Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial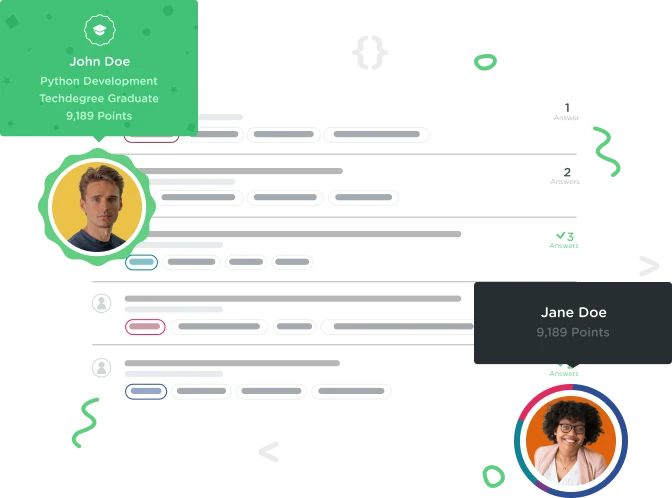

Ben S
5,638 PointsEmpty card array declaration
Is there any specific reason why card is first declared as an empty array and then the values are pushed to it, as opposed to declaring it with the values included?
for (let i = 0; i < suites.length; i++) {
for (let j = 0; j < ranks.length; j++) {
const card = [];
card.push(ranks[j], suites[i]);
deck.push(card);
}
}
vs
for (let i = 0; i < suites.length; i++) {
for (let j = 0; j < ranks.length; j++) {
const card = [ranks[j], suites[i]];
deck.push(card);
}
}
1 Answer
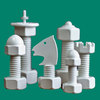
Steven Parker
229,783 PointsI expect the video uses separate steps to clearly illustrate that the values are being put into an array, and then that array is being added to another one. You can make the whole process even more compact by eliminating the intermediate variables entirely:
for (let i = 0; i < suites.length; i++) {
for (let j = 0; j < ranks.length; j++) {
deck.push([ranks[j], suites[i]]);
}
}
And it can be even further compacted by using array methods instead of loops:
var deck = suites.map(s => ranks.map(r => [r, s])).reduce((d,c) => [...d, ...c]);
But as you see, the more compact you make it, the less obvious what it is doing becomes.