Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial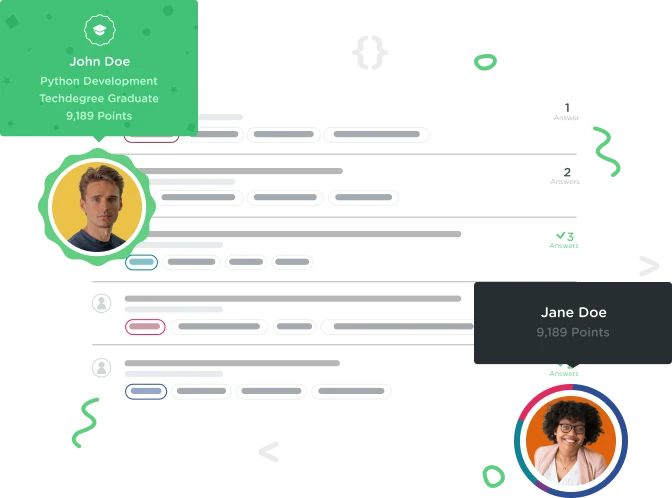
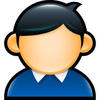
Daniel Hildreth
16,170 PointsEncountering _EntryForm not found or no supported view engine...
So my code looks the same as the video, yet I'm getting a buggy error. When I hit F5 to run my app, I get this error:
An exception of type 'System.InvalidOperationException' occurred in System.Web.Mvc.dll but was not handled in user code
Additional information: The partial view '_EntryForm' was not found or no view engine supports the searched locations. The following locations were searched:
- $exception {"The partial view '_EntryForm' was not found or no view engine supports the searched locations. The following locations were searched:\r\n~/Views/Entries/_EntryForm.aspx\r\n~/Views/Entries/_EntryForm.ascx\r\n~/Views/Shared/_EntryForm.aspx\r\n~/Views/Shared/_EntryForm.ascx\r\n~/Views/Entries/_EntryForm.cshtml\r\n~/Views/Entries/_EntryForm.vbhtml\r\n~/Views/Shared/_EntryForm.cshtml\r\n~/Views/Shared/_EntryForm.vbhtml"} System.InvalidOperationException
Does anyone know why I am getting this error?
3 Answers

James Churchill
Treehouse TeacherDaniel,
Looking at the error message, you can see the locations that MVC is looking within for your partial view.
~/Views/Entries/EntryForm.aspx
~/Views/Entries/_EntryForm.ascx
~/Views/Shared/EntryForm.aspx
~/Views/Shared/EntryForm.ascx
~/Views/Entries/EntryForm.cshtml
~/Views/Entries/_EntryForm.vbhtml
~/Views/Shared/_EntryForm.cshtml
~/Views/Shared/EntryForm.vbhtml
Looking at your GitHub repo, it looks like the "_EntryForm.cshtml" partial view is located in the "Views" folder. I'd try moving that file to either the "Views/Entries" or "Views/Shared" folder.
If a partial view is only shared across views for a single controller, I'd recommend placing the partial view in views folder for that controller. If a partial view is shared across views for more than one controller, I'd place the partial view in the "Shared" folder.
Hope this helps.
Thanks ~James
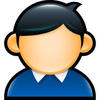
Daniel Hildreth
16,170 PointsThank you James, that fixed my issue. Amazing a simple folder moving can make such a big difference.
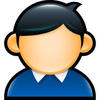
Daniel Hildreth
16,170 PointsSteven it is on GitHub. Didn't think to post that part earlier. Here is the link to the GitHub page.
https://github.com/HanSolo0001/Fitness-Frog
Here is also a link to the latest video on that part of the code where I noticed it giving that error.
https://teamtreehouse.com/library/creating-a-partial-view-for-our-form
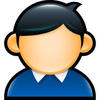
Daniel Hildreth
16,170 PointsHere is the physical code too so you don't have to go to GitHub for it (sorry for it being long).
