Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial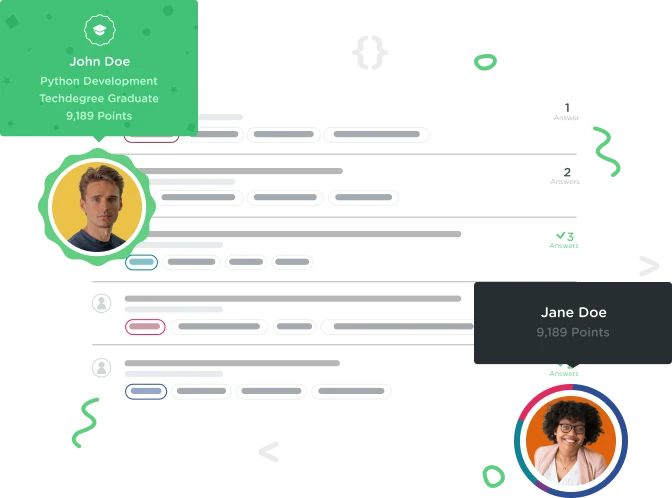

ameerm
Full Stack JavaScript Techdegree Graduate 14,976 PointsElse if conditional statements
I am stuck on this challenge task using else clause.
var isAdmin = false;
var isStudent = false;
if ( isAdmin ) {
alert('Welcome administrator');
} else if (isStudent) {
alert('Welcome student');
}
else if (isAdmin === false isStudent === false) {
alert("Who are you"?);
}
<!DOCTYPE HTML>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>JavaScript Basics</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
2 Answers
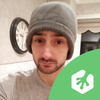
Jamie Reardon
Treehouse Project ReviewerYou don't need another else if clause here as the challenge tells you to add a final else clause. It should also be a hint to you that the program can't check for any other conditions and now needs a final fall back piece of code to run since if both the initial if statement and the else if statement following fails.
Your new else if statement you have added is not needed here, as the program has already checked for the first value in isAdmin to be false, if it is, then it will go the next condition in the else if statement which will then check the isStudent value, if it is to be false as well, then both checks evaluate to false (which is the same as your conditional logical of "isAdmin === false isStudent === false"). All you need is to add an else statement after the first else if statement and add the code inside the block that the challenge asks of you.
var isAdmin = false;
var isStudent = false;
if ( isAdmin ) {
alert('Welcome administrator');
} else if (isStudent) {
alert('Welcome student');
} else {
// add code here...
}

ameerm
Full Stack JavaScript Techdegree Graduate 14,976 PointsGreat explanation, thank you.
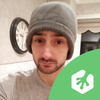
Jamie Reardon
Treehouse Project ReviewerGlad it helped!
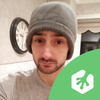
Jamie Reardon
Treehouse Project ReviewerJust to note: I forgot to mention about your condition that was checking for two values. If you are trying to create a condition that will evaluate two or more values like this, you needed to add the logical operator and (&&) which checks that both values need to be true in order for the whole condition to be true.
Example:
(isAdmin === false && isStudent === false)
If you wanted to check that either of the values need to be true and not strictly both, you could use the OR operator (||)
Example:
(isAdmin === false || isStudent === false)

ameerm
Full Stack JavaScript Techdegree Graduate 14,976 PointsJamie Reardon, thank you, I was going to use a comma for that but good to know about the and, or operators because the comma wouldn’t have worked
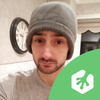
Jamie Reardon
Treehouse Project ReviewerThere is a video under the JavaScript Basics course that talks about the types of operators you can use in your conditional statements. :)