Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial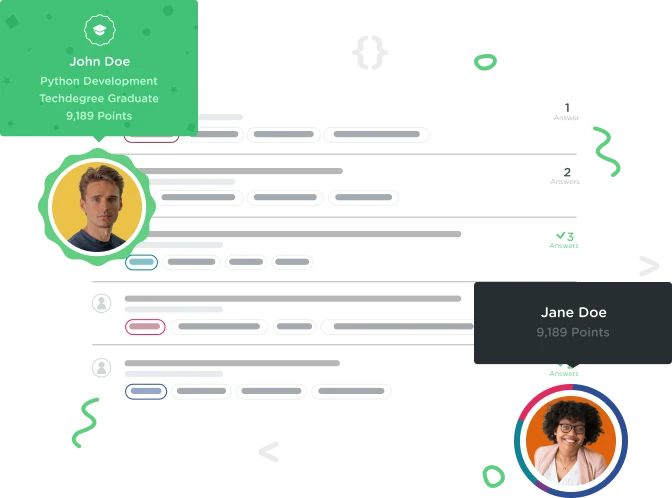

Kenneth Phillips
Courses Plus Student 10,188 PointsEditText FileNotFoundException
I'm writing an app where if someone answers a question correctly in the EditText it will move on to the next one. This process I put in my if condition in the SpecialCondition method. However it seems that android studio can't read the text. Here is my code:
private Game game; private ImageView gameImageView; private TextView gameTextView; private Button submitButton; private TextView pointsTextView; private int points;
@Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_game);
gameImageView = (ImageView) findViewById(R.id.gameImageView);
gameTextView = (TextView) findViewById(R.id.gameTextView);
pointsTextView = (TextView) findViewById(R.id.pointsTextView);
submitButton = (Button) findViewById(R.id.submitButton);
game = new Game();
loadPage(0);
specialCondition(0);
}
private void loadPage(final int pageNumber) {
submitButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
specialCondition(pageNumber);
}
});
}
private void specialCondition(int pageNumber) { final Page page = game.getPage(pageNumber); if (pageNumber == 20) { startSubmit(); } else if (pageNumber < 20) { Drawable image = ContextCompat.getDrawable(this, page.getImageId()); gameImageView.setImageDrawable(image); String gameAnswer = getString(game.getPage(pageNumber).getAnswerId());
String pageText = getString(page.getTextId());
gameTextView.setText(pageText);
String pointsText = String.format("%1$s", points);
pointsTextView.setText(pointsText);
String gameEditText = ((EditText) findViewById(R.id.gameSquare)).getText().toString();
if (gameEditText == gameAnswer.toLowerCase()) {
points++;
} else if (gameEditText != gameAnswer.toLowerCase()) {
points--;
}
int nextPage = page.getNextPage();
loadPage(nextPage);
}
}
private void startSubmit() { Intent submitIntent = new Intent(this, SubmitActivity.class); startActivity(submitIntent); } }
My error is FileNotException. I think this means there isn't a clear path for the machine to see what I'm referencing. How would I fix this so that it can see the EditText? Also sorry my code is coming out in separate chunks.
2 Answers

Seth Kroger
56,413 PointsI don't think this is what's causing the error but, if you are comparing Strings you should use .equals() or .equalsIgnoreCase(), not == or != (and strictly speaking, you don't need the second comparison either, if it isn't equal it has to be not equal)
A quick Google search of "E/Hyphenator: error loading hyphenation" suggests it could be corrupted SDK or emulator but nothing definitive.

Kenneth Phillips
Courses Plus Student 10,188 PointsYes that was mostly the problem. Thanks for the help Seth.

Kenneth Phillips
Courses Plus Student 10,188 PointsThis is my error message that repeated 20 times for every answer I put in:
10-11 19:38:56.503 1366-1366/? E/Hyphenator: error loading hyphenation /system/usr/hyphen-data/hyph-en-us.pat.txt java.io.FileNotFoundException: /system/usr/hyphen-data/hyph-en-us.pat.txt: open failed: ENOENT (No such file or directory) at libcore.io.IoBridge.open(IoBridge.java:452) at libcore.io.IoUtils$FileReader.<init>(IoUtils.java:207) at libcore.io.IoUtils.readFileAsString(IoUtils.java:114) at android.text.Hyphenator.loadHyphenator(Hyphenator.java:96) at android.text.Hyphenator.init(Hyphenator.java:154) at com.android.internal.os.ZygoteInit.preloadTextResources(ZygoteInit.java:207) at com.android.internal.os.ZygoteInit.preload(ZygoteInit.java:186) at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:593) Caused by: android.system.ErrnoException: open failed: ENOENT (No such file or directory) at libcore.io.Posix.open(Native Method) at libcore.io.BlockGuardOs.open(BlockGuardOs.java:186) at libcore.io.IoBridge.open(IoBridge.java:438)
It won't tell me what line of code causes the exception but when I add in the if and else method for points and the gameEditText that is when the exception is thrown.
Seth Kroger
56,413 PointsSeth Kroger
56,413 PointsWhich exact line of your code causes or precipitates the exception? (The answer is in the exception message)