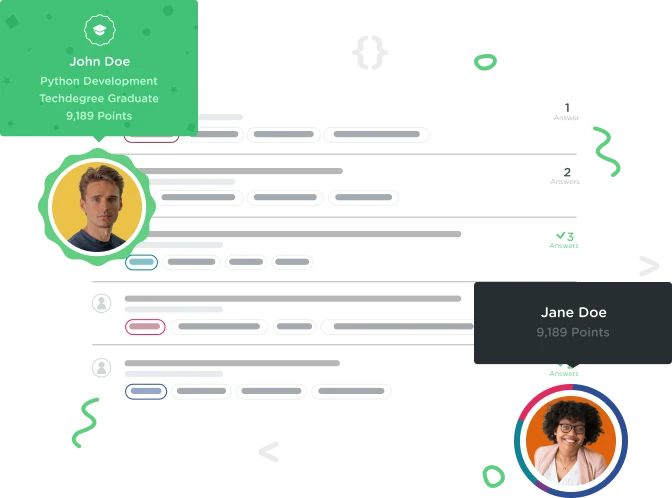
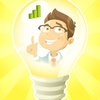
J.R. Sedivy
2,545 PointsDynamic Typing Exercise
I am attempting to follow along with the instructor with the implementation of the dynamic typing exercise but my compiler output doesn't match. The program compiles but varies from the example. The instructor mentioned that he created a Shape class (which creates the Shape.h file and Shape.m files) and I had done this as well but suspect that I should have code in this file which I don't have.
The example compiler output is:
23 tango I am Shape. My parent is NSObject. area -1.000000
My compiler output is:
23 tango <Shape: 0x10010bd70>
Here is the code: main.m:
#import <Cocoa/Cocoa.h>
#import "Shape.h"
int main()
{
Shape *shape = [[Shape alloc] init];
NSArray *mixed = @[@23, @"tango", shape];
for (id thing in mixed) {
NSLog(@"%@", thing);
}
return 0;
}
Shape.h:
#import <Foundation/Foundation.h>
@interface Shape : NSObject
@end
Shape.m:
#import "Shape.h"
@implementation Shape
@end
3 Answers

Taylor Weeks
4,009 PointsTry entering this as your Shape.h file. All the header file does is declare the properties and methods that the class will use, and based on what is in his implementation file, these are the properties and methods that need to be declared.
#import <Foundation/Foundation.h>
@interface Shape : NSObject
@property(nonatomic,strong) NSString* color;
-(double)area;
-(BOOL)hitTestAtLocation:(CGPoint)location;
-(NSString *)description;
@end

Taylor Weeks
4,009 PointsThe reason your console output is different from the instructor's is because his output was the result of
NSLog(@"%@", [thing description]);
where you have
NSLog(@"%@", thing);
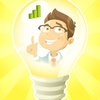
J.R. Sedivy
2,545 PointsI updated as you had suggested but unfortunately this didn't correct the issue. Here's the updated "main.m" file (the other files remained unchanged:
#import <Cocoa/Cocoa.h>
#import "Shape.h"
int main()
{
Shape *shape = [[Shape alloc] init];
NSArray *mixed = @[@23, @"tango", shape];
for (id thing in mixed) {
NSLog(@"%@", [thing description]);
}
return 0;
}
Here is the XCode output:
2014-03-24 16:17:05.108 MyFirstObjectiveCProgram[416:303] 23 2014-03-24 16:17:05.111 MyFirstObjectiveCProgram[416:303] tango 2014-03-24 16:17:05.112 MyFirstObjectiveCProgram[416:303] <Shape: 0x10010bd80>

Taylor Weeks
4,009 PointsOkay, I misunderstood the question. Go to 4:44 in the video and you will see that you are missing code in your shape.m file. Here, he has defined an instance method "description" that returns their values in a string.
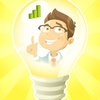
J.R. Sedivy
2,545 PointsNo problem, I appreciate you taking the time to talk through this. After continuing on with the Dynamic Typing video I also noticed the updated "Shape.m" file. I had copied it and updated the original Shape.m to the following:
#import "Shape.h"
@implementation Shape
@synthesize color = _color;
-(double)area {
return -1;
}
-(BOOL)hitTestAtLocation:(CGPoint)location {
return NO;
}
-(NSString *)description {
return [NSString stringWithFormat:@"I am %@. My parent is %@. area %f.",
[self class], [self superclass], [self area]];
}
@end
I ran this with the suggested edits from "main.m" in our previous discussion but I now get a compiler error for Shape.m specific to the "@synthesize line of code: "Property implementation must have its declaration in interface "Shape"." I suppose there may have been an update to "Shape.h" which wasn't shown in the video.
J.R. Sedivy
2,545 PointsJ.R. Sedivy
2,545 PointsThat did the trick. The compiler output is now:
Thanks for your help!