Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial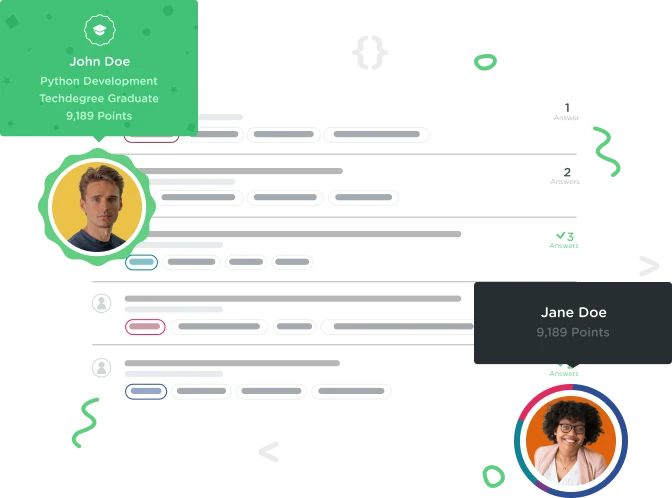
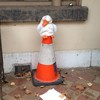
bronnyreinhardt
1,007 PointsEncapsulation
After the structs lesson got to encapsulation I found it very confusing.
#include <stdio.h>
typedef struct {
float center[3];
float radius;
} Sphere;
Sphere makeSphere(float *c, float r);
int main()
{
float c[] = {23, 45, 67};
float r = 32;
Sphere ball = makeSphere(c, r);
printf("center %f %f %f radius %f\n", ball.center[0], ball.center[1], ball.center[2], ball.radius);
return 0;
}
Sphere makeSphere(float *c, float r) {
Sphere sphere;
sphere.center[0] = c[0];
sphere.center[1] = c[1];
sphere.center[2] = c[2];
sphere.radius = r;
return sphere;
}
Does anybody have another example of encapsulation that would elucidate this process? Also,
– Why does the instructor mention declaring two functions? I can only see one – 'makeSphere'
– In the function call, how come you don't need a star notation or some other kind of array identifier?:
Sphere ball = makeSphere (c, r);
Also, in the first part of the lesson (structs) the instructor uses the values 24, 32, 45 and 67. These change in the second part of the lesson (structs + functions) – is this intentional? Is changing them meant to demonstrate something?
At the end the instructor mentions making multiple spheres using the makeSphere function – how would you do this? I can't see how combining a struct with a function has saved time here or has the potential to save time.
Andrew Shook? Sorry to name you; you were so helpful last time :)
2 Answers
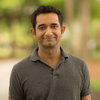
Amit Bijlani
Treehouse Guest TeacherThere are a lot of questions here and rightly so because these are all new concepts. You will gain better understanding as you move forward with Objective-C.
Q. And maybe give another small example of encapsulation?
Encapsulation in plain english means hiding direct access to data and only providing access via functions or methods.
Q. Why does the instructor mention declaring two functions? I can only see one – 'makeSphere'
I think that was an error. Sorry about that.
Q. What is actually happening during the implementation and calling of the function? The instructor keeps mentioning 'passing' but this process is opaque to me.
In the main
function when you call the makeSphere
you are giving it two variables: Sphere ball = makeSphere(c, r);
You are passing values to that function which can be accessed within the function using the parameter names in its implementation. That's what he means by 'passing'.
Q. In the function call, how come you don't need a star notation?
It all depends what is being returned by the function. In this case the function returns a struct and the struct is simply a container of values. You don't have pointer to a struct.
Q. Also, in the first part of the lesson (structs) the instructor uses the values 24, 32, 45 and 67. These change in the second part of the lesson (structs + functions) – is this intentional? Is changing them meant to demonstrate something?
No that was just part of the editing where we might have had to correct some mistake while recording.
Q. At the end the instructor mentions making multiple spheres using the makeSphere function – how would you do this? I can't see how combining a struct with a function has saved time here or has the potential to save time.
You would just call the function to make sphere each time.
int main()
{
float c[] = {23, 45, 67};
float r = 32;
Sphere ball = makeSphere(c, r);
Sphere ball2 = makeSphere(c,45); // you don't have to store values in a variable to pass it to a function
float c2[] = {33, 25, 17};
float r2 = 77;
Sphere ball3 = makeSphere(c2,r2);
printf("center %f %f %f radius %f\n", ball.center[0], ball.center[1], ball.center[2], ball.radius);
return 0;
}
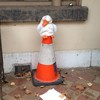
bronnyreinhardt
1,007 PointsThanks Amit Bijlani – just to clarify:
Sphere ball2 = makeSphere(c,45); // you don't have to store values in a variable to pass it to a function
Is the above not relevant for arrays?
{
float c[] = {23, 45, 67};
float r = 32;
Sphere ball = makeSphere(c, r);
float c2[] = {42, 26, 68};
float r2 = 55;
Sphere marble = makeSphere(c, r);
printf("ball center %f %f %f radius %f marble center %f %f %f radius %f\n", ball.center[0], ball.center[1], ball.center[2], ball.radius, marble.center[0], marble.center[1], marble.center[2], marble.radius);
return 0;
}
I tried this myself but had two issues (c2 and r2 were 'unused variables').
Is something in the function or the implementation supposed to be changed? (And if so, this relates to my original confusion about how to use a function to make multiple Spheres and its time-saving potential)
Cheers.
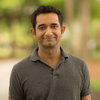
Amit Bijlani
Treehouse Guest TeacherYes it is relevant for arrays too.
You declared c2 and r2 but you never used them in the following call to the makeSphere
function you should probably pass the c2 and r2 variables.