Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial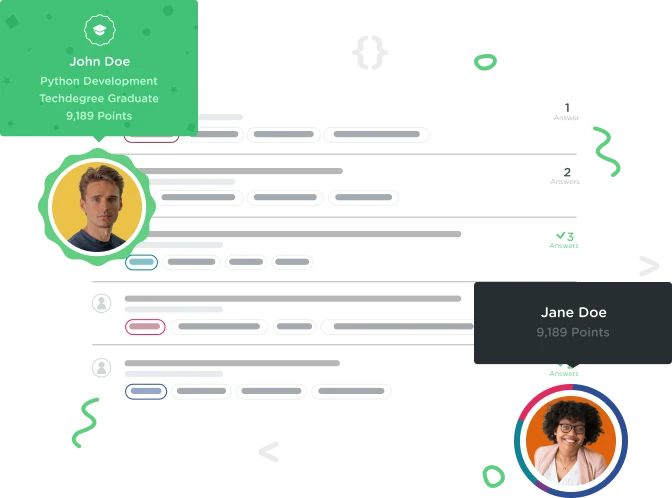
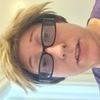
Katriel Paige
9,260 PointsEmpty? method (returning Boolean values)
Okay, so I've gotten things up to this point as the syntax is fairly straightforward, but for some reason I keep getting a "bummer, try again" on this exercise. Am I missing something obvious?
Also wouldn't I get nil if there is only one item in the todo_items array?
class TodoList
attr_reader :name, :todo_items
def initialize(name)
@name = name
@todo_items = []
end
def add_item(name)
todo_items.push(TodoItem.new(name))
end
def empty?(name)
if @todo_items == 0
return true
else @todo_items >= 1
return false
end
end
end
2 Answers
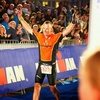
Steve Hunter
57,712 PointsHi Katriel,
I just used a straight comparison with zero. However, I don't think this fits the challenge but the code does pass.
def empty?
@todo_items.count == 0
end
The question isn't covering the scenario where there is one item in the array, as you say! However, the code is expecting only two outcomes; true or false, based on empty & not empty. The challenge code doesn't distinguish three states, empty; has one; has more than one.
I hope that helps,
Steve.
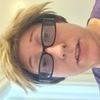
Katriel Paige
9,260 PointsOh, okay! Sometimes the questions are not always so straightforward in interpretation (because naturally I'd think you'd have to cover three cases - empty, more than one, one - based on the wording of the question) but the clarification helps in terms of the exercise. Thanks!
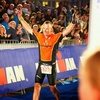
Steve Hunter
57,712 PointsI agree this question isn't accurately defining what code is required to pass the challenge. But, it is the correct interpretation of a helper method like empty?
. That should return only two possible values; true or false. Having other states is contradictory to the purpose of this type of method.
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsIn short, I agree with your interpretation of the question; your code reflects a solution to the question posed.