Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial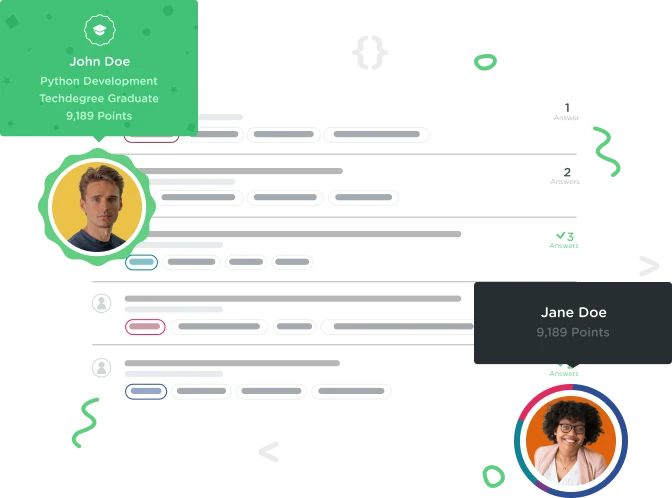
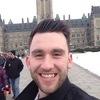
Joseph Greco
13,405 Points$.each() Uncaught ReferenceError: i is not defined
I'm getting a reference error. I'm retrieving json data via ajax. I'm able to post all the data to my console so I know the json object is properly formatted. I'm attempting to loop through the errors array inside the object and manipulate the data accordingly for form validation.
However, the loop itself isn't working and I'm not sure what I'm doing wrong.
$(".contact-form").submit(function(e) {
e.preventDefault();
var url = $(this).attr("action");
var data = $(this).serialize();
$.getJSON(url, data, function(response){
console.log(response);
console.log(response.errors[0].field);
console.log(response.errors[1].field);
console.log(response.errors[0].message);
console.log(response.errors[1].message);
var msg = "<h2>" + response.status[0].message + "</h2>";
$(".outputMsg").replaceWith(msg);
//WHERE ERROR IS OCCURING
$.each(response.errors[i],function(i,val){
var elm = $("#"+response.errors[i].field);
elm.css('border-color','red');
var elm2 = elm.parent().find('.help-block');
elm2.html(response.errors[i].message);
elm2.show();
});
});
return false;
});
2 Answers
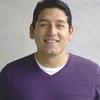
Jose Soto
23,407 PointsjQuery's each method accepts an object and a callback. The callback is scoping the i
variable, so it will not be available outside of that function. Maybe you could try this:
$.each(response.errors, function(i, val){
var elm = $("#"+val[i].field);
elm.css('border-color','red');
var elm2 = elm.parent().find('.help-block');
elm2.html(val[i].message);
elm2.show();
});
You can also check out the documentation here.
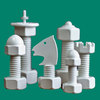
Steven Parker
231,261 PointsYou haven't defined "i" yet, but you are using it as an index.
The variable "i" is only available inside the anonymous function. But since the $.each function is expecting an array, perhaps you didn't mean to subscript response.errors there at all?
Perhaps you meant to do this:
$.each(response.errors, function(i, val) {