_EntryForm.cshtml
@model Treehouse.FitnessFrog.Models.Entry
@using Treehouse.FitnessFrog.Models
@using (Html.BeginForm())
{
@Html.ValidationSummary("The following errors were found:", new { @class = "alert alert-danger" })
<div class="row">
<div class="col-md-6">
<div class="form-group">
@Html.LabelFor(m => m.Date, new { @class = "control-label" })
@Html.TextBoxFor(m => m.Date, "{0:d}", new { @class = "form-control datepicker" })
</div>
<div class="form-group">
@Html.LabelFor(m => m.ActivityId, new { @class = "control-label" })
@Html.DropDownListFor(m => m.ActivityId, (SelectList)ViewBag.ActivitiesSelectListItems, "", new { @class = "form-control" })
</div>
<div class="form-group">
@Html.LabelFor(m => m.Duration, new { @class = "control-label" })
@Html.TextBoxFor(m => m.Duration, new { @class = "form-control" })
</div>
<div class="form-group">
@Html.LabelFor(m => m.Intensity, new { @class = "control-label" })
<div>
<label class="radio-inline">
@Html.RadioButtonFor(m => m.Intensity, Entry.IntensityLevel.Low) @Entry.IntensityLevel.Low
</label>
<label class="radio-inline">
@Html.RadioButtonFor(m => m.Intensity, Entry.IntensityLevel.Medium) @Entry.IntensityLevel.Medium
</label>
<label class="radio-inline">
@Html.RadioButtonFor(m => m.Intensity, Entry.IntensityLevel.High) @Entry.IntensityLevel.High
</label>
</div>
</div>
<div class="form-group">
<div class="checkbox">
<label>
@Html.CheckBoxFor(m => m.Exclude) @Html.DisplayNameFor(m => m.Exclude)
</label>
</div>
</div>
</div>
<div class="col-md-6">
<div class="form-group">
@Html.LabelFor(m => m.Notes, new { @class = "control-label" })
@Html.TextAreaFor(m => m.Notes, 14, 20, new { @class = "form-control" })
</div>
</div>
<div class="col-md-12">
<div class="pad-top">
<button type="submit" class="btn btn-success btn-lg margin-right">
<span class="glyphicon glyphicon-save"></span> Save
</button>
<a href="@Url.Action("Index")" class="btn btn-warning btn-lg">
<span class="glyphicon glyphicon-remove"></span> Cancel
</a>
</div>
</div>
</div>
}
Edit.cshtml
@model Treehouse.FitnessFrog.Models.Entry
@{
ViewBag.Title = "Edit Entry";
}
<h2>@ViewBag.Title</h2>
@Html.Partial("_EntryForm")
Add.cshtml
@model Treehouse.FitnessFrog.Models.Entry
@{
ViewBag.Title = "Add Entry";
}
<h2>@ViewBag.Title</h2>
@Html.Partial("_EntryForm")
EntriesController.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Net;
using System.Web;
using System.Web.Mvc;
using Treehouse.FitnessFrog.Data;
using Treehouse.FitnessFrog.Models;
namespace Treehouse.FitnessFrog.Controllers
{
public class EntriesController : Controller
{
private EntriesRepository _entriesRepository = null;
public EntriesController()
{
_entriesRepository = new EntriesRepository();
}
public ActionResult Index()
{
List<Entry> entries = _entriesRepository.GetEntries();
// Calculate the total activity.
double totalActivity = entries
.Where(e => e.Exclude == false)
.Sum(e => e.Duration);
// Determine the number of days that have entries.
int numberOfActiveDays = entries
.Select(e => e.Date)
.Distinct()
.Count();
ViewBag.TotalActivity = totalActivity;
ViewBag.AverageDailyActivity = (totalActivity / (double)numberOfActiveDays);
return View(entries);
}
public ActionResult Add()
{
var entry = new Entry()
{
Date = DateTime.Today,
};
SetupActivitiesSelectListItems();
return View(entry);
}
[HttpPost]
public ActionResult Add(Entry entry)
{
ValidateEntry(entry);
if (ModelState.IsValid)
{
_entriesRepository.AddEntry(entry);
return RedirectToAction("Index");
}
SetupActivitiesSelectListItems();
return View(entry);
}
public ActionResult Edit(int? id)
{
if (id == null)
{
return new HttpStatusCodeResult(HttpStatusCode.BadRequest);
}
Entry entry = _entriesRepository.GetEntry((int)id);
if (entry == null)
{
return HttpNotFound();
}
SetupActivitiesSelectListItems();
return View(entry);
}
[HttpPost]
public ActionResult Edit(Entry entry)
{
ValidateEntry(entry);
if (ModelState.IsValid)
{
_entriesRepository.UpdateEntry(entry);
return RedirectToAction("Index");
}
SetupActivitiesSelectListItems();
return View(entry);
}
public ActionResult Delete(int? id)
{
if (id == null)
{
return new HttpStatusCodeResult(HttpStatusCode.BadRequest);
}
return View();
}
private void ValidateEntry(Entry entry)
{
// If there aren't any "Duration" field validation errors
// then make sure that the duration is greater than "0".
