Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial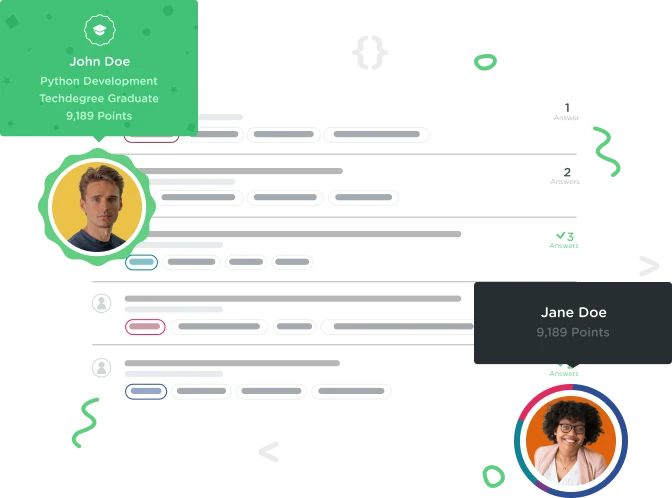

James Croxford
12,722 PointsDynamically changing category labels- my solution isn't working? Can anyone explain why?
So I ended up building a very similar solution to the class (with the exception of using a template literal and not making separate variables within the for loop). I wanted to also make the labels within each template in the for loop dynamic, so if the 'Student', 'Points' etc labels changed in the future within my array of objects, they would automatically update.
I saw online that Object.Keys() was a method to log the keys from an object. After declaring and assigning these values to an array (and logging to the console to check the correct values), and putting this into my template literal, now each value/string is coming up as undefined, even though the labels are correct? What's going on here?
function print (studentInformation) {
let outputDiv = document.getElementById('output');
outputDiv.innerHTML = studentInformation;
}
let studentInformationReadOut = ``;
let categoryArray = Object.keys(students[1]);
console.log(categoryArray);
for (let i = 0; i < students.length; i += 1) {
studentInformationReadOut += `<h3>${categoryArray[0]}: ${students[i]['name']} </h3>
<p>${categoryArray[1]}: ${students[i]['track']}</p>
<p>${categoryArray[2]}: ${students[i]['achievements']}</p>
<p>${categoryArray[3]}: ${students[i]['points']}</p>`;
}
print(studentInformationReadOut);
//data
let students = [
{Name : 'Simon',
Track : 'Java',
Achievements : 16,
Points : 4434
},
{Name : 'Melissa',
Track : 'HTML & CSS',
Achievements : 7,
Points : 1284
},
{Name : 'Amy',
Track : 'JavaScript',
Achievements : 72,
Points : 10983
},
{Name : 'Adam',
Track : 'iOS',
Achievements : 48,
Points : 8712
},
{Name : 'Charlie',
Track : 'Python',
Achievements : 34,
Points : 6790
}
];
//for each person of the five, the output is:
//Name: undefined
//Track: undefined
//Achievements: undefined
//Points: undefined
//no errors are raised in the JavaScript console
1 Answer

James Croxford
12,722 PointsI just noticed my mistake: When I added the Object.keys() method, I capitalised each key within the data (students.js) to correct the formatting when printed to the screen. Then within student-records.js I didn't update the property strings (which access the individual values) within the square brackets on each line.
function print (studentInformation) {
let outputDiv = document.getElementById('output');
outputDiv.innerHTML = studentInformation;
}
let studentInformationReadOut = ``;
let categoryArray = Object.keys(students[1]);
console.log(categoryArray);
for (let i = 0; i < students.length; i += 1) {
studentInformationReadOut += `<h3>${categoryArray[0]}: ${students[i]['Name']} </h3>
<p>${categoryArray[1]}: ${students[i]['Track']}</p>
<p>${categoryArray[2]}: ${students[i]['Achievements']}</p>
<p>${categoryArray[3]}: ${students[i]['Points']}</p>`;
}
print(studentInformationReadOut);
'''