Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial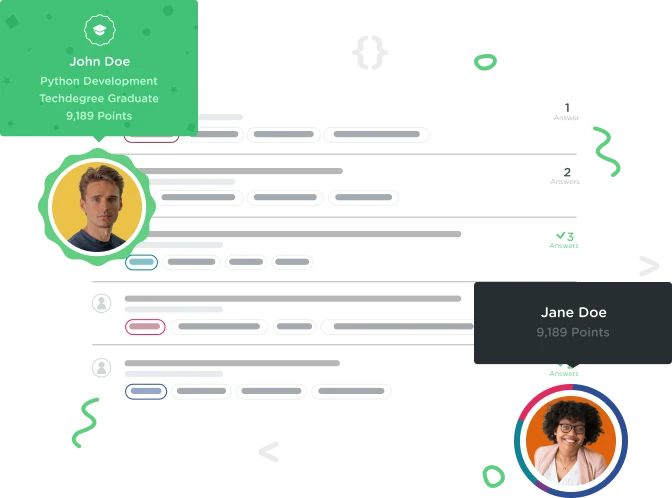
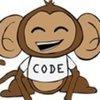
Žiga Pregelj
18,126 PointsEdit button doesn't work on added tasks, but it works on default tasks.
//Problem: user interaction doesn't provide desired results.
//Solution: add interactivity so the user can manage daily tasks.
var taskInput = document.getElementById("new-task"); //new-task
var addButton = document.getElementsByTagName("button")[0];//first-button
var incompleteTasksHolder = document.getElementById("incomplete-tasks");//incomplete-tasks
var completedTasksHolder = document.getElementById("completed-tasks");//completed-tasks
//New task List item
var createNewTaskElement = function (taskString) {
//Create listItem
var listItem = document.createElement("li");
//input checkbox (checkbox)
var checkBox = document.createElement("input");
//label
var label = document.createElement("label");
//input (text)
var editInput = document.createElement("editInput");
//button.edit
var editButton = document.createElement("button");
//button.delete
var deleteButton = document.createElement("button");
//Each element needs modifying
checkBox.type = "checkbox";
editInput.type = "text";
editButton.innerText = "Edit";
editButton.className = "edit";
deleteButton.innerText = "Delete";
deleteButton.className = "delete";
label.textContent = taskString;
//Each element needs appending
listItem.appendChild(checkBox);
listItem.appendChild(label);
listItem.appendChild(editInput);
listItem.appendChild(editButton);
listItem.appendChild(deleteButton);
return listItem;
};
//Add a new task
var addTask = function () {
console.log("Add task...");
//create a new list item with the text from #new-task:
var listItem = createNewTaskElement(taskInput.value);
//Append listItem to IncompleteTaskHolder
incompleteTasksHolder.appendChild(listItem);
bindTaskEvents(listItem, taskCompleted);
};
//edit an existing task
var editTask = function () {
console.log("Edit task...");
var listItem = this.parentNode;
var editInput = listItem.querySelector("input[type=text]");
var label = listItem.querySelector("label");
var containsClass = listItem.classList.contains("editMode");
//if the class of the parent is .editMode
if (containsClass) {
//switch from .editMode
//label text become the input value
label.innerText = editInput.value;
} else {
//switch to edit .editMode
//input value becomes the label's text
editInput.value = label.innerText;
}
//toggle .editMode on the list item
listItem.classList.toggle("editMode");
};
//delete an existing task
var deleteTask = function () {
console.log("delete task...");
var listItem = this.parentNode;
var ul = listItem.parentNode;
//remove the parent list item from the ul
ul.removeChild(listItem);
};
//Mark a task as complete
var taskCompleted = function () {
console.log("Task complete");
//append the task list item to the #completed-task
var listItem = this.parentNode;
completedTasksHolder.appendChild(listItem);
bindTaskEvents(listItem, taskIncomplete);
};
//Mark a task as incomplete
var taskIncomplete = function () {
console.log("task Incomplete");
//append the task list item ti the #incomplete-task
var listItem = this.parentNode;
incompleteTasksHolder.appendChild(listItem);
bindTaskEvents(listItem, taskCompleted);
};
var bindTaskEvents = function (taskListItem, checkBoxEventHandler) {
console.log("Bind list item events");
//select taskListItem's children
var checkBox = taskListItem.querySelector("input[type=checkbox]");
var editButton = taskListItem.querySelector("button.edit");
var deleteButton = taskListItem.querySelector("button.delete");
//bind the editTask to edit button
editButton.onclick = editTask;
//bind the deleteTask to the deleteButton
deleteButton.onclick = deleteTask;
//bind checkBoxEventHandler to the checkbox
checkBox.onchange = checkBoxEventHandler;
};
//Set the click hanler to the addTask function
addButton.onclick = addTask; //without brackets, so its called on click, not as function
//cycle over the incompleteTaskHolder ul list items
for (var i = 0; i < incompleteTasksHolder.children.length; i++){
//bind events to list item's children (taskCompleted)
bindTaskEvents(incompleteTasksHolder.children[i], taskCompleted);
}
//cycle over the completeTaskHolder ul list items
for (var i = 0; i < completedTasksHolder.children.length; i++){
//bind events to list item's children (taskIncomplete)
bindTaskEvents(completedTasksHolder.children[i], taskIncomplete);
What is wrong? I checked many questions and answers on this topic, but no one is working for me... Please help :)