Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial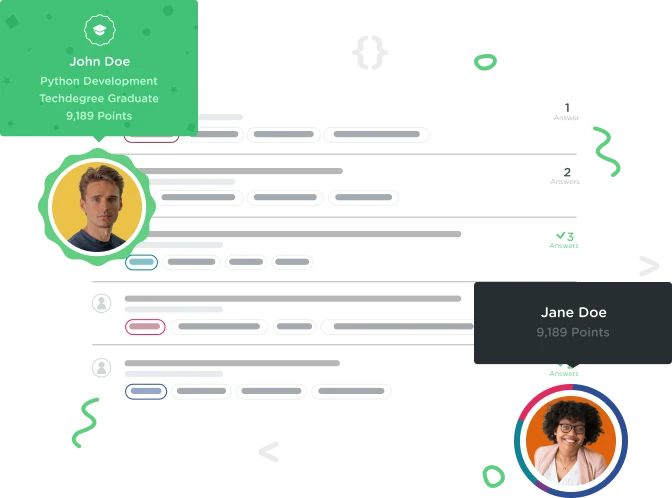

andynguyen9
1,629 Pointselsif
car_speed = 55 speed_limit = 55 going_speed_limit = "true" if car_speed == speed_limit return going_speed_limit == "true" end
What's wrong with these codes?
car_speed = 55
speed_limit = 55
going_speed_limit = "true"
if car_speed == speed_limit
return going_speed_limit == "true"
end
3 Answers
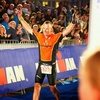
Steve Hunter
57,712 PointsHi Andy,
The challenge here is to modify the code to use an elsif
rather than just an else
.
The elsif
allows a second conditional test to take place if the first test has failed. Here, the first test has failed, in the car_speed
is not less than speed_limit
. So we want to introduce a second test in the elsif
to see if car_speed
equals speed_limit
.
If that condition is met, we want to set a variable called going_speed_limit
to true
.
To do this, insert before the existing else
section of the code. Add the elsif
keyword. After this keyword, on the same line, test, using ==
whether car_speed
is equal to speed_limit
. Inside the block, on the next line, set going_speed_limit
to true
using single-equals.
This all looks like:
car_speed = 55
speed_limit = 55
if car_speed < speed_limit
too_fast = false
elsif car_speed == speed_limit
going_speed_limit = true
else
too_fast = true
end
Steve.

andynguyen9
1,629 PointsMy codes would run like this:
car_speed = 55
speed_limit = 55
going_speed_limit = true
if car_speed < speed_limit
too_fast = false
elsif
going_speed_limit = true
end
=========================== However, I got confused that:
- There is no variable too_fast.
- why using elsif when we can use else
Please explain. Th ank you, Steve.
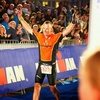
Steve Hunter
57,712 PointsHi Andy,
This challenge starts with code that sets the value of too_fast
to true
or false
depending whether car_speed
is greater or less than the speed_limit
. This challenge wants us to add the third option; the case where car_speed
equals speed_limit
.
For this, we need a new condition as the existing test just lets us know whether car_speed < speed_limit
. To introduce a new condition, we use elsif
. This allows us to make a new test - so here, we can insert a test to see if car_speed == speed_limit
.
This allows a third outcome to come from an if
conditional statement. Yes, in this challenge there are some 'made up' variables, too_fast
and going_speed_limit
are expressly defined but the challenge knows to expect them to be used. Don't worry about it; call it 'artistic licence'.
so, in between the if
and the else
add an elsif
and the new test on the same line. On the next (new) line set the variable going_speed_limit
to true. This means the code can handle the three possible outcomes depending on the value of car_speed
.
if car_speed < speed_limit
too_fast = false
elsif car_speed == speed_limit
going_speed_limit = true
else
too_fast = true
end
I hope that helps.
Steve.