if (ModelState.IsValidField("Duration") && entry.Duration <= 0)
{
ModelState.AddModelError("Duration", "The Duration field value must be greater than '0'.");
}
}
private void SetupActivitiesSelectListItems()
{
ViewBag.ActivitiesSelectListItems = new SelectList(Data.Data.Activities, "Id", "Name");
}
}
}
_Layout.cshtml
@{
var applicationName = "Fitness Frog";
}
@helper GetAddEntryButtonVisibility()
{
if ((string)ViewContext.RouteData.Values["controller"] == "Entries" &&
(string)ViewContext.RouteData.Values["action"] == "Add")
{
<text>hidden</text>
}
else
{
<text>visible</text>
}
}
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>@ViewBag.Title - @applicationName</title>
<link href="~/favicon.ico" rel="shortcut icon" type="image/x-icon">
<link href="~/favicon.ico" rel="icon" type="image/x-icon">
<link href="https://fonts.googleapis.com/css?family=Open+Sans|Secular+One" rel="stylesheet">
@*<link href="~/Content/bootstrap.min.css" rel="stylesheet" type="text/css" />
<link href="~/Content/bootstrap-datepicker3.min.css" rel="stylesheet" type="text/css" />*@
<link href="~/Content/site.css" rel="stylesheet" type="text/css" />
<script src="~/Scripts/modernizr-2.6.2.js"></script>
</head>
<body>
<div class="navbar navbar-inverse navbar-fixed-top">
<div class="container">
<div class="navbar-header">
<button type="button" class="navbar-toggle" data-toggle="collapse" data-target=".navbar-collapse">
<span class="icon-bar"></span>
<span class="icon-bar"></span>
<span class="icon-bar"></span>
</button>
<a class="navbar-brand" href="@Url.Action("Index", "Entries")">
@Html.Partial("_Logo", new ViewDataDictionary { { "element-id", "logo" } })
@applicationName
</a>
</div>
<div class="navbar-collapse collapse">
<ul class="nav navbar-nav">
</ul>
<a href="@Url.Action("Add", "Entries")" style="visibility: @GetAddEntryButtonVisibility()"
class="btn btn-primary navbar-btn navbar-right">
<span class="glyphicon glyphicon-plus"></span> Add Entry
</a>
</div>
</div>
</div>
<div class="container body-content">
@RenderBody()
<footer>
@Html.Partial("_Logo", new ViewDataDictionary { { "element-id", "footer_logo" } })
<p>© @DateTime.Now.Year @applicationName, All Rights Reserved.</p>
</footer>
</div>
<script src="~/Scripts/jquery-1.10.2.min.js"></script>
<script src="~/Scripts/jquery.validate.min.js"></script>
<script src="~/Scripts/jquery.validate.unobtrusive.min.js"></script>
<script src="~/Scripts/jquery.validate.bootstrap.js"></script>
<script src="~/Scripts/bootstrap.min.js"></script>
<script src="~/Scripts/bootstrap-datepicker.min.js"></script>
<script>
$('input.datepicker').datepicker({
format: 'm/d/yyyy'
})
</script>
</body>
</html>
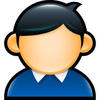
Daniel Hildreth
16,170 PointsI am still getting this error. Any advice?
An exception of type 'System.InvalidOperationException' occurred in System.Web.Mvc.dll but was not handled in user code
Additional information: The partial view '_EntryForm' was not found or no view engine supports the searched locations. The following locations were searched:
$exception {"The partial view 'EntryForm' was not found or no view engine supports the searched locations. The following locations were searched:
\r\n~/Views/Entries/EntryForm.aspx
\r\n~/Views/Entries/_EntryForm.ascx
\r\n~/Views/Shared/EntryForm.aspx
\r\n~/Views/Shared/EntryForm.ascx
\r\n~/Views/Entries/EntryForm.cshtml
\r\n~/Views/Entries/_EntryForm.vbhtml
\r\n~/Views/Shared/_EntryForm.cshtml
\r\n~/Views/Shared/EntryForm.vbhtml"}
System.InvalidOperationException
Steven Parker
231,275 PointsSteven Parker
231,275 PointsTry sharing your code (or your github repo) here.
You might be overlooking a typo or some other issue that is causing the problem, and perhaps someone else can help you spot it when they see your code.