Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial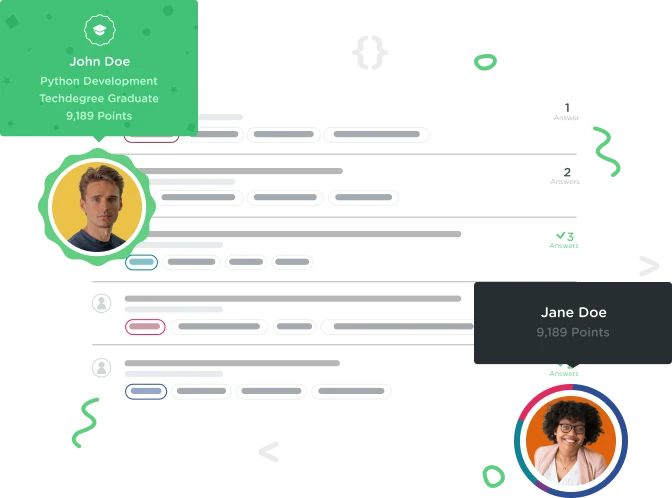
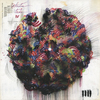
eyeloveotters
15,097 Points$email_body
I don't understand why it makes sense to reassign the variable $email_body three times and have each variable display in the browser when it is only echoed one time.
This is what we were instructed to do:
<?php
$name = $_POST["name"];
$email = $_POST["email"];
$message = $_POST["message"];
$email_body = "";
$email_body = $email_body . "Name: " . $name . "\n";
$email_body = $email_body . "Email: " . $email . "\n";
$email_body = $email_body . "Message: " . $message . "\n";
echo $email_body;
?>
The strange thing to me is that it works.
Shouldn't echoing $email_body only display the message since that was the last assignment? Could someone please explain how this works?
Much thanks.
3 Answers
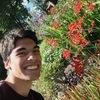
Matthew Mascioni
20,444 PointsHi Adam,
In this particular case, we're re-assigning the variable as itself, and then concatenating something to it. First, we initialize it with no value, and with every line after that, we're adding something new to the value for the variable.
For example, if I declared a variable called favourite_foods, and set it's value to 'pizza':
$favourite_foods = 'pizza';
We can add something new to the value of this variable by concatenating itself to the new value to be added. Let's say I wanted to add 'ice cream' to the value of $favourite_foods, I could do this:
$favourite_foods = $favourite_foods . " ice cream";
The value of $favourite_foods should now be:
pizza ice cream

Ryan Stratton
19,181 PointsBased on the code example you provided, echoing the variable $email_body should output all three lines. In PHP the right side of the equation is evaluated first. By declaring that "$email_body = $email_body . $name" you are taking the current value of $email_body, concatenating on the value $name and assigning the result back to $email_body.
So you can visualize what's happening I've updated your code example below with comments showing the value of $email_body after each expression.
$name = "My Name";
$email = "My Email";
$email = "My Message";
$email_body = "";
// $email_body equals an empty string.
$email_body = $email_body . "Name: " . $name . "\n";
// $email_body's empty string is concatenated to "Name: My Name\n"
// $email_body equals "Name: My Name\n"
$email_body = $email_body . "Email: " . $email . "\n";
// $email_body equals "Name: My Name \n Email: My Email\n"
// '\n' being a newline
There's more than one way to handle concatenation in PHP. Another common way to handle concatenation is to use the '.=' assignment operator, which some people find much easier to read. Here's an example of how that might work.
$email_body = "";
$email_body .= "Email: ";
$email_body .= "My Email";
echo $email_body;
// 'Email: My Email'
Hope this help!
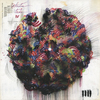
eyeloveotters
15,097 PointsAwesome answer. Thank you!
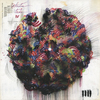
eyeloveotters
15,097 PointsHi Matthew,
Thank you for answering! I think I see why that happens now. So each new line that reassigns the variable is concatinating the previous variable assignment with the new assignment?
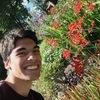
Matthew Mascioni
20,444 PointsExactly! Happy hacking :)